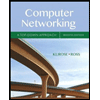
package hw1;
import java.util.Arrays;
import dsUtils.WordReader;
public class WordFrequencyAnalyzer {
/**********************************************************************************/
/* You are not allowed to add any fields to this class beyond the one given below */
/* You may only read in from the file once. This means you may only use a single */
/* word reader object. */
/**********************************************************************************/
// Currently, the field counters is not used.
// Your task is to make this class more efficent by storing the word counts
// as a symbol table / map / dictionary in the field counters.
private SequentialSearchST<String, Integer> counters;
private String filename;
/**
* Stores a count of the number of times any word appears in a file. The file is
* read in exactly once at the time time this object is constructed.
* (You need to modify the constructor to do this.)
*
* @param filename the name of the file on which to count all word occurrences.
*/
public WordFrequencyAnalyzer(String filename) {
this.filename = filename;
}
/**
* Returns the number of times a given word appears in the file from which this
* object was created.
* (Currently, it reads through the entire file to count the number of times
* the given word appears. Modify this to instead use the information stored
* in the counters field.)
*
* @param word the word to count
* @return the number of times <code>word</code> appears.
*/
public int getCount(char[] word) {
WordReader r = new WordReader(filename);
int count = 0;
for(char[] w : r) {
if (Arrays.equals(w, word))
count++;
}
return count;
}
/**
* Returns the maximum frequency over all words in the file from which this
* object was created.
* (Currently, this method is not implemented. You must implement it.)
*
* @return the maximum frequency of any word in the the file.
*/
public int maxCount() {
throw new RuntimeException("Not implemented.");
}
}
Only solve for maxCount

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

What would be the print Statement for the code above?
What would be the print Statement for the code above?
- USING C++ Implement the Rectangle class below: *******RECTANGLE CLASS******* class Rectangle { private: double width; double length; public: void setWidth(double); void setLength(double); double getWidth() const; double getLength() const; double getArea() const; }; *******END OF RECTANGLE CLASS******* Enhance the Rectangle class with two new member functions: double getPerimeter() - returns the perimeter of the rectangle, i.e. 2*width+2*length bool isSquare() - returns true if the rectangle is a square, i.e. length is equal to width, returns false if not Demonstrate your class works with the main function that instantiates a Rectangle object and shows sample output for these functions workingarrow_forwardPlease help me make a game with Java. The game should have only a class and needs to include at least 3 of the following AP topics: String Array, ArrayList, 2D Array Interfaces and/or Abstract classes Polymorphism Inheritance Searching Algorithms Sorting Algorithms Recursion You also need to include a new topic that is different than the above. Once you finish creating the game, please show me the new topic that you included and let me know what are the other 3 topics that you chose from the list above and what was challenging.arrow_forwardModify the unsortedArrayAccess class by adding a method that find the smallest element and average in the array. import java.util.*; public class unsortedArrayAccess{ public static double[] arr; public int arraySize; public unsortedArrayAccess(int scale) { arr = new double[scale]; arraySize = 0; } public int search(double Key) { int i = 0; while ((i < arraySize) && (arr[i] != Key) ) { i = i + 1; } if (i < arraySize) { return i; } else { System.out.println("There is no such item!"); return -1; } } public void append(double Item) { arr[arraySize] = Item; arraySize = arraySize + 1; } public double remove() { if (arraySize == 0) { System.out.println("There is no item in the array!"); return -1; } double x = arr[arraySize - 1]; arraySize = arraySize - 1; return x; } public void deletion(double Key) { int k = search(Key); if (k != -1) { for (int i = k; i…arrow_forward
- See ERROR in Java file 2: Java file 1: import java.util.Random;import java.util.Scanner; public class Account {// Declare the variablesprivate String customerName;private String accountNumber;private double balance;private int type;private static int numObjects;// constructorpublic Account(String customerName, double balance, int type) { this.customerName = customerName;this.balance = balance;this.type = type;setaccountNumber(); // set account number functionnumObjects += 1;}private void setaccountNumber() // definition of set account number{Random rand = new Random();accountNumber = customerName.substring(0, 3).toUpperCase();accountNumber += type;accountNumber += "#";accountNumber += (rand.nextInt(100) + 100);}// function to makedepositvoid makeDeposit(double amount){if (amount > 0){balance += amount;}}// function for withdrawboolean makeWithdrawal(double amount) {if (amount < balance)balance -= amount;elsereturn false;return true;}public String toString(){return accountNumber +…arrow_forwardImplement move the following way. public int[] walk(int... stepCounts) { // Implement the logic for walking the players returnnewint[0]; } public class WalkingBoardWithPlayers extends WalkingBoard{ privatePlayer[] players; privateintround; publicstaticfinalintSCORE_EACH_STEP=13; publicWalkingBoardWithPlayers(int[][] board, intplayerCount) { super(board); initPlayers(playerCount); } publicWalkingBoardWithPlayers(intsize, intplayerCount) { super(size); initPlayers(playerCount); } privatevoidinitPlayers(intplayerCount) { if(playerCount <2){ thrownewIllegalArgumentException("Player count must be at least 2"); } else { this.players=newPlayer[playerCount]; this.players[0] =newMadlyRotatingBuccaneer(); for (inti=1; i < playerCount; i++) { this.players[i] =newPlayer(); } } } package walking.game.player; import walking.game.util.Direction; public class Player{ privateintscore; protectedDirectiondirection=Direction.UP; publicPlayer() {} publicintgetScore() { return score; }…arrow_forwardjava programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt? I would like the physical receipt to export as text file, not print the console's order receipt. import java.util.*; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and pricesString[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"};double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99};StringusersName; // The user's name, as entered by the user.ArrayList<String> arr = new ArrayList<>(); // Define scanner objectScanner input=new Scanner(System.in); // Welcome messageSystem.out.println("Welcome to AppleStoreRecreation, what is your name:");usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to meet you!, May I Take Your Order?"); // Display menu items and…arrow_forward
- import java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forwardUsing the code in the image provided: Implement a method called summation which adds two integers and returns their sum. INPUT: The first line of input contains an integer a. The Second Line of input containes and integer b. OUTPUT: Print the result which is the sum of a and b.arrow_forwardupper limit. Define the class Random Integer in full.arrow_forward
- package lab1; /** * A utility class containing several recursive methods * * <pre> * * For all methods in this API, you are forbidden to use any loops, * String or List based methods such as "contains", or methods that use regular expressions * </pre> * * */ public final class Lab1 { /** * This is empty by design, Lab class cannot be instantiated */ privateLab1(){ // empty by design } /** * Returns the product of a consecutive set of numbers from <code> start </code> * to <code> end </code>. * * @param start is an integer number * @param end is an integer number * @return the product of start * (start + 1) * ..*. + end * @pre. * <code> start </code> and <code> end </code> are small enough to let * this method return an int. This means the return value at most * requires 4 bytes and no overflow would happen. */ publicstaticintproduct(ints,inte){ if(s==e){ returne; }else{ returns*product(s+1,e); } }…arrow_forward*JAVA* complete method Delete the largest valueremoveMax(); Delete the smallest valueremoveMin(); class BinarHeap<T> { int root; static int[] arr; static int size; public BinarHeap() { arr = new int[50]; size = 0; } public void insert(int val) { arr[++size] = val; bubbleUP(size); } public void bubbleUP(int i) { int parent = (i) / 2; while (i > 1 && arr[parent] > arr[i]) { int temp = arr[parent]; arr[parent] = arr[i]; arr[i] = temp; i = parent; } } public int retMin() { return arr[1]; } public void removeMin() { } public void removeMax() { } public void print() { for (int i = 0; i <= size; i++) { System.out.print( arr[i] + " "); } }} public class BinarH { public static void main(String[] args) { BinarHeap Heap1 = new BinarHeap();…arrow_forwardusing java *two classes*arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
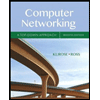
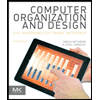
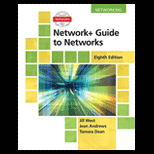
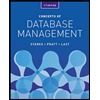
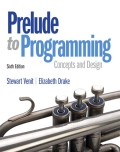
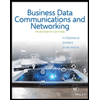