public class VisitorPackage { // Declare data fields: an int named travelerID, // a String named destination, and // a double named totalCost. // Your code here... // Implement the default contructor. // Set the value of destination to N/A, and choose an // appropriate value for the other data fields, // Your code here... // Implement the overloaded constructor that passes new // values to all data fields. // Your code here... // Implement method getTotalCost to return the totalCost. // Your code here... // Implement method applyDiscount. // // This method applies a discount off of the total cost // of the tour. The way the discount is applied depends // on the kind of visitor. // // Child discount: the unit discount is subtracted ONCE // from the total cost of the tour. // Senior discount: the unit discount is subtracted TWICE // from the total cost of the tour. // Parameters: // - a char to indicate the kind of visitor // ('c' for child, 's' for senior) // - a double that indicates the amount of the discount. // // Consider the case when the kind is neither 'c' or 's' // and output a message, "Discount cannot be applied." // // Does not return a value. public void applyDiscount(char kind, double discount) { // Your code here... // Implement method applyWeekdayRate // Subtracts the discount, computed from the percentage, // from the total cost. // // Parameter: a double to indicate a percentage (1.0 to 99.0) // // Example: If the tour cost so far is $150.00 and the percentage // is 10, then the tour cost will go down to $135.00. // // Does not return a value. public void applyWeekdayRate(double percentage) { // Your code here... // Implement method printInfo. // Prints out the visitor ID, the destination, and the // total cost of the tour. // // Output is formatted on one line separated by dashes // in the format: id - destination - $totalCost // // The total cost is formatted with 2 decimal places. // // Does not return a value. public void printInfo() { // Your code here... public class Z_RunVP { public static void main( String args[] ) { // GROUP 1: // John and Luke are going to Six Flags. // The price for a ticket is $65.00 per person. // John's id is 765, and Luke's id is 432. // They are going on a weekday. // Luke is a senior. // // 1. Declare two objects (john and luke) and initialize // their id, destination, and cost.... // 2. Use method applyWeekdayRate to apply a 20% discount // for both John and Luke.... // 3. Use method applyDiscount to apply a discount with // unit amount $12.50 for Luke. // Remember that Luke is a senior.... // 4. Print out the information for both John and Luke // using the printInfo method.... // 5. Calculate and output the total cost of their tour. // (Output the cost to two decimal places.) // Hint: Use the get method to get the total cost for // each and sum them up.... System.out.println(); // GROUP 2: // Peter, Paul, and Mary are going to Disneyland. // The price for the ticket is $99.00 per person. // Peter's id is 413, Paul's id is 189, and Mary's id is 516. // Paul and Mary are children. The unit discount is $8.95. // // 1. Declare and initialize three objects (peter, // paul, and mary).... // 2. Use method applyWeekdayRate to apply a 15% // discount for Peter and Paul. // 3. Use the method applyDiscount to apply the // child discount for Paul and Mary.... // 4. Print out the information for all three of them.... // 5. Calculate and output the total cost of // their tour (to 2 decimal places)....
public class VisitorPackage
{
// Declare data fields: an int named travelerID,
// a String named destination, and
// a double named totalCost.
// Your code here...
// Implement the default contructor.
// Set the value of destination to N/A, and choose an
// appropriate value for the other data fields,
// Your code here...
// Implement the overloaded constructor that passes new
// values to all data fields.
// Your code here...
// Implement method getTotalCost to return the totalCost.
// Your code here...
// Implement method applyDiscount.
//
// This method applies a discount off of the total cost
// of the tour. The way the discount is applied depends
// on the kind of visitor.
//
// Child discount: the unit discount is subtracted ONCE
// from the total cost of the tour.
// Senior discount: the unit discount is subtracted TWICE
// from the total cost of the tour.
// Parameters:
// - a char to indicate the kind of visitor
// ('c' for child, 's' for senior)
// - a double that indicates the amount of the discount.
//
// Consider the case when the kind is neither 'c' or 's'
// and output a message, "Discount cannot be applied."
//
// Does not return a value.
public void applyDiscount(char kind, double discount)
{
// Your code here...
// Implement method applyWeekdayRate
// Subtracts the discount, computed from the percentage,
// from the total cost.
//
// Parameter: a double to indicate a percentage (1.0 to 99.0)
//
// Example: If the tour cost so far is $150.00 and the percentage
// is 10, then the tour cost will go down to $135.00.
//
// Does not return a value.
public void applyWeekdayRate(double percentage)
{
// Your code here...
// Implement method printInfo.
// Prints out the visitor ID, the destination, and the
// total cost of the tour.
//
// Output is formatted on one line separated by dashes
// in the format: id - destination - $totalCost
//
// The total cost is formatted with 2 decimal places.
//
// Does not return a value.
public void printInfo()
{
// Your code here...
public class Z_RunVP
{
public static void main( String args[] )
{
// GROUP 1:
// John and Luke are going to Six Flags.
// The price for a ticket is $65.00 per person.
// John's id is 765, and Luke's id is 432.
// They are going on a weekday.
// Luke is a senior.
//
// 1. Declare two objects (john and luke) and initialize
// their id, destination, and cost....
// 2. Use method applyWeekdayRate to apply a 20% discount
// for both John and Luke....
// 3. Use method applyDiscount to apply a discount with
// unit amount $12.50 for Luke.
// Remember that Luke is a senior....
// 4. Print out the information for both John and Luke
// using the printInfo method....
// 5. Calculate and output the total cost of their tour.
// (Output the cost to two decimal places.)
// Hint: Use the get method to get the total cost for
// each and sum them up....
System.out.println();
// GROUP 2:
// Peter, Paul, and Mary are going to Disneyland.
// The price for the ticket is $99.00 per person.
// Peter's id is 413, Paul's id is 189, and Mary's id is 516.
// Paul and Mary are children. The unit discount is $8.95.
//
// 1. Declare and initialize three objects (peter,
// paul, and mary)....
// 2. Use method applyWeekdayRate to apply a 15%
// discount for Peter and Paul.
// 3. Use the method applyDiscount to apply the
// child discount for Paul and Mary....
// 4. Print out the information for all three of them....
// 5. Calculate and output the total cost of
// their tour (to 2 decimal places)....

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

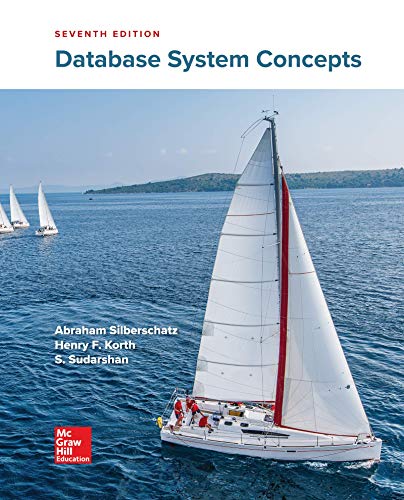
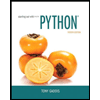
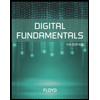
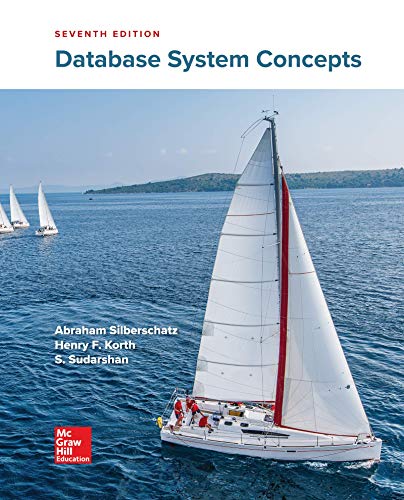
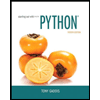
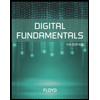
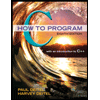
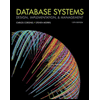
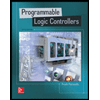