Question and compiler output with error is provided in the attachment. And my solution is provided below. kindly correct my code and make it error-free also match output. -----MY SOLUTION----- MAIN.CPP #include #include #include #include #include #include #include #include"UserBO.cpp" using namespace std; int main() { int num; cout<<"Enter the number of users:"; cin>>num; string name; string contact; string uname; string password; User U[10]; for(int i = 0; i < num; i++) { cout<>name; cout<>contact; cout<>uname; cout<>password; User k(name, uname, password, contact); U[i] = k; } ofstream file; file.open("example.txt",ios::out); UserBO ub; ub.writeUserdetails(file, U, num); return 0; } UserBo.cpp #include #include #include #include #include #include #include #include"User.cpp" using namespace std; class UserBO { public: void writeUserdetails(ofstream &file, User obj[], int m) { for(int i = 0; i < m; i++) { file< #include #include #include #include #include #include using namespace std; class User { private: string name; string username; string password; string contactnumber; public: User(){} User(string name, string username, string password, string contactnumber) { this->name = name; this->username = username; this->password = password; this->contactnumber = contactnumber; } void setName(string name) { this->name = name; } void setUsername(string uname) { this->username = uname; } void setPassword(string pass) { this->password = pass; } void setContactnumber(string connum) { this->contactnumber = connum; } string getName() { return name; } string getUsername() { return username; } string getPassword() { return password; } string getContactNumber() { return contactnumber; } };
Question and compiler output with error is provided in the attachment. And my solution is provided below. kindly correct my code and make it error-free also match output. -----MY SOLUTION----- MAIN.CPP #include #include #include #include #include #include #include #include"UserBO.cpp" using namespace std; int main() { int num; cout<<"Enter the number of users:"; cin>>num; string name; string contact; string uname; string password; User U[10]; for(int i = 0; i < num; i++) { cout<>name; cout<>contact; cout<>uname; cout<>password; User k(name, uname, password, contact); U[i] = k; } ofstream file; file.open("example.txt",ios::out); UserBO ub; ub.writeUserdetails(file, U, num); return 0; } UserBo.cpp #include #include #include #include #include #include #include #include"User.cpp" using namespace std; class UserBO { public: void writeUserdetails(ofstream &file, User obj[], int m) { for(int i = 0; i < m; i++) { file< #include #include #include #include #include #include using namespace std; class User { private: string name; string username; string password; string contactnumber; public: User(){} User(string name, string username, string password, string contactnumber) { this->name = name; this->username = username; this->password = password; this->contactnumber = contactnumber; } void setName(string name) { this->name = name; } void setUsername(string uname) { this->username = uname; } void setPassword(string pass) { this->password = pass; } void setContactnumber(string connum) { this->contactnumber = connum; } string getName() { return name; } string getUsername() { return username; } string getPassword() { return password; } string getContactNumber() { return contactnumber; } };
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Question and compiler output with error is provided in the attachment. And my solution is provided below. kindly correct my code and make it error-free also match output.
-----MY SOLUTION-----
MAIN.CPP
#include<iostream> #include<string> #include<stdio.h> #include<fstream> #include<list> #include<iterator> #include<sstream> #include"UserBO.cpp" using namespace std; int main() { int num; cout<<"Enter the number of users:"; cin>>num; string name; string contact; string uname; string password; User U[10]; for(int i = 0; i < num; i++) { cout<<endl<<"Enter the name of user :"; cin>>name; cout<<endl<<"Enter the contact number :"; cin>>contact; cout<<endl<<"Enter the username :"; cin>>uname; cout<<endl<<"Enter the password :"; cin>>password; User k(name, uname, password, contact); U[i] = k; } ofstream file; file.open("example.txt",ios::out); UserBO ub; ub.writeUserdetails(file, U, num); return 0; } |
UserBo.cpp
#include<iostream> #include<string> #include<stdio.h> #include<fstream> #include<list> #include<iterator> #include<sstream> #include"User.cpp" using namespace std; class UserBO { public: void writeUserdetails(ofstream &file, User obj[], int m) { for(int i = 0; i < m; i++) { file<<obj[i].getName()<<","<<obj[i].getContactNumber()<<","<<obj[i].getUsername()<<","<<obj[i].getPassword()<<endl; } file.close(); } }; |
User.cpp
#include<iostream> #include<string> #include<stdio.h> #include<fstream> #include<list> #include<iterator> #include<sstream> using namespace std; class User { private: string name; string username; string password; string contactnumber; public: User(){} User(string name, string username, string password, string contactnumber) { this->name = name; this->username = username; this->password = password; this->contactnumber = contactnumber; } void setName(string name) { this->name = name; } void setUsername(string uname) { this->username = uname; } void setPassword(string pass) { this->password = pass; } void setContactnumber(string connum) { this->contactnumber = connum; } string getName() { return name; } string getUsername() { return username; } string getPassword() { return password; } string getContactNumber() { return contactnumber; } }; |
![ABC Bank needs to store the information of all the users. For a user, the information that the bank holds is the user's name, username, password, contact number. Read this data from
the console and store it in a file named "example.txt".
Write a C++ program to read inputs from the console and write the user details into a file as the comma-separated values.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified
in the problem statement. File names used should be the same as mentioned in the problem description.
The class named User has the following private member variables
Data type
string
string
string
string
Variable name
name
username
password
contactnumber
Define the following member function in the class UserBO.
Method name
Description
This function accepts a user object and an ofstream file
object. The function is used to write the data into the file.
void writeUserdetails(ofstream &file,User obj)
In the main method, read the input from the user through the console and open a file named "example.txt", into which the data are needed to be written. Pass the file object to the
writeUserdetails() function and write the inputs as comma-separated-values into the file.
Input and Output format:
Refer sample input and output for formatting specifications.
Sample input and output 1:
[All text in bold corresponds to input and rest corresponds to output]
Enter the name of user:
ramesh
Enter the contact number:
900900901
Enter the username:
ramesh123
Enter the password:
qwertyramesh
Datas written in file successfully
sample output in file: (example.txt)
ramesh,900900901,ramesh123,qwertyramesh](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe7652118-c626-4c01-801b-5e334e12a23b%2Feca3286f-c2ff-4d8c-9128-fa349c1821ff%2Fogd301o_processed.png&w=3840&q=75)
Transcribed Image Text:ABC Bank needs to store the information of all the users. For a user, the information that the bank holds is the user's name, username, password, contact number. Read this data from
the console and store it in a file named "example.txt".
Write a C++ program to read inputs from the console and write the user details into a file as the comma-separated values.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified
in the problem statement. File names used should be the same as mentioned in the problem description.
The class named User has the following private member variables
Data type
string
string
string
string
Variable name
name
username
password
contactnumber
Define the following member function in the class UserBO.
Method name
Description
This function accepts a user object and an ofstream file
object. The function is used to write the data into the file.
void writeUserdetails(ofstream &file,User obj)
In the main method, read the input from the user through the console and open a file named "example.txt", into which the data are needed to be written. Pass the file object to the
writeUserdetails() function and write the inputs as comma-separated-values into the file.
Input and Output format:
Refer sample input and output for formatting specifications.
Sample input and output 1:
[All text in bold corresponds to input and rest corresponds to output]
Enter the name of user:
ramesh
Enter the contact number:
900900901
Enter the username:
ramesh123
Enter the password:
qwertyramesh
Datas written in file successfully
sample output in file: (example.txt)
ramesh,900900901,ramesh123,qwertyramesh
![Compiler Output
TESTCASES
*A Sample snapshot of execution of your program for some of the inputs is given below, kindly check the 'Expected Output' and 'Obtained Output' to ensure there are no deviations (CAPS, SPACE, EXTRA PROMPTS and EXTRA LINES). There may be many other inputs for
which your program may fail.
Normal Test cases
S.No
Test
Expected
Obtained
Differences
1
Input: ramesh
example.txt
example.txt
File Contents dont match
900900901
File Not Found : example.txt
ramesh123
qwertyramesh
Output not matching
Test Script - main
S.No
Test
Expected
Obtained
Differences
1
Test Script - main
example.txt
example.txt
Input: ramesh
900900901
ramesh123
qwertyramesh
2
Test Script - main
example.txt
example.txt
Input: ramesh
900900901
ramesh123
qwertyramesh
3
Test Script - main
example.txt
example.txt
Input: jude
9876523410
jude_user
jude987654321
4
Test Script - main
example.txt
example.txt
Input: deepthi
8907890121
deepthi_c
deepu1234pass
5
Test Script - main
example.txt
example.txt
Input: jungkook
9878987889
jkjk
kooky
6
Test Script - main
example.txt
example.txt
Input: JK
7656765678
Compiler Error
jeon
kooky
Observations:
Compilation Errors Main.cpp: In function 'int main()': Main.cpp:68:36: error: no matching function for call to 'UserBO::writeUserdetails(std:ofstream&, User&)' obbo.writeUserdetails(fb,ob); ^ In file included
from Main.cpp:9:0: UserBO.cpp:13:6: note: candidate: void UserBO::writeUserdetails(std:ofstream&, User", int) void writeUserdetails(ofstream &file, User obj[], int m) ^-
UserBO.cpp:13:6:
note: candidate expects 3 arguments, 2 provided make: *** [test.o] Error 1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe7652118-c626-4c01-801b-5e334e12a23b%2Feca3286f-c2ff-4d8c-9128-fa349c1821ff%2Fs6qwuem_processed.png&w=3840&q=75)
Transcribed Image Text:Compiler Output
TESTCASES
*A Sample snapshot of execution of your program for some of the inputs is given below, kindly check the 'Expected Output' and 'Obtained Output' to ensure there are no deviations (CAPS, SPACE, EXTRA PROMPTS and EXTRA LINES). There may be many other inputs for
which your program may fail.
Normal Test cases
S.No
Test
Expected
Obtained
Differences
1
Input: ramesh
example.txt
example.txt
File Contents dont match
900900901
File Not Found : example.txt
ramesh123
qwertyramesh
Output not matching
Test Script - main
S.No
Test
Expected
Obtained
Differences
1
Test Script - main
example.txt
example.txt
Input: ramesh
900900901
ramesh123
qwertyramesh
2
Test Script - main
example.txt
example.txt
Input: ramesh
900900901
ramesh123
qwertyramesh
3
Test Script - main
example.txt
example.txt
Input: jude
9876523410
jude_user
jude987654321
4
Test Script - main
example.txt
example.txt
Input: deepthi
8907890121
deepthi_c
deepu1234pass
5
Test Script - main
example.txt
example.txt
Input: jungkook
9878987889
jkjk
kooky
6
Test Script - main
example.txt
example.txt
Input: JK
7656765678
Compiler Error
jeon
kooky
Observations:
Compilation Errors Main.cpp: In function 'int main()': Main.cpp:68:36: error: no matching function for call to 'UserBO::writeUserdetails(std:ofstream&, User&)' obbo.writeUserdetails(fb,ob); ^ In file included
from Main.cpp:9:0: UserBO.cpp:13:6: note: candidate: void UserBO::writeUserdetails(std:ofstream&, User", int) void writeUserdetails(ofstream &file, User obj[], int m) ^-
UserBO.cpp:13:6:
note: candidate expects 3 arguments, 2 provided make: *** [test.o] Error 1
Expert Solution

Step by step
Solved in 9 steps with 5 images

Recommended textbooks for you
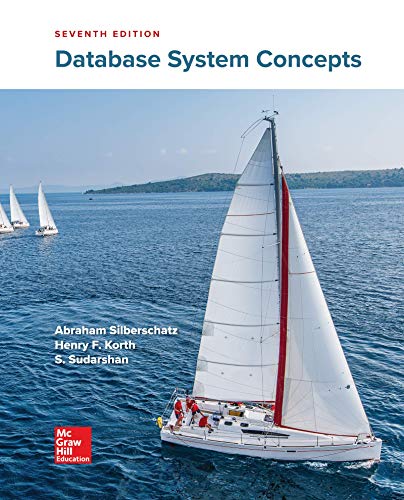
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
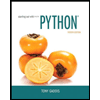
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
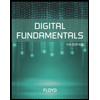
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
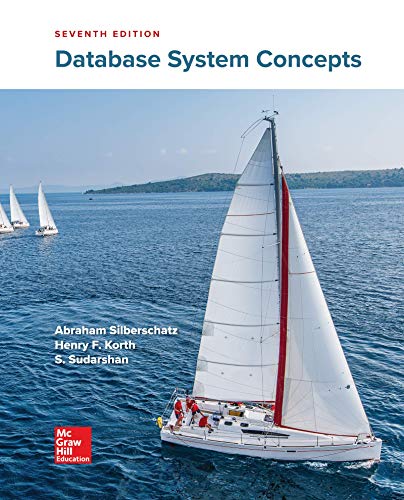
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
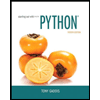
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
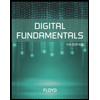
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
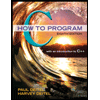
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
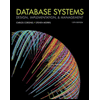
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
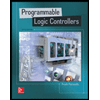
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education