The class will be called "Item" and will represent an item in the game that can be carried and used by a player (such as a weapon or a tool). The Item Class The Item class will have only one property: A string description. The Item class will have exactly 3 methods: There will be a default constructor that takes no parameters. This constructor will give a generic, default description of the Item. There will be a constructor that takes a string parameter, and it will initialize the Item's description based on the parameter. There will be a method called "print" that will print the Item's description to standard output.
You will create a couple of classes that could be used to create a Text Adventure game.
The class will be called "Item" and will represent an item in the game that can be carried and used by a player (such as a weapon or a tool).
The Item Class
The Item class will have only one property:
- A string description.
The Item class will have exactly 3 methods:
- There will be a default constructor that takes no parameters. This constructor will give a generic, default description of the Item.
- There will be a constructor that takes a string parameter, and it will initialize the Item's description based on the parameter.
- There will be a method called "print" that will print the Item's description to standard output.
PLEASE MAKE SURE ITS POSSIBLE TO COMPILE WITH THIS CODE. THANKS.
#include "Item.h"
#include <iostream>
#include <string> using namespace std;
int main()
{
Item sword("Sword of Destiny");
Item potion("Healing Potion");
Item key("Key of Wisdom");
string name;
cout << "Enter your name: ";
getline(cin, name);
Player user(name);
user.print();
cout << "USER PICKS UP SWORD:" << endl;
user.pickUpItem(sword);
user.print();
cout << "USER MOVES NORTH:" << endl;
user.move(NORTH);
user.print();
cout << "USER PICKS UP POTION:" << endl;
user.pickUpItem(potion);
user.print();
cout << "USER PUTS DOWN ITEM:" << endl;
user.putDownItem();
user.print();
cout << "USER MOVES WEST:" << endl;
user.move(WEST);
user.print();
cout << "USER PICKS UP KEY:" << endl;
user.pickUpItem(key);
user.print();
}

Step by step
Solved in 4 steps with 1 images

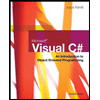
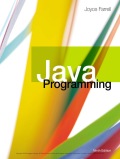
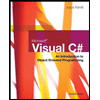
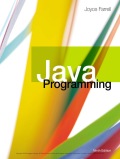