Write a simple trivia quiz game using c++ Start by creating a Trivia class that contains information about a single trivia question. The class should contain a string for the question, a string for the answer to the question, and an integer representing the dollar amount the question is worth (harder questions should be worth more). Add appropriate constructor and accessor functions. In your main function create either an array or a vector of type Trivia and hard-code at least five trivia questions of your choice. Your program should then ask each question to the player, input the player’s answer, and check if the player’s answer matches the actual answer. If so, award the player the dollar amount for that question. If the player enters the wrong answer your program should display the correct answer. When all questions have been asked display the total amount that the player has won.
Write a simple trivia quiz game using c++
Start by creating a Trivia class that contains
information about a single trivia question. The class should contain a string
for the question, a string for the answer to the question, and an integer representing the dollar amount the question is worth (harder questions should
be worth more). Add appropriate constructor and accessor functions. In your
main function create either an array or a
at least five trivia questions of your choice. Your program should then ask
each question to the player, input the player’s answer, and check if the player’s
answer matches the actual answer. If so, award the player the dollar amount
for that question. If the player enters the wrong answer your program should
display the correct answer. When all questions have been asked display the total
amount that the player has won.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

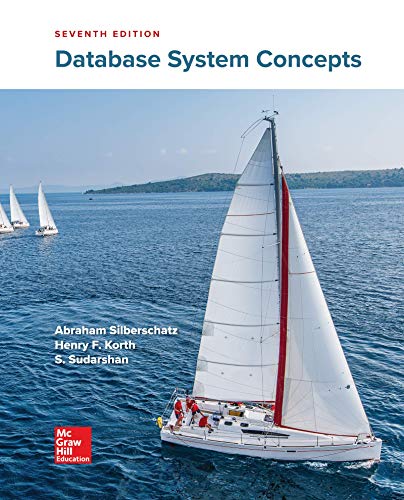
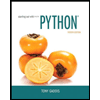
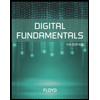
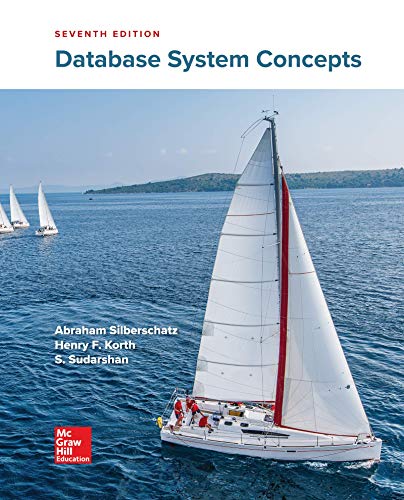
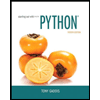
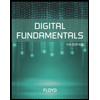
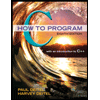
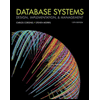
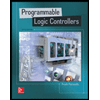