or this problem set, you will submit a single java file named Homework.java. You are supposed to add the functions described below to your Homework class. The functional signatures of the functions you create must exactly match the signatures provided below. You are not allowed to use any 3rd party libraries in the homework assignment. When you have completed your homework, upload the Homework.java file to Grader Than. All tests will timeout and fail if your code takes longer than 10 seconds to complete. Remove Duplicates This function will receive a list of elements with duplicate elements, this function should remove the duplicate elements in the list and return a list without duplicate elements. The elements in the returned list must be in the same order that they were found in the list the function received. A duplicate element is an element found more than one time in the specified list. Signature:
java programming
For this problem set, you will submit a single java file named Homework.java. You are supposed to add the functions described below to your Homework class. The functional signatures of the functions you create must exactly match the signatures provided below. You are not allowed to use any 3rd party libraries in the homework assignment. When you have completed your homework, upload the Homework.java file to Grader Than. All tests will timeout and fail if your code takes longer than 10 seconds to complete.
Remove Duplicates
This function will receive a list of elements with duplicate elements, this function should remove the duplicate elements in the list and return a list without duplicate elements. The elements in the returned list must be in the same order that they were found in the list the function received. A duplicate element is an element found more than one time in the specified list.
Signature:
public static ArrayList<Object> removeDuplicates(ArrayList<Object> list)
Example:
INPUT: [2, 4, 5, 3, 3, 5, 2]
OUTPUT: [2, 4, 5, 3]
Duplicate Set
This function will receive a list of elements with duplicate elements. It should add all of the duplicate elements to a set and return the set containing the duplicate elements. A duplicate element is an element found more than one time in the specified list. The order of the set does not matter.
Signature:
public static HashSet<Object> duplicateSet(ArrayList<Object> list)
Example:
INPUT: [2, 4, 5, 3, 3, 5]
OUTPUT: {5, 3}
Remove Char
This function will be given a list of strings and a character. You must remove all occurrences of the character from each string in the list. The function should return the list of strings with the character removed.
Signature:
public static ArrayList<String> removeChar(String pattern, ArrayList<String> list)
Example:
list: ['adndj', 'adjdlaa', 'aa', 'djoe']
pattern: a
Output: ['dndj', 'djdl', '', 'djoe']
Return Growing NumList
This function will be given a single number, it should return a list of strings of numbers. Each string in the list will only contain a single number repeated an arbitrary amount of times. The number each string will contain will be equal to the current string's index+1. The number in the string should be repeated the same number of times as the string's index+1. Each number in the string should be separated by a space. This list should stop when its size equals the max number specified.
Signature:
public static ArrayList<String> returnGrowingNumList(int max)
Example:
INPUT: 3
OUTPUT: [1, 2 2, 3 3 3]
INPUT: 6
OUTPUT: [1, 2 2, 3 3 3, 4 4 4 4, 5 5 5 5 5, 6 6 6 6 6 6]
Unique Values
This function will receive a single map parameter known as a_map. a_map will contain a single letter as a string and numbers as values. This function is supposed to search a_map to find all values that appear only once in a_map. The function will create another map named to_ret. For all values that appear once in a_map, the function will add the value as a key in to_ret and set the value of the key to the key of the value in a_map (swap the key-value pairs, since a_map contains letters to numbers, to_ret will contain Numbers to Letters). Finally, the function should return to_ret.
Signature:
public static HashMap<String, Integer> uniqueValues(HashMap<Integer, String> a_map)
Example:
INPUT: {0=M, 2=M, 3=M, 5=M, 6=n, 7=M, 9=M}
OUTPUT: {n=6}
INPUT: {0=A, 1=c, 2=c, 4=c, 5=a, 8=Q, 9=c}
OUTPUT: {A=0, a=5, Q=8}
Concatenate Map
This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for adding
values to the map.
You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps.
Signature:
public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList)
Example:
INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]
OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93}
INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]
OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43}
INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29, g=44, x=59, y=8, z=82}]
OUTPUT: {p=79, b=10, g=28, h=21, x=59, y=8, z=62}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

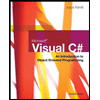
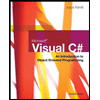