So I have this code (in C++) and I'm not sure what I'm getting wrong with the actual code. I need to make it so the letter that the code outputs DOES NOT count characters and that the code chooses the letter that is earliest in the alphabet. (I added some examples at the bottom) For this problem you must create a program that accepts a line of English text as input. The string may contain spaces, so make sure you read the entire line of input. Next your program should count the occurrences of each letter of the English alphabet and determine which letter occurs most frequently in the string. Print this letter to the screen. If two letters tie for the most frequent count, break the tie by choosing the letter that occurs first in the alphabet. For example if the input string was "aabb", then your program should identify 'a' as the most frequently occurring letter. Finally, remove all occurrences of this most frequently occurring letter by replacing them with the '-' character, and print the final string to the screen. HINT: Since ascii characters are stored as 8 bit ints "under the hood", and they are also represented in order (i.e. 'a' comes before 'b'); we can use them both as characters as well as for counting. Thus if we wanted to loop through all of the lowercase letters of the alphabet we could do something like this: for(char currentLetter = 'a'; currentLetter <= 'z'; currentLetter++){ //interesting code goes here... } Sample Input aaaab Sample Output a ----b Simplifying Assumptions You may assume that all letters are lowercase You only need to count the letters of the alphabet (i.e. don't worry about counting special characters like punctuation, digits, etc.) If two letters tie for the most frequent count, break the tie by choosing the letter that occurs first in the alphabet. MY CODE #include <iostream>#include <string>#include <vector>#include <cctype>#include<bits/stdc++.h>using namespace std; string replaceLetter(string originalString, char character){ cout << character << endl;int originalLength = originalString.length(); for (int i = 0; i < originalLength; i++){ if (originalString[i] == character){ originalString[i] = '-'; } } return originalString;} int main(){int arr[26] = { 0 }; string s; getline(cin, s); string s1=s; //sort(s.begin(),s.end()); int originalLength = s.length(); int i = 0; int max_count = 0; int temp = 0; for ( i = 0; i < originalLength; i++){ temp = tolower(s[i]) - 97; arr[temp] ++; if (arr[temp] > max_count){ max_count = arr[temp]; } } vector<int> lettersWithMaxCount; for (i = 0; i < 26; i++){ if (arr[i] == max_count){ lettersWithMaxCount.push_back(i + 97);} } if (lettersWithMaxCount.size() == 1){ s = replaceLetter(s, lettersWithMaxCount[0]); } else{for (i = 0; i < originalLength; i++){ temp = s[i]; bool found = false; int result; for (std::vector<int>::iterator it = lettersWithMaxCount.begin();it != lettersWithMaxCount.end(); ++it){ if (*it == temp){ result = temp; found = true;break; } } if (found){ s = replaceLetter(s1, temp); cout << s << endl;return 0; } } } cout << s << endl; return 0;} EXAMPLE OUTPUT Output differs. Input : This is a sentence. Your output: s Thi- i- a -entence. Expected output: e This is a s-nt-nc-. EXAMPLE 2: Input: this??? Your output: this??? Expected output: h t-is??? Thanks!
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
So I have this code (in C++) and I'm not sure what I'm getting wrong with the actual code. I need to make it so the letter that the code outputs DOES NOT count characters and that the code chooses the letter that is earliest in the alphabet. (I added some examples at the bottom)
For this problem you must create a program that accepts a line of English text as input. The string may contain spaces, so make sure you read the entire line of input. Next your program should count the occurrences of each letter of the English alphabet and determine which letter occurs most frequently in the string. Print this letter to the screen. If two letters tie for the most frequent count, break the tie by choosing the letter that occurs first in the alphabet. For example if the input string was "aabb", then your program should identify 'a' as the most frequently occurring letter.
Finally, remove all occurrences of this most frequently occurring letter by replacing them with the '-' character, and print the final string to the screen.
HINT:
Since ascii characters are stored as 8 bit ints "under the hood", and they are also represented in order (i.e. 'a' comes before 'b'); we can use them both as characters as well as for counting. Thus if we wanted to loop through all of the lowercase letters of the alphabet we could do something like this:
for(char currentLetter = 'a'; currentLetter <= 'z'; currentLetter++){ //interesting code goes here... }
Sample Input
aaaab
Sample Output
a ----b
Simplifying Assumptions
- You may assume that all letters are lowercase
- You only need to count the letters of the alphabet (i.e. don't worry about counting special characters like punctuation, digits, etc.)
- If two letters tie for the most frequent count, break the tie by choosing the letter that occurs first in the alphabet.
MY CODE
#include <iostream>
#include <string>
#include <
#include <cctype>
#include<bits/stdc++.h>
using namespace std;
string replaceLetter(string originalString, char character){
cout << character << endl;
int originalLength = originalString.length();
for (int i = 0; i < originalLength; i++){
if (originalString[i] == character){
originalString[i] = '-';
}
}
return originalString;
}
int main(){
int arr[26] = { 0 };
string s;
getline(cin, s);
string s1=s;
//sort(s.begin(),s.end());
int originalLength = s.length();
int i = 0;
int max_count = 0;
int temp = 0;
for ( i = 0; i < originalLength; i++){
temp = tolower(s[i]) - 97;
arr[temp] ++;
if (arr[temp] > max_count){
max_count = arr[temp];
}
}
vector<int> lettersWithMaxCount;
for (i = 0; i < 26; i++){
if (arr[i] == max_count){
lettersWithMaxCount.push_back(i + 97);
}
}
if (lettersWithMaxCount.size() == 1){
s = replaceLetter(s, lettersWithMaxCount[0]);
}
else{
for (i = 0; i < originalLength; i++){
temp = s[i];
bool found = false;
int result;
for (std::vector<int>::iterator it = lettersWithMaxCount.begin();
it != lettersWithMaxCount.end(); ++it){
if (*it == temp){
result = temp;
found = true;
break;
}
}
if (found){
s = replaceLetter(s1, temp);
cout << s << endl;
return 0;
}
}
}
cout << s << endl;
return 0;
}
EXAMPLE OUTPUT

Step by step
Solved in 3 steps with 3 images

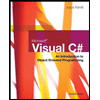
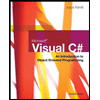