Suppose you have a class Rectangle with data members width and height. The public member functions of class Rectangle are as follows: setValues(int w, int h); // to set values of width and height of objects of Rectangle class int Area();// to find area of an object of rectangle class biggerRectangle(Rectangle A, Rectangle B); prints the rectangle details which is bigger.
NOTE: All questions attempted in visual studio.
Question 1:
Need C++ code
Suppose you have a class Rectangle with data members width and height. The public member functions of class Rectangle are as follows:
- setValues(int w, int h); // to set values of width and height of objects of Rectangle class
- int Area();// to find area of an object of rectangle class
- biggerRectangle(Rectangle A, Rectangle B); prints the rectangle details which is bigger.
Question2 :
Find errors and rewrite the code and show output
C++
#include <iostream>
using namespace std;
class CRectangle {
int width. height;
public:
void set_values (int, int);
int area () {return (width * height);}
};
void CRectangle::set_values (int a, int b) {
width = a;
height = b;
}
int main () {
CRectangle a,*b,*c;
c= a;
a.set_values (1,2);
b= new CRectangle;
c=&b;
c->set_values (3,4);
cout << "a area: " << c->area() << endl;//
cout << "*b area: " << a.area() << endl;//
c->set_values (5,6);
cout << "*b area: " << b.area() << endl;//
delete b;
return 0;
}
Question3:
Run the following code in Visual Studio and see if it generates an exception or not? If it generates an exception, then why is it doing so. Write the differences between static and dynamic objects as well.
#include <iostream>
using namespace std;
class CRectangle {
int width, height;
public:
void set_values (int, int);
int area () {return (width * height);}
};
void CRectangle::set_values (int a, int b) {
width = a;
height = b;
}
int main () {
CRectangle a;// static object
CRectangle* b=new CRectangle;//dynamic object
a.set_values (1,2);
b->set_values (3,4);
cout << "*b area: " << b->area() << endl;//12
cout << "*b area: " << b->area() << endl;//12
delete b;
cout << "*b area: " << b->area() << endl;//12
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

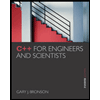
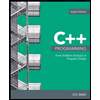
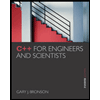
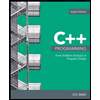