The first function you will write and test is the clean_data function. The function is described in the starter code in a function header comment above the function. Here is the function: def clean_data(list, x): #TODO: write your code here pass Take a look at the first few lines of the data file: A 83 140 228 286 426 612 486 577 836 0 0 Aaliyah 0 0 0 0 0 0 0 0 0 380 215 Aaron 193 208 218 274 279 232 132 36 32 31 41 Each line is a baby name followed by how popular the name was in 11 different years. Each number is a ranking, so Aaron was 193th most popular name in 1900 and 41st most popular name in 2000. The lower the number, the more popular the name. However, if the name was not popular enough to make the list in a particular year, a zero was recorded. If we plot the data as is, a name that was so unpopular that it didn’t make the list (ranked 0) will appear most popular (since lower means more popular). Therefore, we need to clean up the data a bit in order for it to make sense. The function clean_data has two parameters. The first parameter is a list (e.g. a list of rankings by year for a particular baby name). The second parameter is a value x. The function will do in-place modification of the parameter list and replace all zeros with the value x. You will write this function generally. Here are three of the cases you should test: # test 1 # before data is cleaned list1 = [0, 4, 3, 2, 0, 6, 7, 8, 3, 0, 7, 7, 8, 3, 0] x1 = 5 # after data is cleaned list1 = [5, 4, 3, 2, 5, 6, 7, 8, 3, 5, 7, 7, 8, 3, 5] # test 2 # before data is cleaned list2 = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] x2 = 10001 # after data is cleaned list2 = [10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001]
The first function you will write and test is the clean_data function. The function is described in the starter code in a function header comment above the function. Here is the function: def clean_data(list, x): #TODO: write your code here pass Take a look at the first few lines of the data file: A 83 140 228 286 426 612 486 577 836 0 0 Aaliyah 0 0 0 0 0 0 0 0 0 380 215 Aaron 193 208 218 274 279 232 132 36 32 31 41 Each line is a baby name followed by how popular the name was in 11 different years. Each number is a ranking, so Aaron was 193th most popular name in 1900 and 41st most popular name in 2000. The lower the number, the more popular the name. However, if the name was not popular enough to make the list in a particular year, a zero was recorded. If we plot the data as is, a name that was so unpopular that it didn’t make the list (ranked 0) will appear most popular (since lower means more popular). Therefore, we need to clean up the data a bit in order for it to make sense. The function clean_data has two parameters. The first parameter is a list (e.g. a list of rankings by year for a particular baby name). The second parameter is a value x. The function will do in-place modification of the parameter list and replace all zeros with the value x. You will write this function generally. Here are three of the cases you should test: # test 1 # before data is cleaned list1 = [0, 4, 3, 2, 0, 6, 7, 8, 3, 0, 7, 7, 8, 3, 0] x1 = 5 # after data is cleaned list1 = [5, 4, 3, 2, 5, 6, 7, 8, 3, 5, 7, 7, 8, 3, 5] # test 2 # before data is cleaned list2 = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] x2 = 10001 # after data is cleaned list2 = [10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001]
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 3E
Related questions
Question
The first function you will write and test is the clean_data function. The function is described in the starter code in a function header comment above the function. Here is the function:
def clean_data(list, x):
#TODO: write your code here
pass
Take a look at the first few lines of the data file:
A 83 140 228 286 426 612 486 577 836 0 0
Aaliyah 0 0 0 0 0 0 0 0 0 380 215
Aaron 193 208 218 274 279 232 132 36 32 31 41
Each line is a baby name followed by how popular the name was in 11 different years. Each number is a ranking, so Aaron was 193th most popular name in 1900 and 41st most popular name in 2000. The lower the number, the more popular the name. However, if the name was not popular enough to make the list in a particular year, a zero was recorded. If we plot the data as is, a name that was so unpopular that it didn’t make the list (ranked 0) will appear most popular (since lower means more popular). Therefore, we need to clean up the data a bit in order for it to make sense.
The function clean_data has two parameters. The first parameter is a list (e.g. a list of rankings by year for a particular baby name). The second parameter is a value x. The function will do in-place modification of the parameter list and replace all zeros with the value x.
You will write this function generally. Here are three of the cases you should test:
# test 1
# before data is cleaned
list1 = [0, 4, 3, 2, 0, 6, 7, 8, 3, 0, 7, 7, 8, 3, 0]
x1 = 5
# after data is cleaned
list1 = [5, 4, 3, 2, 5, 6, 7, 8, 3, 5, 7, 7, 8, 3, 5]
# test 2
# before data is cleaned
list2 = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
x2 = 10001
# after data is cleaned
list2 = [10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001]
# test 3
# before data is cleaned
list3 = [857, 797, 972, 883, 0, 0, 0, 0, 0, 0, 0]
x3 = 1113
# after data is cleaned
list3 = [857, 797, 972, 883, 1113, 1113, 1113, 1113, 1113, 1113, 1113]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
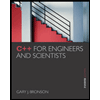
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
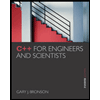
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr