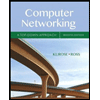
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Create a C# application named ShapesDemo that creates several objects that descend from an abstract class called GeometricFigure. Each GeometricFigure includes a height, a width, and an area.
Provide get and set accessors for each field except area; the area is computed and is read-only. Include an abstract method called ComputeArea() that computes the area of the GeometricFigure.
Next you will create three additional classes derived from the GeometricFigure class. Name these derived classes: Rectange, Square, and Triangle.
using static System.Console;
class ShapesDemo
{
static void Main()
{
// Your code here
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- Write an application for Nina’s Cookie Emporium named CookieDemo that declares and demonstrates objects of the CookieOrder class and its descendants. The CookieOrder class includes the following auto-implemented properties: OrderNum - The order number Name - The recipient’s name CookieType - The cookie type (for example, chocolate chip) The class should also include fields for number of dozens ordered and price (named Dozens and Price). When the field value for number of dozens ordered is set, the price field is set as $15 per dozen for the first two dozen and $13 per dozen for each dozen over two. Next, create a child class named SpecialCookieOrder, which includes a field with a description as to why the order is special (for example, gluten-free). Override the method that sets a CookieOrder’s price as described in the step above, but also to include special handling, which is $10 for orders up to $40 and $8 for higher-priced orders. An example of the program is shown below: Order…arrow_forward3. Design a user-defined class called Course. Each course object will hold data about a course. The data includes courseID, courseName, credits and instructorName. Define the instance variables required of the appropriate type. Provide only get methods along with toString() and equals() methods. Design another user-defined class called Student. Each student object will hold data on name, GPA and courseList. Make sure to define courseList as an ArrayList holding references to Course objects Implement a constructor that will take values to initialize name, and gpa while courseList is initialized to an ArrayList() object. Provide the following get methods: getStudent ID ( ), getName(), getGPA (), get Gender(), and get CourseList() Note: the getCouresList() method will return a copy of the coureList arraylist with copies of course objects. Provide the following set methods: setName(), setGPA (), setGender() Another method: addCourse () that will take a Course object and add it to the…arrow_forwardDevelop a set of classes for a college to use in various student service andpersonnel applications. Classes you need to design include the following:• Person—A Person contains a first name, last name, street address, zip code,and phone number. The class also includes a method that sets each datafield, using a series of dialog boxes and a display method that displays all of aPerson’s information on a single line at the command line on the screenarrow_forward
- Write a program IN C# named SalespersonDemo that instantiates objects using classes named RealEstateSalesperson and GirlScout. Demonstrate that each object can use a SalesSpeech() method appropriately. Also, use a MakeSale() method two or three times with each object, and display the final contents of each object’s data fields. First, create an abstract class named Salesperson. Fields include firstName and lastName; the Salesperson constructor requires both these values. Include properties for the fields. Include a method called GetName that returns a string that holds the Salesperson’s full name—the first and last names separated by a space. Then perform the following tasks: Create two child classes of Salesperson: RealEstateSalesperson and GirlScout. The RealEstateSalesperson class contains fields for the following: TotalValueSold - The total value sold in dollars (an int initialized to 0) TotalCommissionEarned - Total commission earned (a double initialized to 0) CommissionRate -…arrow_forwardStep 2 ShapesDemo.cs 1 using static System.Console; 2 class ShapesDemo 3 { static void Main() Create a Rectangle is a GeometricFigure 4 whose area is { // Your code here } 8 } determined by multiplying width by height. 7 Create a Square is a Rectangle in which the width and height are the same. Provide a constructor that accepts both height and width, forcing them to be equal if they are not. Provide a second constructor that accepts just one dimension and uses it for both height and width. The Square class uses the Rectangle 's ComputeArea() method. Create a Triangle is a GeometricFigure whose area is determined by multiplying the width by half the height. In the ShapesDemo class , after each object is created, pass it to a method that accepts a GeometricFigure argument in which the figure's data is displayed. Change some dimensions of some of the figures, and pass each to the display method again.arrow_forwardInheritance and polmorphism: In Java, Using Classes, desine an online address book to keep track of the names , addresses phone numbers, and birthdays of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries. A. Define the class Address that can store a street address, city, state, and zip code. Use the appropriae methods to print and store the address. Also, use consructors to automatically initialize the data members. B. Define the class ExtPerson using the class Person (designed in chapter 8, example 8-8), the class Date, and the class Address. Add a data member to the class to claddify the person as a family member, friend, or business associate. Also, add a data member to store the phone number. Add (or override) methods to print and store the appropriate informatiom. Use constructors to automatically omotoa;ize the data members. C. Define the class AddressBook using previously defined classes. An object of…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
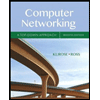
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
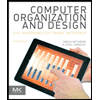
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
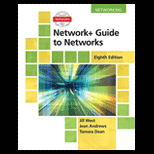
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
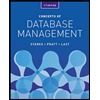
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
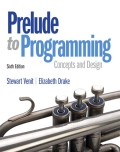
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
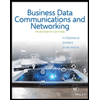
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY