The code is already given above. Problem: Using PYTHON, create and implement a recursive function that will do the following: Input #1 Output #1 10 Enter n: 10 [2, 3, 5, 7] Input #2 Output #2 Enter n: 50 50 [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47]
Given code (copy-paste):
Problem (see pic):
def createList(n):
#Base Case/s
#ToDo: Add conditions here for base case/s
#if <condition> :
#return <value>
#Recursive Case/s
#ToDo: Add conditions here for your recursive case/s
#else:
#return <operation and recursive call>
#remove the line after this once all ToDo is completed
return []
def removeMultiples(x, arr):
#Base Case/s
#TODO: Add conditions here for your base case/s
#if <condition> :
#return <value>
#Recursive Case/s
#TODO: Add conditions here for your recursive case/s
#else:
#return <operation and recursive call>
#remove the line after this once you've completed all ToDo
return []
def Sieve_of_Eratosthenes(list):
#Base Case/s
if len(list) < 1 :
return list
#Recursive Case/s
else:
return [list[0]] + Sieve_of_Eratosthenes(removeMultiples(list[0], list[1:]))
if __name__ == "__main__":
n = int(input("Enter n: "))
print(n)
list = createList(n)
#Solution 1
primes = Sieve_of_Eratosthenes(list)
print(primes)
![The code is already given above.
Problem: Using PYTHON, create and implement a recursive function that will do the following:
Input #1
Output #1
10
Enter n: 10
[2, 3, 5, 7]
Input #2
Output #2
Enter n: 50
50
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47]
V The input is a single integer value. This value will be the upper bound of the list of values that will be created using
the createList() function.
V The input is an integer from the range [2,200] (inclusive). Assume that the input is always valid.
Output:
V The output will be a list containing primes within the range of 2 to n (inclusive), where n is the value of the user's input.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e9f5a4e-733b-4ef8-89d9-ff335bcd5624%2Fb9d5f7ca-baae-4ead-a244-d8185374da25%2F1v3dp1p_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 4 images

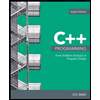
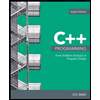