Python program. Instructions are given within the quotations for each function. 1) def isValidKey(key): """ Returns True if key is a string that has 26 characters and each of the letter 'a'/'A'-'z'/'Z' appeared once and only once in either lower case or upper case. Returns False otherwise. """ 2) def replace(letter, key): """ Assume letter is a single characer string. Replace a single letter with its corresponding key, returns letter if it is not in the alphabet 'a'-'z' or 'A'-'Z' """ 3) def substitution(plainText, key): """ Returns encrypted string for the plainText using substitution encryption, which is to substitute 'a'/'A' with the lower/upper case of key[0], and substitute 'b'/'B' with the lower/upper case of key[1], and so on all the way to 'z'/'Z'. Leave all other non-alpha characters as it is in plainText. Your algorithm should be efficient so that it can run fairly quickly for very large strings. 1. use replace() to convert each letter to its encrypted letter 2. append encrypted letter to a list 3. use ''.join(list_of_letters) to form the final string. Do not use + to do string concatenation to form the encrypted string. """ 4) def run(): """ 1. Prompt user for a key; 2. Check if key is valid. If not, print an error message then return; if valid, prompt for a plain text to be encrypted with the valid key, 3. Send the plain text and valid key to substitution function. 4. Print out the encrypted message. """
Python program. Instructions are given within the quotations for each function. 1) def isValidKey(key): """ Returns True if key is a string that has 26 characters and each of the letter 'a'/'A'-'z'/'Z' appeared once and only once in either lower case or upper case. Returns False otherwise. """ 2) def replace(letter, key): """ Assume letter is a single characer string. Replace a single letter with its corresponding key, returns letter if it is not in the alphabet 'a'-'z' or 'A'-'Z' """ 3) def substitution(plainText, key): """ Returns encrypted string for the plainText using substitution encryption, which is to substitute 'a'/'A' with the lower/upper case of key[0], and substitute 'b'/'B' with the lower/upper case of key[1], and so on all the way to 'z'/'Z'. Leave all other non-alpha characters as it is in plainText. Your algorithm should be efficient so that it can run fairly quickly for very large strings. 1. use replace() to convert each letter to its encrypted letter 2. append encrypted letter to a list 3. use ''.join(list_of_letters) to form the final string. Do not use + to do string concatenation to form the encrypted string. """ 4) def run(): """ 1. Prompt user for a key; 2. Check if key is valid. If not, print an error message then return; if valid, prompt for a plain text to be encrypted with the valid key, 3. Send the plain text and valid key to substitution function. 4. Print out the encrypted message. """
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section4.3: Nested If Statements
Problem 7E
Related questions
Question
Python program. Instructions are given within the quotations for each function.
1) def isValidKey(key):
"""
Returns True if key is a string that has 26 characters and each of the letter
'a'/'A'-'z'/'Z' appeared once and only once in either lower case or upper case.
Returns False otherwise.
"""
2) def replace(letter, key):
"""
Assume letter is a single characer string.
Replace a single letter with its corresponding key, returns letter if it is
not in the alphabet 'a'-'z' or 'A'-'Z'
"""
3) def substitution(plainText, key):
"""
Returns encrypted string for the plainText using substitution encryption, which
is to substitute 'a'/'A' with the lower/upper case of key[0], and substitute
'b'/'B' with the lower/upper case of key[1], and so on all the way to 'z'/'Z'.
Leave all other non-alpha characters as it is in plainText.
Your algorithm should be efficient so that it can run fairly quickly for very
large strings.
1. use replace() to convert each letter to its encrypted letter
2. append encrypted letter to a list
3. use ''.join(list_of_letters) to form the final string. Do not use + to do
string concatenation to form the encrypted string.
"""
4) def run():
"""
1. Prompt user for a key;
2. Check if key is valid. If not, print an error message then return;
if valid, prompt for a plain text to be encrypted with the valid key,
3. Send the plain text and valid key to substitution function.
4. Print out the encrypted message.
"""
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
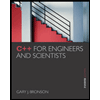
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
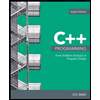
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
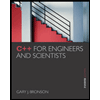
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
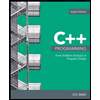
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning