THE BELOW IS IMPORTANT Rewrite the above code so that It has all of the correct access modifiers for good encapsulation. Your code obeys the principle of encapsulation that obliges you to put each method in the proper class. Your code uses NO getters and NO additional classes or methods. You are welcome to modify any existing methods (including signatures and bodies) and/or move them between the existing classes. Your code DOES NOT modify any of the constructors. Important notes: You DO NOT have to write a main meth
THE BELOW IS IMPORTANT Rewrite the above code so that It has all of the correct access modifiers for good encapsulation. Your code obeys the principle of encapsulation that obliges you to put each method in the proper class. Your code uses NO getters and NO additional classes or methods. You are welcome to modify any existing methods (including signatures and bodies) and/or move them between the existing classes. Your code DOES NOT modify any of the constructors. Important notes: You DO NOT have to write a main meth
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
THE BELOW IS IMPORTANT
Rewrite the above code so that
- It has all of the correct access modifiers for good encapsulation.
- Your code obeys the principle of encapsulation that obliges you to put each method in the proper class.
- Your code uses NO getters and NO additional classes or methods. You are welcome to modify any existing methods (including signatures and bodies) and/or move them between the existing classes.
- Your code DOES NOT modify any of the constructors.
Important notes:
- You DO NOT have to write a main method.
- You DO NOT have to write tests.
- You DO NOT have to write Javadocs.
- You DO NOT have to write interfaces to encapsulate data structures.
- There should be no incorrect syntax or other compile-time errors.
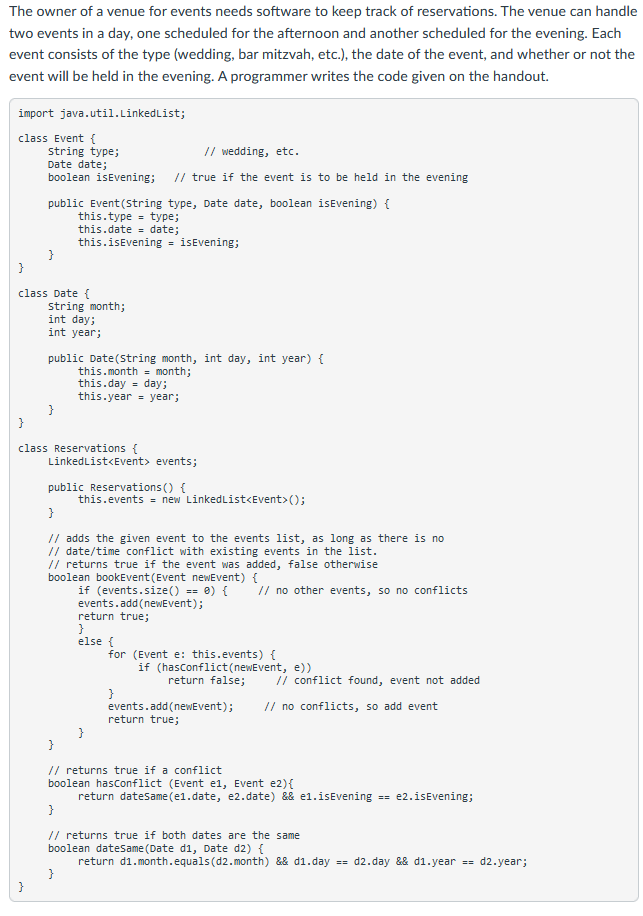
Transcribed Image Text:The owner of a venue for events needs software to keep track of reservations. The venue can handle
two events in a day, one scheduled for the afternoon and another scheduled for the evening. Each
event consists of the type (wedding, bar mitzvah, etc.), the date of the event, and whether or not the
event will be held in the evening. A programmer writes the code given on the handout.
import java.util.LinkedList;
class Event {
// wedding, etc.
String type;
Date date;
boolean isEvening;
// true if the event is to be held in the evening
public Event(string type, Date date, boolean isEvening) {
this.type - type;
this.date = date;
this.isEvening = isEvening;
}
class Date {
string month;
int day;
int year;
public Date(string month, int day, int year) {
this.month = month;
this.day = day;
this.year = year;
class Reservations {
LinkedList<Event> events;
public Reservations() {
this.events = new LinkedList<Event>();
// adds the given event to the events list, as long as there is no
// date/time conflict with existing events in the list.
// returns true if the event was added, false otherwise
boolean bookEvent (Event newEvent) {
if (events.size() == 0) {
events.add(newEvent);
return true;
}
else {
// no other events, so no conflicts
for (Event e: this.events) {
if (hasconflict(newEvent, e))
return false;
/i conflict found, event not added
}
events.add(newEvent);
return true;
// no conflicts, so add event
// returns true if a conflict
boolean hasconflict (Event e1, Event e2){
return datesame(e1.date, e2.date) && e1.isEvening = e2.isEvening;
}
// returns true if both dates are the same
boolean datesame (Date di, Date d2) {
return d1.month.equals (d2. month) && d1.day == d2.day && d1.year == d2.year;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
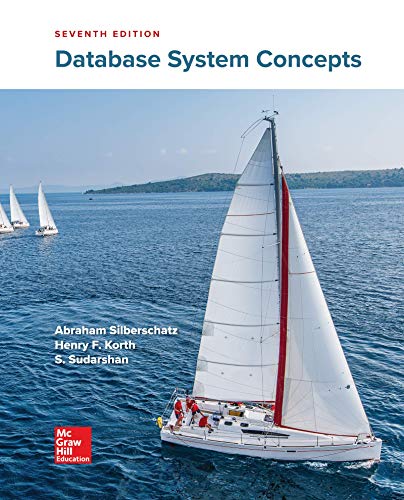
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
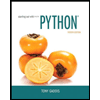
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
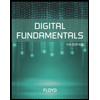
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
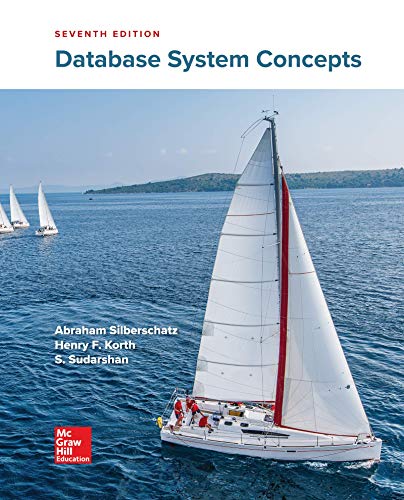
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
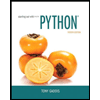
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
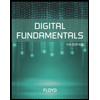
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
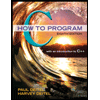
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
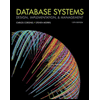
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
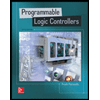
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education