THE PROGRAM SHOULD BE WRITTEN USING C++ Checkpoints Absolutely no arrays or structures. The problem is designed to be solved using primitive types only: int, float, double, char. Use only pass by value. Also, ABSOLUTELY NO GLOBAL VARIABLE DECLARATIONS. All variables need to be declared in main() or another function. No loops for this lab. 1) Include name, e-mail, and lab# as comment and print same to output 2) Minimum of three (3) comments in each function (Purpose: Pre: Post:) For how to write Pre/Post/Purpose watch the video and follow the model code posted on week 5 of Canvas: Code from Webinar - Training Heart Rate (Watch Video First). 3) Use a globally defined constant for the allotment per person, i.e. 50 gallons 4) Input may not be case sensitive. That is, if user enters ‘s’ change it to ‘S’ immediately. To do this use if() and assign uppercase value or you may use toupper() function (see 10.2 of text). Only an error message should be output and nothing more if data is erroneous. Use exit() function as explained in seciotn 6.15 of text 5) One function which must be called from main() to calculate the total budget. Use C++ Style with prototype before main() and the function definition after main(). 6) One function which must be called from main() to calculate the cost based on budget (calculated in previous function) and CCFs used. Use C++ Style with prototype before main() and the function definition after main(). 7) One function which must be called from main() to be used for all the output. Output must be formatted using 2 decimal places for all values and a dollar sign for the amount as shown in examples.
- THE
PROGRAM SHOULD BE WRITTEN USING C++ - Checkpoints Absolutely no arrays or structures. The problem is designed to be solved using primitive types only: int, float, double, char. Use only pass by value. Also, ABSOLUTELY NO GLOBAL VARIABLE DECLARATIONS. All variables need to be declared in main() or another function. No loops for this lab.
1) Include name, e-mail, and lab# as comment and print same to output
2) Minimum of three (3) comments in each function (Purpose: Pre: Post:) For how to write Pre/Post/Purpose watch the video and follow the model code posted on week 5 of Canvas: Code from Webinar - Training Heart Rate (Watch Video First).
3) Use a globally defined constant for the allotment per person, i.e. 50 gallons
4) Input may not be case sensitive. That is, if user enters ‘s’ change it to ‘S’ immediately. To do this use if() and assign uppercase value or you may use toupper() function (see 10.2 of text). Only an error message should be output and nothing more if data is erroneous. Use exit() function as explained in seciotn 6.15 of text
5) One function which must be called from main() to calculate the total budget. Use C++ Style with prototype before main() and the function definition after main().
6) One function which must be called from main() to calculate the cost based on budget (calculated in previous function) and CCFs used. Use C++ Style with prototype before main() and the function definition after main().
7) One function which must be called from main() to be used for all the output. Output must be formatted using 2 decimal places for all values and a dollar sign for the amount as shown in examples.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

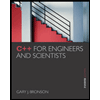
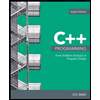
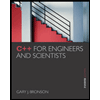
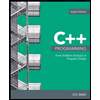