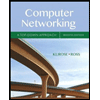
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Answer this in C++:
In the Student.cpp file and Student.h file, build the Student class with the following specifications:
- Private data members
- string name - Initialized in default constructor to "Louie"
- double gpa - Initialized in default constructor to 1.0
- Default constructor
- Public member functions
- SetName() - sets the student's name
- GetName() - returns the student's name
- SetGPA() - sets the student's GPA
- GetGPA() - returns the student's GPA
Ex. If a new Student object is created, the default constructor sets name to "Louie" and gpa to 1. The output of GetName() and GetGPA() before and after calling SetName("Felix") and SetGPA(3.7) is:
Louie/1
Felix/3.7
main.cpp
#include <iostream>
#include <vector>
#include "Student.h"
using namespace std;
int main() {
Student student = Student();
cout << student.GetName() << "/" << student.GetGPA() << endl;
student.SetName("Felix");
student.SetGPA(3.7);
cout << student.GetName() << "/" << student.GetGPA() << endl;
return 0;
}
Student.h
#ifndef STUDENT_H_
#define STUDENT_H_
#include <iostream>
using namespace std;
class Student {
public:
Student();
void SetName(string n);
// FIXME: Declare 3 more functions
private:
// FIXME: Add 2 private data members
};
#endif /* STUDENT_H_ */
Student.cpp
// Simple class to hold student information
#include "Student.h"
#include <iostream>
#include <string>
using namespace std;
Student::Student() {
//FIXME: complete the constructor
}
void Student::SetName(string n) {
//FIXME: complete SetName function
}
// FIXME: Add 3 more functions
Expert Solution

arrow_forward
Step 1
The answer is given below:-
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

Knowledge Booster
Similar questions
- Select the statement that is NOT true about static and dynamic binding. Question options: a. dynamic binding occurs at run time b. static binding occurs at compile time c. pass by reference is an example of dynamic binding d. pass by reference is an example of static bindingarrow_forwardC++arrow_forwardC++ Write the class declaration for StorageFile and declare (no definitions) the following members:arrow_forward
- Summary In this lab, you create a derived class from a base class, and then use the derived class in a Python program. The program should create two Motorcycle objects, and then set the Motorcycle’s speed, accelerate the Motorcycle object, and check its sidecar status. Instructions Open the file named Motorcycle.py. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class __init()__ method inside the Motorcycle class's __init()__ method. In theMotorcycle class, create an attribute named sidecar. Write a public set method to set the value for sidecar. Write a public get method to retrieve the value of sidecar. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the message in the accelerate method so the following is displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This motorcycle cannot go that fast". Open the file named MyMotorcycleClassProgram.py. In the…arrow_forwardWRITE IN C++ A Date class is described by month(m), day(d), year(y). An Employee is described by a unique data structure having a name (e.g. “George”), salary(75000.25) and date_of_birth (06, 10, 1998). Calculate the number of days between his day of birth and (09, 20, 2023). Assume that the Employee object contains a Date sub-object)arrow_forwardUsing C++: Write a program that creates a Bus class that includes a bus ID number, number of seats, driver name, and current speed. Create a default constructor without any parameters that initializes the value of ID number, number of seats, and driver name. Create another constructor with three parameters of bus id number, number of seats, and driver name. Car speed should always be initialized as 0. The program will also include the member functions to perform the various operations: modify, set, and display bus information (Bus id, number of seats, driver name, and current speed). For example:- Set the bus id number- Set the number of seats- Set of driver number----------------------------------------------------- Return the bus id number by using get method- Return the number of seats- Return of driver number- Return the current speed-----------------------Member function to display the bus information- busInformation() Also write two member functions, Accelerate() and Brake().…arrow_forward
- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardAnswer this iin C++: Given main(), complete the Calculator class (in files Calculator.h and Calculator.cpp) that emulates basic functions of a calculator: add, subtract, multiple, divide, and clear. The class has one private data member called value for the calculator's current value. Implement the following constructor and public member functions as listed below: Calculator() - default constructor to set the data member to 0.0 void Add(double val) - add the parameter to the data member void Subtract(double val) - subtract the parameter from the data member void Multiply(double val) - multiply the data member by the parameter void Divide(double val) - divide the data member by the parameter void Clear( ) - set the data member to 0.0 double GetValue( ) - return the data member Given two double input values num1 and num2, the program outputs the following values: The initial value of the data member, value The value after adding num1 The value after multiplying by 3 The value after…arrow_forwardThe static data members and static member functions aren't quite what I'm going for in this particular instance.arrow_forward
- In C++, if a member of a class is private, we cannot access it outside the class, but what if the member variable is protected?arrow_forwardSubmit code in C++ CODE with comments and output screenshot is must to get Upvote. Thanksarrow_forwardCreate a User class with the following features (C++): A Private class member fname that is a string A Private class member lname that is a string A setter function setFName(string s) that sets the fname member A setter function setLName(string s) that sets the lname member A getter function getName() that returns the full name with a space between the first and last names Use the following code and save the class in a header file User.hpparrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
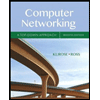
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
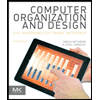
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
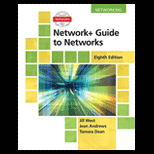
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
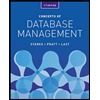
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
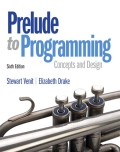
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
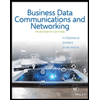
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY