Homework Assignment Chapter 4b Create a Java Project folder named Chap4b Create a class named Student 1. The Student class will contain private instance data fields for these fields only: studentiD (String), test1 (int), test2 (int), test3 (int) 2. Define a final and static constant for the divisor. 3. Create one constructor with parameters for all instance data fields. 4. Create getters and setters for all instance data fields. 5. Provide a method called computeTotal() which does not receive any parameters. This method creates a total of all test scores and returns the integer total to the driver program. 6. Provide a method called computeAverage() which receives the total from the driver program. This method computes the average test score and returns the double average test score for an object to the driver program. Use the constant. 7. Create a displaylnfo() method that receives two parameters, the integer total and the double average being passed from the driver program. This method displays student ID, all test scores, the total, and the average test score. Use "\t" for indentation on some output lines. a. Even though technically unnecessary, use get methods or use the this reference to display the student ID and test scores. b. Use the total and the average test score passed to this method to display the total and the average.
Homework Assignment Chapter 4b Create a Java Project folder named Chap4b Create a class named Student 1. The Student class will contain private instance data fields for these fields only: studentiD (String), test1 (int), test2 (int), test3 (int) 2. Define a final and static constant for the divisor. 3. Create one constructor with parameters for all instance data fields. 4. Create getters and setters for all instance data fields. 5. Provide a method called computeTotal() which does not receive any parameters. This method creates a total of all test scores and returns the integer total to the driver program. 6. Provide a method called computeAverage() which receives the total from the driver program. This method computes the average test score and returns the double average test score for an object to the driver program. Use the constant. 7. Create a displaylnfo() method that receives two parameters, the integer total and the double average being passed from the driver program. This method displays student ID, all test scores, the total, and the average test score. Use "\t" for indentation on some output lines. a. Even though technically unnecessary, use get methods or use the this reference to display the student ID and test scores. b. Use the total and the average test score passed to this method to display the total and the average.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 1PE
Related questions
Question
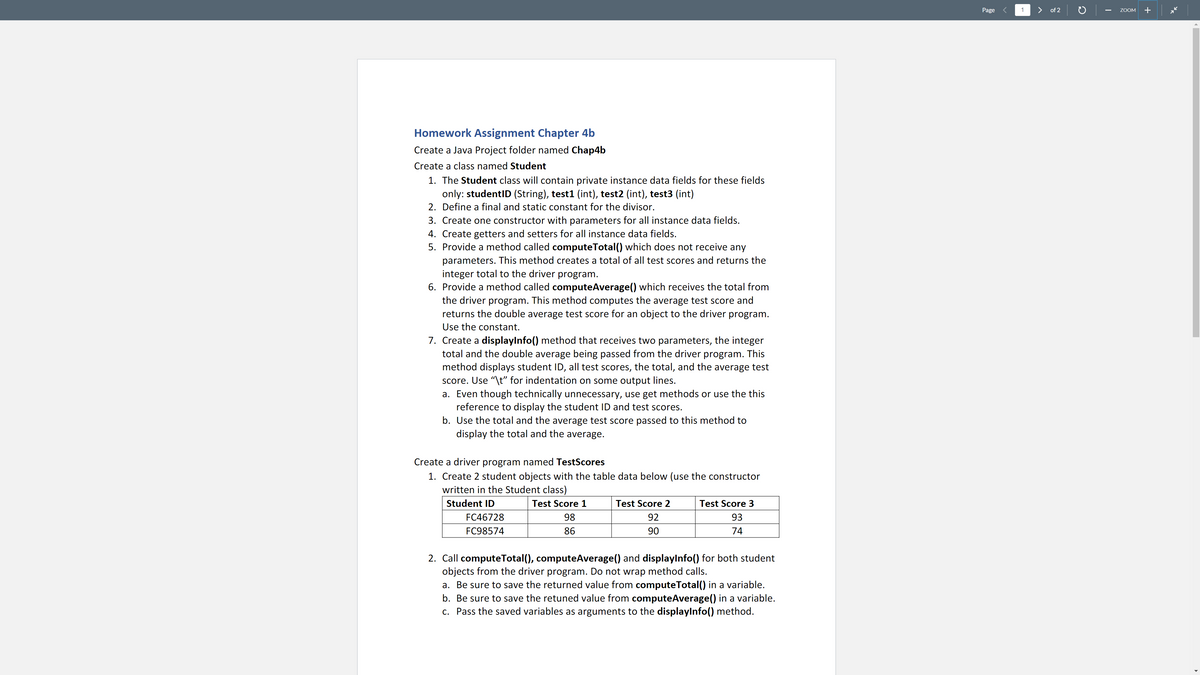
Transcribed Image Text:Page
1
> of 2
+
ZOOM
Homework Assignment Chapter 4b
Create a Java Project folder named Chap4b
Create a class named Student
1. The Student class will contain private instance data fields for these fields
only: studentID (String), test1 (int), test2 (int), test3 (int)
2. Define a final and static constant for the divisor.
3. Create one constructor with parameters for all instance data fields.
4. Create getters and setters for all instance data fields.
5. Provide a method called computeTotal() which does not receive any
parameters. This method creates a total of all test scores and returns the
integer total to the driver program.
6. Provide a method called computeAverage() which receives the total from
the driver program. This method computes the average test score and
returns the double average test score for an object to the driver program.
Use the constant.
7. Create a displaylnfo() method that receives two parameters, the integer
total and the double average being passed from the driver program. This
method displays student ID, all test scores, the total, and the average test
score. Use "\t" for indentation on some output lines.
a. Even though technically unnecessary, use get methods or use the this
reference to display the student ID and test scores.
b. Use the total and the average test score passed to this method to
display the total and the average.
Create a driver program named TestScores
1. Create 2 student objects with the table data below (use the constructor
written in the Student class)
Student ID
Test Score 1
Test Score 2
Test Score 3
FC46728
98
92
93
FC98574
86
90
74
2. Call computeTotal(), computeAverage() and displaylnfo() for both student
objects from the driver program. Do not wrap method calls.
a. Be sure to save the returned value from computeTotal() in a variable.
b. Be sure to save the retuned value from computeAverage() in a variable.
c. Pass the saved variables as arguments to the displaylnfo() method.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
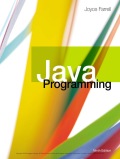
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
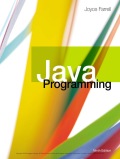
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT