the program that will allow user searc should see the list of items currently a should see the window for the rearch
the program that will allow user searc should see the list of items currently a should see the window for the rearch
Chapter6: Using Arrays
Section: Chapter Questions
Problem 10E
Related questions
Question
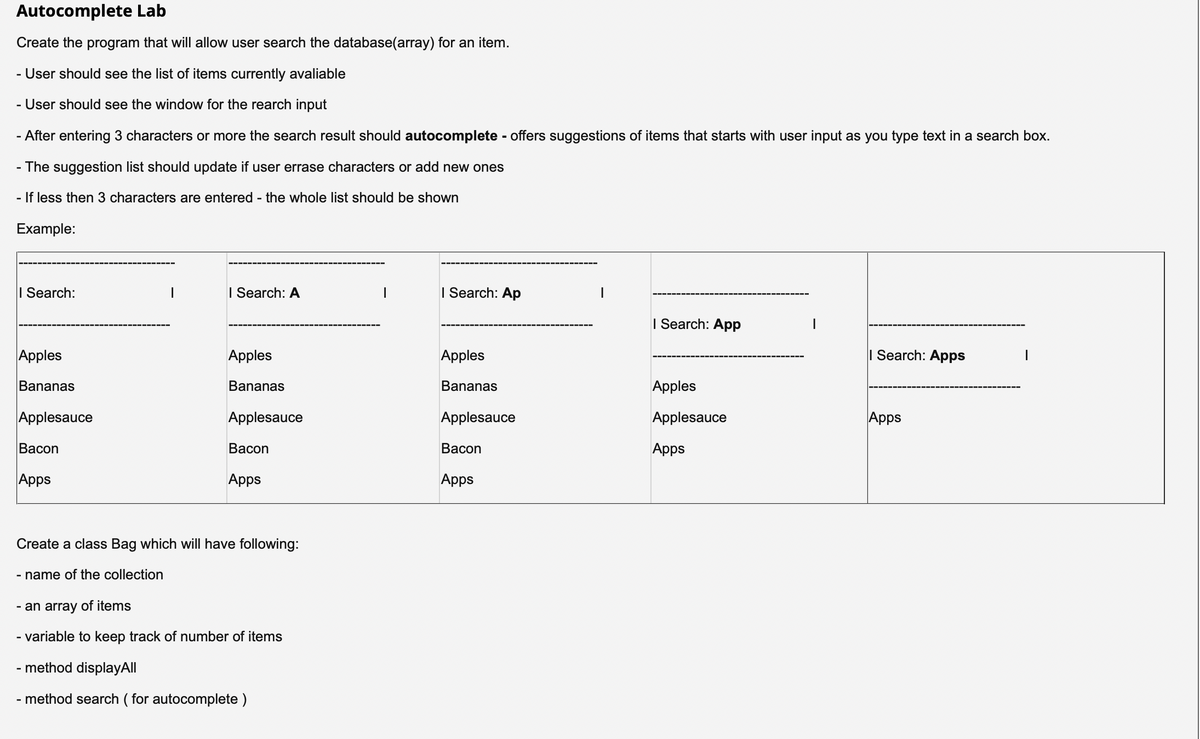
Transcribed Image Text:Autocomplete Lab
Create the program that will allow user search the database(array) for an item.
- User should see the list of items currently avaliable
- User should see the window for the rearch input
- After entering 3 characters or more the search result should autocomplete - offers suggestions of items that starts with user input as you type text in a search box.
- The suggestion list should update if user errase characters or add new ones
- If less then 3 characters are entered - the whole list should be shown
Example:
I Search:
I Search: A
I Search: Ap
I Search: App
Apples
Apples
Apples
I Search: Apps
Bananas
Bananas
Bananas
Apples
Applesauce
Applesauce
Applesauce
Applesauce
Apps
Ваcon
Ваcon
Ваcon
Apps
Apps
Apps
Аpps
Create a class Bag which will have following:
- name of the collection
- an array of items
- variable to keep track of number of items
- method displayAll
- method search ( for autocomplete )
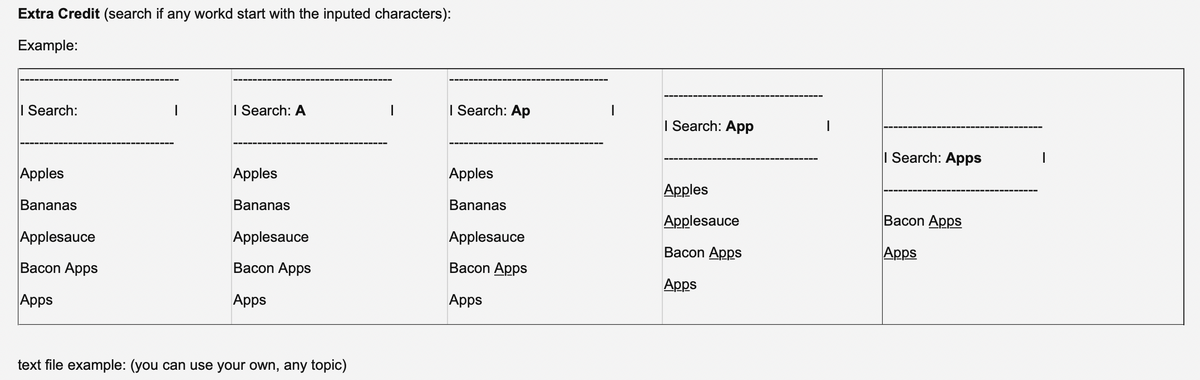
Transcribed Image Text:Extra Credit (search if any workd start with the inputed characters):
Example:
I Search:
I Search: A
I Search: Ap
I Search: App
Search: Apps
Apples
Apples
Apples
Аpples
Bananas
Bananas
Bananas
Applesauce
Bacon Apps
Applesauce
Applesauce
Applesauce
Bacon Apps
Аpps
Bacon Apps
Bacon Apps
Ваcon Apps
Apps
Apps
Apps
Apps
text file example: (you can use your own, any topic)
Expert Solution

Step 1
Language: C++/ CPP
Complete Source Code with proper indentation and line by line comments has been provided for better understanding.
Source Code:
--Start of Code:
/* * Create the program that will allow user search the detabaselarray) for an item.
*User should soe the list of tems currently avaliable
*User should see the window for the rearch input
*After entening 3 characters or more the search result shoukd autocomplete - offers suggestions of items that starts with user input as you type text in a search box
*The suggostion list should update if user errase characters or add new ones
*If less then 3 characters are entered -the whole list should be shown
EXAMPLE:
-----------------------------
| Search: A |
-----------------------------
| Apple |
| Banana |
| Avocado |
| Applesauce |
| Apps |
-----------------------------
| Search: App |
-----------------------------
| Apple |
| Applesauce |
| Apps |
-----------------------------
| Search: Apps |
-----------------------------
| Apps |
Create a class Bag which: has name of collection an array of items variable to keep track of number of items in the bag method displayAll method Search (for Autocomplete)
*/
#include <iostream>
// to use cout and cin
#include <string> // to use string
#include <vector> // to use vector
#include <algorithm> // to use sort
using namespace std; // to use cout and cin as std::cout and std::cin
class Bag
{
// class Bag to store all the items in the bag private:
// private variables string name;
// name of the bag vector<string> items;
// vector of items int numberOfItems; // number of items in the bag //Publice functions public: Bag(string name) //constructor { this->name = name; // set the name of the bag } // end of constructor void addItem(string item)
//add the item to the bag
{ items.push_back(item);
// add the item to the vector
}
// end of addItem
void displayAll()
// Displays all the elements in the bag
{
cout << "Bag: " << name << endl;
// display the name of the bag
for (int i = 0; i < items.size(); i++)
// loop through the vector
{
cout << items[i] << endl;
// display the item
}
// end of for loop
}
// end of displayAll
void search()
{
// search for the auto complete
/* * Autocomplete */ string search;
// string to store the search
cout<<"-----------------------------"<<endl;
// display the search box cout<<"| Search: ";
// display the search box cin>>search;
// get the search
cout<<"-----------------------------"<<endl;
// display the search box
vector<string>::iterator it;
// iterator to loop through the
vector it = find_if(items.begin(), items.end(),
[search](string item)
{
// find the item return item.find(search) == 0;
// return the item that starts with the search });
// end of find_if
//if length of search is less than 3 - show all items if (search.length() < 3)
{
displayAll();
// display all items
}
else
//if length of searching item is more than 3
{
//if search is found - show autocomplete
if (it != items.end())
{
//Check if search matches the substring of the item
if (search.length() == (*it).length())
{
cout << *it << endl;
// display the found item
}
else
//if the search dont matches
{
//if search matches the substring of the item - show autocomplete cout << *it << endl;
//search for the rest of the items it++;
// increment the iterator
while (it != items.end())
//loop through the vector of other elements
{
if ((*it).find(search) == 0)
// if the element starts with the search
{ cout << *it << endl;
// display the element
}
// end of
if it++;
// increment the iterator
}
// end of while loop
}
// end of else
}
//end of if else
// nothing found
{
cout << "No items found" << endl;
// display no items found
}
// end of else
}
// end of else
}
// end of search
};
// end of class Bag
int main()
//Main Driver code
{
Bag bag("Fruits");
// create a bag with name of fruits
bag.addItem("Apple");
// add an item to the bag
bag.addItem("Banana"); // add an item to the bag bag.addItem("Avocado"); // add an item to the bag bag.addItem("Applesauce"); // add an item to the bag bag.addItem("Apps"); // add an item to the bag
bag.displayAll(); // display all the items in the
bag do{
// do while loop to run infinitely bag.search();
// search for the item }while(true);
// end of do while loop return 0;
// end of main }
--End of Code
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
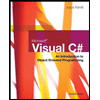
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
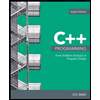
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
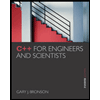
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
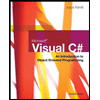
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
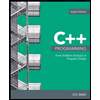
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
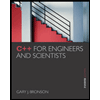
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
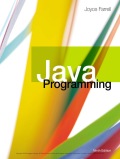
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
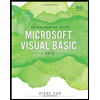
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage