Create an HLA Assembly language program that prompts a number from the user. Create and call a function that calculates the sum of all the numbers from 1 up to the number entered. So for example, a value of 10 would lead to the sum: 1+2+3+4+5+6+7+8+9+10 = 55 In order to receive full credit, you must not use recursion to solve this problem. Instead, you should build an iterative (that is, looping or branching…) function. In order to receive full credit, you must define a function, preserve registers, pass arguments on the run-time stack and return the answer in the register AH. To solve this problem, define a function whose signature is: procedure summing( value : int8 ); @nodisplay; @noframe; Here are some example program dialogues to guide your efforts: Provide a number: 10 summing(10) equals 55! Provide a letter: 5 summing(5) equals 15!
Create an HLA Assembly language
In order to receive full credit, you must not use recursion to solve this problem. Instead, you should build an iterative (that is, looping or branching…) function. In order to receive full credit, you must define a function, preserve registers, pass arguments on the run-time stack and return the answer in the register AH. To solve this problem, define a function whose signature is:
procedure summing( value : int8 ); @nodisplay; @noframe;
Here are some example program dialogues to guide your efforts:
Provide a number: 10
summing(10) equals 55!
Provide a letter: 5
summing(5) equals 15!

Step by step
Solved in 2 steps

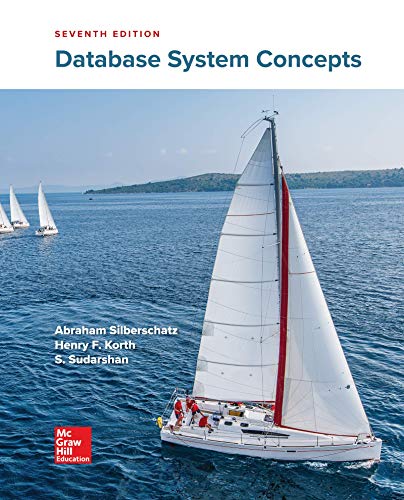
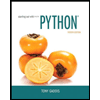
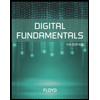
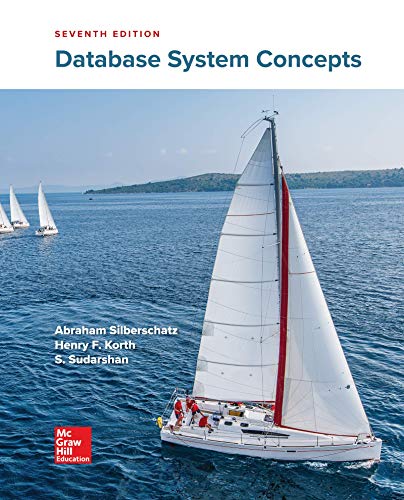
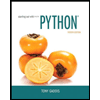
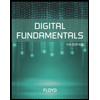
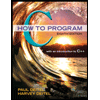
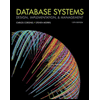
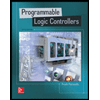