Task #1 Average Class-Create a class called Average according to the UML diagram. Average: -data [ ] :int -mean: double +Average( ): +calculateMean( ): void +toString( ): String +selectionSort( ): void This class will allow a user to enter 5 scores into an array. It will then rearrange the data in descending order and calculate the mean for the data set. Attributes: -data [ ]— the array which will contain the scores -mean — the arithmetic average of the scores Methods: -Average — the constructor. It will allocate memory for the array. Use a for loop to repeatedly display a prompt for the user which should indicate that user should enter score number 1, score number 2, etc. Note: The computer starts counting with 0, but people start counting with 1, and your prompt should account for this. For example, when the user enters score number 1, it will be stored in indexed variable 0. The constructor will then call the selectionSort and the calculateMean methods. calculateMean — this is a method that uses a for loop to access each score in the array and add it to a running total. The total divided by the number of scores (use the length of the array), and the result is stored into the mean. toString — returns a String containing data in descending order and the mean. selectionSort — this method uses the selection sort algorithm to rearrange the data set from highest to lowest. Task #2 Average Driver 1. Create an AverageDriver class. This class only contains the main method. The main method should declare and instantiate an Average object. The Average object information should then be printed to the console. 2. Compile, debug, and run the program. It should output the data set from highest to lowest and the mean. Compare the computer’s output to your hand calculation using a calculator. If they are not the same, do not continue until you correct your code. CODE: /** This program demonstrates the selectionSort method in the ArrayTools class. */ public class SelectionSortDemo { public static void main(String[] arg) { int[] values = {5, 7, 2, 8, 9, 1}; // Display the unsorted array. System.out.println("The unsorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); // Sort the array. selectionSort(values); // Display the sorted array. System.out.println("The sorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); } /** The selectionSort method performs a selection sort on an int array. The array is sorted in ascending order. @param array The array to sort. */ public static void selectionSort(int[] array) { int startScan, index, minIndex, minValue; for (startScan = 0; startScan < (array.length-1); startScan++) { minIndex = startScan; minValue = array[startScan]; for(index = startScan + 1; index < array.length; index++) { if (array[index] < minValue) { minValue = array[index]; minIndex = index; } } array[minIndex] = array[startScan]; array[startScan] = minValue; } } } Upload 2 files: the Average and the AverageDriver files. In addition, copy your output to a text/word file and upload that as well
Task #1 Average Class-Create a class called Average according to the UML diagram.
Average:
-data [ ] :int
-mean: double
+Average( ):
+calculateMean( ): void
+toString( ): String
+selectionSort( ): void
This class will allow a user to enter 5 scores into an array. It will then rearrange the data in descending order and calculate the mean for the data set.
Attributes:
-data [ ]— the array which will contain the scores
-mean — the arithmetic average of the scores
Methods:
-Average — the constructor. It will allocate memory for the array. Use a for loop to repeatedly display a prompt for the user which should indicate that user should enter score number 1, score number 2, etc. Note: The computer starts counting with 0, but people start counting with 1, and your prompt should account for this. For example, when the user enters score number 1, it will be stored in indexed variable 0. The constructor will then call the selectionSort and the calculateMean methods.
calculateMean — this is a method that uses a for loop to access each score in the array and add it to a running total. The total divided by the number of scores (use the length of the array), and the result is stored into the mean.
toString — returns a String containing data in descending order and the mean.
selectionSort — this method uses the selection sort
the data set from highest to lowest.
Task #2 Average Driver
1. Create an AverageDriver class. This class only contains the main method. The main method should declare and instantiate an Average object. The
Average object information should then be printed to the console.
2. Compile, debug, and run the program. It should output the data set from highest to lowest and the mean. Compare the computer’s output to your hand calculation
using a calculator. If they are not the same, do not continue until you correct your code.
CODE:
/** This program demonstrates the selectionSort method in the ArrayTools class.
*/
public class SelectionSortDemo
{
public static void main(String[] arg)
{
int[] values = {5, 7, 2, 8, 9, 1};
// Display the unsorted array.
System.out.println("The unsorted values are:");
for (int i = 0; i < values.length; i++)
System.out.print(values[i] + " ");
System.out.println();
// Sort the array.
selectionSort(values);
// Display the sorted array.
System.out.println("The sorted values are:");
for (int i = 0; i < values.length; i++)
System.out.print(values[i] + " ");
System.out.println();
}
/**
The selectionSort method performs a selection sort on an int array. The array is sorted in ascending order. @param array The array to sort.
*/
public static void selectionSort(int[] array)
{
int startScan, index, minIndex, minValue;
for (startScan = 0; startScan < (array.length-1); startScan++)
{
minIndex = startScan;
minValue = array[startScan];
for(index = startScan + 1; index < array.length; index++)
{
if (array[index] < minValue)
{
minValue = array[index];
minIndex = index;
}
}
array[minIndex] = array[startScan];
array[startScan] = minValue;
}
}
}
Upload 2 files: the Average and the AverageDriver files. In
addition, copy your output to a text/word file and upload that as
well

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

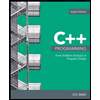
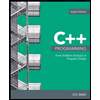