ramm Please output it in the console not a text file. For this exercise, you will define a struct. It will contain a first name, last name, ID, and GPA. You will ask the user how many students to enter, create a dynamic array of that size to get data from the user. The array data will initially be inserted into the array in ID order. Then you will display the array in a table, sort it by last hame with std:sort, redisplay it, and then search for five students (using last name and a binary search) and for any names found display the first name, last name, and GPA. The struct will be named Student and include strings for firstName, lastName, ID, and a float for the GPA. The ID will be in the format 00xxxXXX where the x's are replaced by digits and in the range between 1i1111 and 999999. The GPA will be a floating-point number in the range 2.0 to 4.2 and will be displayed to 2 digits
ramm Please output it in the console not a text file. For this exercise, you will define a struct. It will contain a first name, last name, ID, and GPA. You will ask the user how many students to enter, create a dynamic array of that size to get data from the user. The array data will initially be inserted into the array in ID order. Then you will display the array in a table, sort it by last hame with std:sort, redisplay it, and then search for five students (using last name and a binary search) and for any names found display the first name, last name, and GPA. The struct will be named Student and include strings for firstName, lastName, ID, and a float for the GPA. The ID will be in the format 00xxxXXX where the x's are replaced by digits and in the range between 1i1111 and 999999. The GPA will be a floating-point number in the range 2.0 to 4.2 and will be displayed to 2 digits
New Perspectives on HTML5, CSS3, and JavaScript
6th Edition
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Patrick M. Carey
Chapter14: Exploring Object-based Programming: Designing An Online Poker
Section14.1: Visual Overview: Custom Objects, Properties, And Methods
Problem 7QC
Related questions
Question
100%

Transcribed Image Text:Programming Language: C++
Please output it in the console not a text file.
For this exerc ise, you will define a struct. It will contain a first name, last name, ID, and GPA. You will ask the user how many students to enter, create a dynamic
array of that size to get data from the user. The array data will initially be inserted into the array in ID order. Then you will display the array in a table, sort it by last
name with std::sort, redisplay it, and then search for five students (using last name and a binary search) and for any names found display the first name, last name,
and GPA,
The struct will be named Student and include strings for firstName, lastName, ID, and a float for the GPA. The ID will be in the format 00xxxxxx where the x's are
replaced by digits and in the range between 111111 and 999999. The GPA will be a floating-point number in the range 2.0 to 4.2 and will be displayed to 2 digits
after the decimal point.
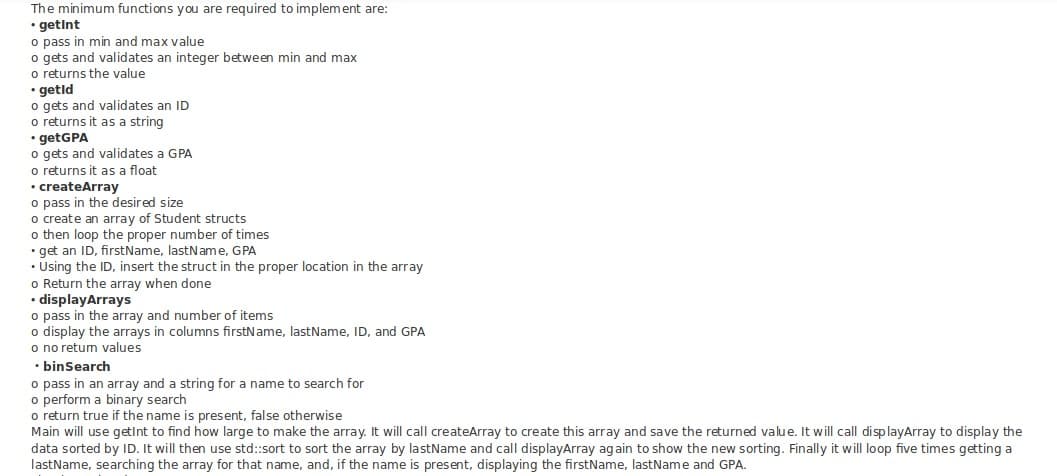
Transcribed Image Text:The minimum functions you are required to implement are:
• getint
o pass in min and max value
o gets and validates an integer between min and max
o returns the value
getld
o gets and validates an ID
o returns it as a string
getGPA
o gets and validates a GPA
o returns it as a float
• createArray
o pass in the desired size
o create an array of Student structs
o then loop the proper number of times
• get an ID, firstName, lastName, GPA
• Using the ID, insert the struct in the proper location in the array
o Return the array when done
displayArrays
o pass in the array and number of items
o display the arrays in columns firstName, lastName, ID, and GPA
o no retum values
• binSearch
o pass in an array and a string for a name to search for
o perform a binary search
o return true if the name is present, false otherwise
Main will use getInt to find how large to make the array. It will call createArray to create this array and save the returned value. It will call displayArray to display the
data sorted by ID. It will then use std::sort to sort the array by lastName and call displayArray again to show the new sorting. Finally it will loop five times getting a
lastName, searching the array for that name, and, if the name is present, displaying the firstName, lastName and GPA.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
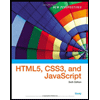
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
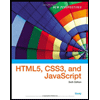
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning