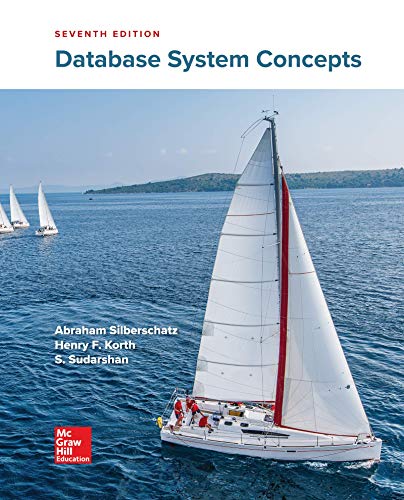
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
program7.py
This assignment requires the main function and a custom value-returning function. The value-returning function takes a list of random integers as its only argument and returns a smaller list of only the elements that end with 7. This value-returning function must use a list comprehension to create this smaller list.
In the main function, code these steps in this sequence:
- Set random seed to 42 import random
random.seed(42) - create an empty list that will the hold random integers.
- use a loop to add 50 random integers to the list. All integers should be between 200 and 250, inclusive. Duplicates are okay.
- sort the list in ascending order and then use another loop to display all 50 sorted integers on one line separated by spaces.
- print a slice showing list elements indexed 5 through 10, inclusive.
- print a second slice showing the final 5 elements in the sorted list.
- execute the custom function with the entire original list as its sole argument.
- report the number of elements in the new "sevens" list returned by the custom function.
- if 207 is not in the "sevens" list, add it to the start of "sevens" and report that this happened. Otherwise, report that nothing was added.
- if 247 is in the "sevens" list, report the index of its first occurrence. Otherwise, append 247 to the end of the list.
- use another loop to display all elements in "sevens" on one line separated by spaces.
- Finally, report the total of the "sevens" list.
Sample Output
![Here is the complete list of 50 elements, sorted from low to high:
200, 200, 201, 203, 203, 203, 204, 205, 207, 209, 210, 210, 211, 212, 213, 213, 216, 217, 218, 218, 219, 220, 221, 221, 222, 224, 224, 225, 225, 226, 228, 229, 231, 234, 234, 237, 241, 244, 245, 246, 246, 247, 247, 248, 248, 250
Elements indexed 5 to 10 in the sorted list are [203, 203, 204, 205, 207, 209]
The final 5 elements in the sorted list are [247, 247, 248, 248, 250]
Number of elements ending with 7: 5
207 was already in the "sevens" list, so nothing was added.
Found 247 at index 3 in the "sevens" list, so nothing was added.
Here are the elements in the "sevens" list:
207, 217, 237, 247, 247
The total of all elements in the "sevens" list is 1155.](https://content.bartleby.com/qna-images/question/93a2e007-7c0b-402a-9935-252f03fd635c/79f8bbb2-2505-44ef-8c07-61fbccdbb417/lhfkpte_thumbnail.png)
Transcribed Image Text:Here is the complete list of 50 elements, sorted from low to high:
200, 200, 201, 203, 203, 203, 204, 205, 207, 209, 210, 210, 211, 212, 213, 213, 216, 217, 218, 218, 219, 220, 221, 221, 222, 224, 224, 225, 225, 226, 228, 229, 231, 234, 234, 237, 241, 244, 245, 246, 246, 247, 247, 248, 248, 250
Elements indexed 5 to 10 in the sorted list are [203, 203, 204, 205, 207, 209]
The final 5 elements in the sorted list are [247, 247, 248, 248, 250]
Number of elements ending with 7: 5
207 was already in the "sevens" list, so nothing was added.
Found 247 at index 3 in the "sevens" list, so nothing was added.
Here are the elements in the "sevens" list:
207, 217, 237, 247, 247
The total of all elements in the "sevens" list is 1155.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forwardQuestion 15 kk .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forwardHAPTER 5: Lists and Dictiona st membership > 51621 O WorkArea Instructions eadline: 03/ 11:59pm EDT Given that k contains an integer and that play_list has been defined to be a list, write a expression that evaluates to True if the value assigned to k is an element of play_list. Additional Notes: play_list and k should not be modifiedarrow_forward
- Create a list L containing at least 10 values ranging from j to k. Using a for loop, code a function that will compute and display the following list: L_out[0] = L[0] L_out[i] = L[i] + L[i-1] if i is odd and i is not equal to 0 L_out[i] = L[i] * 3 if i is even and i is not equal to 0 where L[i] represents the value stored in L at the position i. Example: L ‘(2 3 4 5) L_out ‘(2 5 12 9)arrow_forwardb. Write a function cut12 that removes the last two items from a list given as its argument. The existing list should be changed in-place; the function should not return anything,arrow_forwardQ1: Assignment Difficulty Dan has a list of problems suitable for Assignment 4. The difficulties of these problems are stored in a list of integers a. The i-th problem’s difficulty is represented by a[i] (the higher the integer, the more difficult the problem). Dan is too busy eating saltines to worry about Assignment 4 decisions, so he asks Michael the TA to select at least two problems from the list for the assignment. Since there are many possible subsets of the problems to consider and Michael has a life, he decides to consider only sublists (definition follows) of the list of problems. To make grading the assignment easier, Michael wants to pick problems that don’t vary too much in difficulty. What is the smallest difference between the difficulties of the most difficult selected problem and the least difficult selected problem he can achieve by selecting a sublist of length at least 2 of the original list of problems? Definition: A sublist of a list a is any list you can obtain…arrow_forward
- Complete the reverse_list() function that returns a new string list containing all contents in the parameter but in reverse order. Ex: If the input list is: ['a', 'b', 'c'] then the returned list will be: ['c', 'b', 'a'] Note: Use a for loop. DO NOT use reverse() or reversed(). This is my code so far: def reverse_list(letters): for x in (letters): letters.sort(reverse=True) return lettersif __name__ == '__main__': ch = ['a', 'b', 'c'] print(reverse_list(ch)) # Should print ['c', 'b', 'a'] When submitting I get 4/10 and it says: Test input list ['a', 'b', 'c'] returns ['c', 'b', 'a'] Your output reverse_list() function reversed array incorrectly: ['c', 'b', 'a'] reverse_list() function should have returned: ['a', 'b', 'c'] Test input list ['a', 'b', 'c', 'd'], first element of returned list is ['d'] Your output First character of returned array should be a instead of d Test empty input array TypeError: object of type 'NoneType' has no len()arrow_forward] ] get_nhbr In the cell below, you are to write a function called "get_nhbr(Ist, graph)" that takes in two inputs: a list of vertices and a graph. The function is to return a list that contains the neighborhood of the vertices in 'Ist' (remember that this means you are finding the union of the individual vertices' neighborhoods). + Code + Markdown After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (get_nhbr(["A", "D"], {"A" : ["B"], "B" : ["A", "D", "E"], "C" : ["E"], "D":["B"], "E":["B","C","F"], "F":["E"]}), get_nhbr(["B", "C", "F"], {"A" : ["B"], "B" : ["A", "D", "E"], "C" : ["E"], "D":["B"], "E":["B","C","F"], "F":["E"]})) This should return ["B"] ["A", "D", "E"] Python Pythonarrow_forwardOutput values in a list below a user defined amount - functions Write a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value. Ex: If the input is: 5 50 60 140 200 75 100 the output is: 50 60 75 The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. Such functionality is common on sites like Amazon, where a user can filter results. Your code must define and call the following two functions:def get_user_values()def output_ints_less_than_or_equal_to_threshold(user_values, upper_threshold) Utilizing functions will help to make your main very clean and intuitive. Note: This is a lab from a previous chapter that now requires the use of functions. My…arrow_forward
- program7.pyThis assignment requires the main function and a custom value-returning function. The value-returning function takes a list of random Fahrenheit temperatures as its only argument and returns a smaller list of only the temperatures above freezing. This value-returning function must use a list comprehension to create the list of above freezing temperatures.In the main function, code these steps in this sequence: create an empty list that will the hold random integer temperatures. use a loop to add 24 random temperatures to the list. All temperatures should be between 10 and 55, inclusive. Duplicates are okay. sort the list in ascending order. use another loop to display all 24 sorted temperatures on one line separated by spaces. 32 could be in the list. If it is, report the index of the first instance of 32. if 32 isn't in the list, add it at index 4. assign a slice consisting of the first six temperatures to a new list. Then print it. execute the custom value-returning…arrow_forwardPython Help Write a function equals(a,b) that returns true when the two lists a, and b have the same elements in the same order, and false otherwise.Write main() to call the function equals(a,b)arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
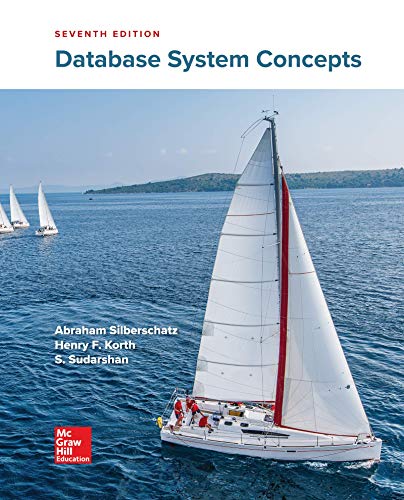
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
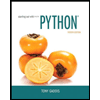
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
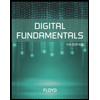
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
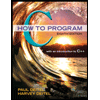
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
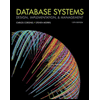
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
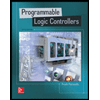
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education