1) Change it to an implementation in a circular fashion. (2) Change it to handle the exceptions. (3) Change the main() function, such that it a. receives integers from the standard I/O and perform enqueue() operations until the queue is full. b. performs one more enqueue() operation to show your program able to handle fullQueue exception. c. prints out the size and all the elements in the queue to show the queue is full with totally 10 integers in it. d. performs dequeue() operations until the queue is empty. e. performs one more dequeue() operation to show your program is able to handle emptyQueue exception. f. Implement a function deleteElement(Q, x) that deletes an element (integer) x from queue Q if x is in Q. g. Call function deleteElement(Q, x) and show that it works correctly. please check
The following C program is an implementation of Queue using an array.
#include <stdio.h>
#include <stdlib.h>
#define size 11
void enqueue(int *q,int *tail, int element);
int dequeue(int *q,int *head);
int full(int tail,const int size);
int empty(int head,int tail);
void init(int *head, int *tail);
void init(int *head, int *tail)
{*head = *tail = 0;}
void enqueue(int *q,int *tail, int element)
{ q[(*tail)++] = element;}
int dequeue(int *q,int *head)
{ return q[(*head)++];}
int full(int tail,const int size)
{return tail == size ? 1 : 0;}
int empty(int head, int tail)
{return head == tail ? 1 : 0;}
}
void main(){
int head,tail,element;
int queue[size];
init(&head,&tail);
// enqueue elements
while(full(tail,size) != 1){
element = rand();
printf("enqueue %d\n",element);
enqueue(queue,&tail,element);
printf("head=%d,tail=%d\n",head,tail);
}
printf("queue is full\n");
// dequeue elements from
while(!empty(head,tail)){
element = dequeue(queue,&head);
printf("dequeue element %d \n",element);
}
printf("queue is empty\n");
}
(1) Change it to an implementation in a circular fashion.
(2) Change it to handle the exceptions.
(3) Change the main() function, such that it
a. receives integers from the standard I/O and perform enqueue() operations until the queue is full.
b. performs one more enqueue() operation to show your program able to handle fullQueue exception.
c. prints out the size and all the elements in the queue to show the queue is full with totally 10 integers in it.
d. performs dequeue() operations until the queue is empty.
e. performs one more dequeue() operation to show your program is able to handle emptyQueue exception.
f. Implement a function deleteElement(Q, x) that deletes an element (integer) x from queue Q if x is in Q.
g. Call function deleteElement(Q, x) and show that it works correctly.
please check

Step by step
Solved in 3 steps

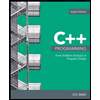
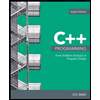