THE QUESTION IS BELOW WITH OUTPUT AND INPUT. AND I HAVE PROVIDED MY ANSWER AND COMPILER ERROR AT THE END OF THE QUESTION. SO YOU HAVE TO SOLVE MY COMPILER ERROR. AND CORRECT MY CODE .
THE QUESTION IS BELOW WITH OUTPUT AND INPUT.
AND I HAVE PROVIDED MY ANSWER AND COMPILER ERROR AT THE END OF THE QUESTION.
SO YOU HAVE TO SOLVE MY COMPILER ERROR. AND CORRECT MY CODE .
------ QUESTION STARTS --------
Write a C++ program to collect blood donor details like name, age, height, weight, gender, blood group. The data like weight, height, age are not fixed and it may change so they wanted to update all these details in their
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. Create separate classes in separate files.
The class called Donor contains the following private attributes.
Data Type | Variable Name |
string | name |
int | age |
float | height |
float | weight |
string | gender |
string | bloodGroup |
Important:- Define the member variables and include appropriate getters and setters for the above class.
Include the following member function in the Donor class
Member Function | Description |
void display() | To display the donor details. |
In the main() method, read the number of donors from the user. Then create an array of ‘n’ donor objects and read the ‘n’ donor details from the user in the console. Display the ‘n’ donor details by calling the display() method present in the Donor class.
Input and Output Format:
Display the height with one decimal point.
Please refer to the sample input and output for formatting specifications.
[All text in bold are input and the remaining are output]
Sample Input and Output :
Enter the number of donors:
3
Enter the donor details 1
Enter the Name :
rami
Enter the Age :
22
Enter the height :
156.5
Enter the weight :
47
Enter the Gender :
F
Enter the Blood Group :
O+
Enter the donor details 2
Enter the Name :
karnu
Enter the Age :
25
Enter the height :
167
Enter the weight :
57
Enter the Gender :
F
Enter the Blood Group :
B-
Enter the donor details 3
Enter the Name :
bhola
Enter the Age :
56
Enter the height :
155.8
Enter the weight :
67.8
Enter the Gender :
M
Enter the Blood Group :
AB-
Name :sowmi
Age :22
Height :156.5
Weight :47
Gender :F
Blood Group :O+
Name :shanu
Age :25
Height :167
Weight :57
Gender :F
Blood Group :B-
Name :siva
Age :56
Height :155.8
Weight :67.8
Gender :M
Blood Group :AB-
---------------QUESTIONS ENDS----------------
---------------------MY ANSWER BELOW-------------
MAIN.CPP
#include <iostream> #include "Donor.cpp" using namespace std; int main()
{ int n;
cout << "\n\n"; cout << "Enter the number of donors: "; cin >> n; Donor dr[n]; for(int i=0; i<n; i++) { cout << "Enter the donor details "<<i+1<<endl; cout << "Enter the Name: " << endl; cin >> dr[i].name; cout << "Enter the Age: " << endl;
cin >> dr[i].age;
cout << "Enter the height: " << endl;
cin >> dr[i].height;
cout << "Enter the weight: " << endl;
cin >> dr[i].weight;
cout << "Enter the Gender: " << endl;
cin >> dr[i].gender;
cout << "Enter the Blood Group: " << endl;
cin >> dr[i].group; } for (int i = 0; i < n; i++) dr[i].display();
cout << "\n\n";
return 0; } |
DONOR.CPP
#include<iostream> using namespace std;
class Donor{ public: string name, gender, group; int age; float height; float weight;
public: void setName(string s) { this -> name=s; } void setAge(int a) { this -> age = a; } void setHeight(float a) { this->height = a; } void setWeight(float a) { this->weight = a; } void setgender(string a) { this->gender = a; } void setgroup(string a) { this->group = a; } string getName() { return this -> name; }
int getAge() { return this->age; } float getHeight() { return this->height; } float getWeight() { return this->weight; } string getgender() { return this->gender; } string getgroup() { return this->group; } void display(){ cout << "\n"; cout << "Name: " <<getName() << endl; cout << "Age: " <<getAge() << endl; cout << "Height: " << getHeight() << endl; cout << "Weight: " << getWeight() << endl; cout << "Gender: " << getgender() << endl; cout << "Blood Group: " << getgroup() << endl; cout << "\n\n"<<endl; } }; |
----------ANSWER ENDS-------------------------
-----------------COMPILER ERROR PLEASE SEE ATTACHED IMAGE----------------
![Observations:
Compilation Errors Main.cpp: In function 'int main()': Main.cpp:94:8: error: 'n' was not declared in this scope cin>>n; ^Main.cpp:106:10:
error: 'obs' was not declared in this scope cin>>obs[i].name; ^w- Main.cpp:106:10: note: suggested alternative: 'abs' cin>>obs[i].name; ^~~
abs Main.cpp:119:10: error:'class Donor' has no member named 'setGender; did you mean 'setgender? obj1.setGender(obs[i).gender);
~~-- setgender Main.cpp:122:10: error:'class Donor'has no member named 'setBloodGroup'; did you mean 'setgroup?
obj1.setBloodGroup(obs[i).bloodGroup); ^~~~~~~~~~~~ setgroup Main.cpp:126:22: error: 'obs' was not declared in this scope
obj1.display(obs); ^~~ Main.cpp:126:22: note: suggested alternative: 'abs' obj1.display(obs); ^~~ abs make: *** [test.o] Error 1
II](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb911f25f-f134-4b9f-b878-c5ce697e6605%2F4b186f6d-89cb-4d64-bd82-ca9ef90518af%2Fjd0jk3_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

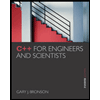
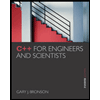