Please write a C++ program that will accept 3 integer numbers from the user, and then output these 3 numbers in ascending order, their sum, and their average. You must stop your program when the first number from the user is -999. Program Design Specifications and Regulations: (1) You must use your full name on your welcome and thank-you messages. (2) You must specify 3 prototypes in your program (before main( ) ) for 3 functions as follows: int max(int p, int q, int r); // prototype to return maximum of p, q, r int min(int p, int q, int r); // prototype to return minimum of p, q, r int mid(int p, int q, int r); // prototype to return middle of p, q, r (3) You must define the above three integer functions properly in your program after main( ). If you define those three functions before main( ), you should not specify those 3 prototypes. (4) You must NOT use any C++ existing or pre-defined functions in your program. (5) You must fully test your program to make sure that these three functions are working perfectly for any 3 numbers in any sequence. (6) How to show those 3 numbers (p, q, r) in ascending order without sorting them at all? The answer is that you show their minimum number, middle number, and maximum number. (7) How to check and detect that q is the middle number among p, q, and r? The answer is that you need to consider two cases as follows: if (q >= p && q <= r) return q ; // case 1: r, q , p i.e., r <= q <= p if (q >= r && q <= p) return q ; // case 2: p, q, r i.e., p <= q <= r ===========================================================================. Your test case #1 must look exactly like the output in the picture, including the data. You must also do test case #2 and test case #3 with different sets of data to prove that your program is working perfectly for any data. Each test case or test run must begin with a welcome message, and must end with a thank-you message
Please write a C++ program that will accept 3 integer numbers from the user, and then output these 3 numbers in ascending order, their sum, and their average. You must stop your program when the first number from the user is -999. Program Design Specifications and Regulations:
(1) You must use your full name on your welcome and thank-you messages.
(2) You must specify 3 prototypes in your program (before main( ) ) for 3 functions as follows: int max(int p, int q, int r); // prototype to return maximum of p, q, r int min(int p, int q, int r); // prototype to return minimum of p, q, r int mid(int p, int q, int r); // prototype to return middle of p, q, r
(3) You must define the above three integer functions properly in your program after main( ). If you define those three functions before main( ), you should not specify those 3 prototypes.
(4) You must NOT use any C++ existing or pre-defined functions in your program.
(5) You must fully test your program to make sure that these three functions are working perfectly for any 3 numbers in any sequence.
(6) How to show those 3 numbers (p, q, r) in ascending order without sorting them at all? The answer is that you show their minimum number, middle number, and maximum number.
(7) How to check and detect that q is the middle number among p, q, and r?
The answer is that you need to consider two cases as follows:
if (q >= p && q <= r) return q ; // case 1: r, q , p i.e., r <= q <= p
if (q >= r && q <= p) return q ; // case 2: p, q, r i.e., p <= q <= r ===========================================================================.
Your test case #1 must look exactly like the output in the picture, including the data. You must also do test case #2 and test case #3 with different sets of data to prove that your program is working perfectly for any data. Each test case or test run must begin with a welcome message, and must end with a thank-you message.
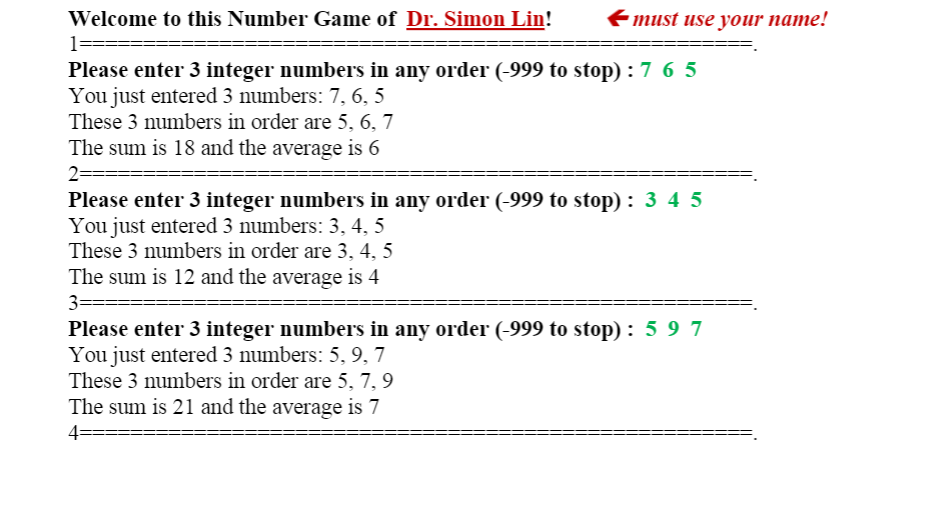
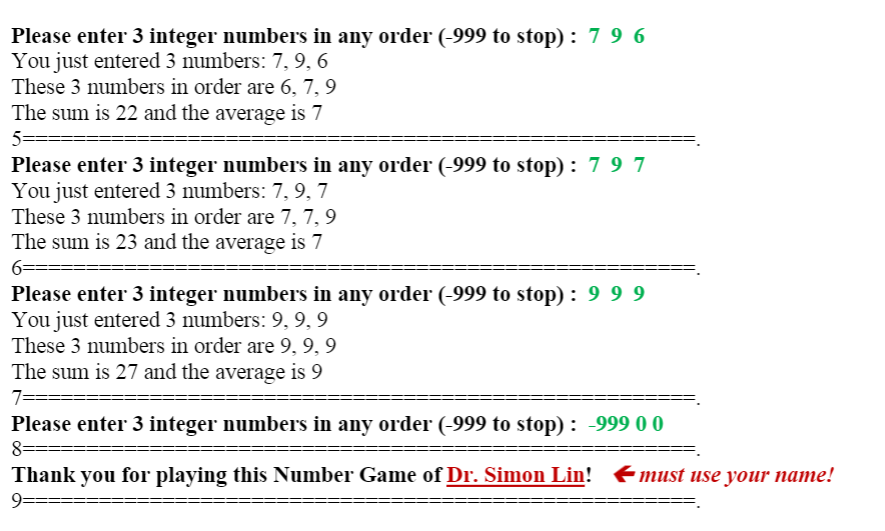

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images
