The code generating the user-app interaction we saw in class yesterday is included below. For our next class, write a UML class diagram for one candidate class to turn this program Into an OO design. Also, modify the program below to teach a user OO concepts using twenty flashcards based on material
The code generating the user-app interaction we saw in class yesterday is included below.
For our next class, write a UML class diagram for one candidate class to turn this program
Into an OO design. Also, modify the program below to teach a user OO concepts using twenty
flashcards based on material included in the two attached files.
You can use C-type strings to implement your modifications. Your program must randomly show
the front or the back of a presented card and randomly present once each card in the set before
letting the user repeat the whole set of cards.
Submit a hard copy of your programs before class and take your computer to the classroom to demo
the flashcards program.
// Exercise 3.38 Solution
// Randomly generate numbers between 1 and 1000 for user to guess.
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
void guessGame(); // function prototype
bool isCorrect( int, int ); // function prototype
int main()
{
// srand( time( 0 ) ); // seed random number generator
guessGame();
return 0; // indicate successful termination
} // end main
// guessGame generates numbers between 1 and 1000
// and checks user's guess
void guessGame()
{
int answer; // randomly generated number
int guess; // user's guess
char response; // 'y' or 'n' response to continue game
// loop until user types 'n' to quit game
do {
// generate random number between 1 and 1000
// 1 is shift, 1000 is scaling factor
answer = 1 + rand() % 1000;
// prompt for guess
cout << "I have a number between 1 and 1000.\n"
<< "Can you guess my number?\n"
<< "Please type your first guess." << endl << "? ";
cin >> guess;
// loop until correct number
while ( !isCorrect( guess, answer ) )
cin >> guess;
// prompt for another game
cout << "\nExcellent! You guessed the number!\n"
<< "Would you like to play again (y or n)? ";
cin >> response;
cout << endl;
} while ( response == 'y' );
} // end function guessGame
// isCorrect returns true if g equals a
// if g does not equal a, displays hint
bool isCorrect( int g, int a )
{
// guess is correct
if ( g == a )
return true;
// guess is incorrect; display hint
if ( g < a )
cout << "Too low. Try again.\n? ";
else
cout << "Too high. Try again.\n? ";
return false;
} // end function isCorrect

Step by step
Solved in 2 steps

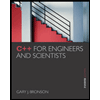
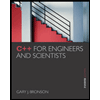