In the source file (CPP), create a C++ class that contains your functions and data members as follows: • Data members: · 2 arrays of strings named names and values respectfully (do not use STL containers e or your score will be reduced significantly). The size of the arrays should be large enough to hold all of the data parsed from the test files (we will be modifying this in the future so don't worry about the size being just right. Err on the side of too large if anything). • names array: will contain all of the names in the object values array: will contain all of the values in the object Member functions:
In the source file (CPP), create a C++ class that contains your functions and data members as follows: • Data members: · 2 arrays of strings named names and values respectfully (do not use STL containers e or your score will be reduced significantly). The size of the arrays should be large enough to hold all of the data parsed from the test files (we will be modifying this in the future so don't worry about the size being just right. Err on the side of too large if anything). • names array: will contain all of the names in the object values array: will contain all of the values in the object Member functions:
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter13: Overloading And Templates
Section: Chapter Questions
Problem 22SA
Related questions
Question
In C++ create a JSON parser, please leave meaningful comments explaining parts of the code.
![In the source file (CPP), create a C++ class that contains your functions and data members as follows:
• Data members:
· 2 arrays of strings named names and values respectfully (do not use STL containers e or your score will be
reduced significantly). The size of the arrays should be large enough to hold all of the data parsed from the
test files (we will be modifying this in the future so don't worry about the size being just right. Err on the side
of too large if anything).
• names array: will contain all of the names in the object
• values array: will contain all of the values in the object
Member functions:
· A function to open the file and store the file object or data. The function should return true on success and
false on failure.
· A function to read the names/values from the JSON file and place them in the 2 member arrays named
names and values respectfully. The function should return true on success and false on failure.
• Create docktest e testcases that tests the results of the parsing of JsonObject1.json L and JsonObject2.json L.
Here is an example:
/////// // Testing //////
TEST_CASE("Testing my JSON Parser")
{
JsonParser jp;
SUBCASE("testing parsing of empty JSON object")
{
// Open the file
CHECK(jp.OpenFile(DATA_FILE_PATH + "Jsonobjectl.json") == true);
// Invoke the parsing process
CHECK(jp.ParseJson() == true);
// Validate the parsed JSON object
CHECK(jp.names[0] == "");
CHECK(jp.values[0] == "");
}
SUBCASE("testing parsing of simple JSON object (only strings for values)")
{
// Open the file
CHECK(jp.OpenFile(DATA_FILE_PATH + "Jsonobject2.json") == true);
// Invoke the parsing process
CHECK(jp.ParseJson() == true);
// Validate the parsed JSON object
CHECK(jp.names[0] == "Name");
CHECK(jp.names[1] == "Symbol");
CHECK(jp.values[0]
CHECK(jp.values[1]
"BitCoin");
"BTC");
==](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5e137e43-f349-4a58-82f7-e9d3080ca5f4%2F6e04b64e-48dc-44f4-b229-b6d29aefe243%2F3fro0nr_processed.png&w=3840&q=75)
Transcribed Image Text:In the source file (CPP), create a C++ class that contains your functions and data members as follows:
• Data members:
· 2 arrays of strings named names and values respectfully (do not use STL containers e or your score will be
reduced significantly). The size of the arrays should be large enough to hold all of the data parsed from the
test files (we will be modifying this in the future so don't worry about the size being just right. Err on the side
of too large if anything).
• names array: will contain all of the names in the object
• values array: will contain all of the values in the object
Member functions:
· A function to open the file and store the file object or data. The function should return true on success and
false on failure.
· A function to read the names/values from the JSON file and place them in the 2 member arrays named
names and values respectfully. The function should return true on success and false on failure.
• Create docktest e testcases that tests the results of the parsing of JsonObject1.json L and JsonObject2.json L.
Here is an example:
/////// // Testing //////
TEST_CASE("Testing my JSON Parser")
{
JsonParser jp;
SUBCASE("testing parsing of empty JSON object")
{
// Open the file
CHECK(jp.OpenFile(DATA_FILE_PATH + "Jsonobjectl.json") == true);
// Invoke the parsing process
CHECK(jp.ParseJson() == true);
// Validate the parsed JSON object
CHECK(jp.names[0] == "");
CHECK(jp.values[0] == "");
}
SUBCASE("testing parsing of simple JSON object (only strings for values)")
{
// Open the file
CHECK(jp.OpenFile(DATA_FILE_PATH + "Jsonobject2.json") == true);
// Invoke the parsing process
CHECK(jp.ParseJson() == true);
// Validate the parsed JSON object
CHECK(jp.names[0] == "Name");
CHECK(jp.names[1] == "Symbol");
CHECK(jp.values[0]
CHECK(jp.values[1]
"BitCoin");
"BTC");
==
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
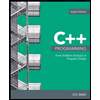
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
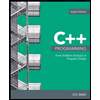
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning