There are 4 types of passengers in the system: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system: StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers. ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators. FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items. GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile item public class Simulation { SimulatorSettings settings = new SimulatorSettings("settings.txt"); public void InitSimulation() throws FileNotFoundException{ ///// Read all parameters from the file and store in the clas File file = new File("settings.txt"); Scanner scanner = new Scanner(file); //FileReader freader = new FileReader(file); while(scanner.hasNextLine()){ String line = scanner.nextLine(); if(line.startsWith("floor=")) { line= line.replace("floor=", ""); // Convert the value to the wanted type System.out.println(line); } } settings.setNofloors(55); Passenger pass1 = new StandardPassenger(); pass1.requestElevator(settings); ArrayList passengers = null; for(int i = 0; i < 100; i++){ //Use the percentage from the file passengers.add(new StandardPassenger()); } } } public class SimulatorSettings { SimulatorSettings(String fileName){ } private int nofloors; public int getNofloors() { return nofloors; } public void setNofloors(int nofloors) { this.nofloors = nofloors; } } public abstract class Passenger { public static int passangerCounter = 0; private String passengerID; protected int startFloor; protected int endFloor; Passenger(){ this.passengerID = ""+passangerCounter; passangerCounter++; } public abstract boolean requestElevator(SimulatorSettings settings); } public class StandardPassenger extends Passenger{ private String type; public StandardPassenger() { } public boolean requestElevator(SimulatorSettings settings){ Random rand = new Random(); this.startFloor = rand.nextInt()% settings.getNofloors(); this.endFloor = rand.nextInt() % settings.getNofloors(); while(this.startFloor == this.endFloor){ this.endFloor = rand.nextInt() % settings.getNofloors(); } return true; } } public class VIPPassenger extends Passenger{ } Elevator abstract class public abstract class Elevator { protected String type; protected int capacity; protected int currentFloor; protected boolean isGoingUp; protected ArrayList passengers; public Elevator(String type, int capacity) { this.type = type; this.capacity = capacity; this.currentFloor = 1; this.isGoingUp = true; this.passengers = new ArrayList(); } public String getType() { return this.type; } public int getCapacity() { return this.capacity; } public int getCurrentFloor() { return this.currentFloor; } public void addPassenger(Passenger p) { if (passengers.size() < capacity) { passengers.add(p); } else { System.out.println("Elevator is full!"); } } public void removePassenger(Passenger p) { if (passengers.contains(p)) { passengers.remove(p); } else { System.out.println("Passenger is not in elevator!"); } } public void moveUp() { if (currentFloor < 10) { currentFloor++; } else { System.out.println("Cannot go up, already on top floor!"); } } public void moveDown() { if (currentFloor > 1) { currentFloor--; } else { System.out.println("Cannot go down, already on ground floor!"); } }
Help, I tried to write what I could but I am not sure what to write next. The code need to include Poylmorphism of passenger and elevator. Any help would be appreciated.
There are 4 types of passengers in the system:
Standard: This is the most common type of passenger and has a request percentage of 70%.
Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority
and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large
items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items
that need to be transported and are more likely to be picked up by glass elevators.
There are 4 types of elevators in the system:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a
maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a
maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a
maximum capacity of 6 passengers and are designed to transport fragile item
public class Simulation {
SimulatorSettings settings = new SimulatorSettings("settings.txt");
public void InitSimulation() throws FileNotFoundException{
///// Read all parameters from the file and store in the clas
File file = new File("settings.txt");
Scanner scanner = new Scanner(file);
//FileReader freader = new FileReader(file);
while(scanner.hasNextLine()){
String line = scanner.nextLine();
if(line.startsWith("floor="))
{
line= line.replace("floor=", "");
// Convert the value to the wanted type
System.out.println(line);
}
}
settings.setNofloors(55);
Passenger pass1 = new StandardPassenger();
pass1.requestElevator(settings);
ArrayList<Passenger> passengers = null;
for(int i = 0; i < 100; i++){
//Use the percentage from the file
passengers.add(new StandardPassenger());
}
}
}
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
public abstract class Passenger {
public static int passangerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger(){
this.passengerID = ""+passangerCounter;
passangerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
public class StandardPassenger extends Passenger{
private String type;
public StandardPassenger() {
}
public boolean requestElevator(SimulatorSettings settings){
Random rand = new Random();
this.startFloor = rand.nextInt()% settings.getNofloors();
this.endFloor = rand.nextInt() % settings.getNofloors();
while(this.startFloor == this.endFloor){
this.endFloor = rand.nextInt() % settings.getNofloors();
}
return true;
}
}
public class VIPPassenger extends Passenger{
}
Elevator abstract class
public abstract class Elevator {
protected String type;
protected int capacity;
protected int currentFloor;
protected boolean isGoingUp;
protected ArrayList<Passenger> passengers;
public Elevator(String type, int capacity) {
this.type = type;
this.capacity = capacity;
this.currentFloor = 1;
this.isGoingUp = true;
this.passengers = new ArrayList<Passenger>();
}
public String getType() {
return this.type;
}
public int getCapacity() {
return this.capacity;
}
public int getCurrentFloor() {
return this.currentFloor;
}
public void addPassenger(Passenger p) {
if (passengers.size() < capacity) {
passengers.add(p);
} else {
System.out.println("Elevator is full!");
}
}
public void removePassenger(Passenger p) {
if (passengers.contains(p)) {
passengers.remove(p);
} else {
System.out.println("Passenger is not in elevator!");
}
}
public void moveUp() {
if (currentFloor < 10) {
currentFloor++;
} else {
System.out.println("Cannot go up, already on top floor!");
}
}
public void moveDown() {
if (currentFloor > 1) {
currentFloor--;
} else {
System.out.println("Cannot go down, already on ground floor!");
}
}
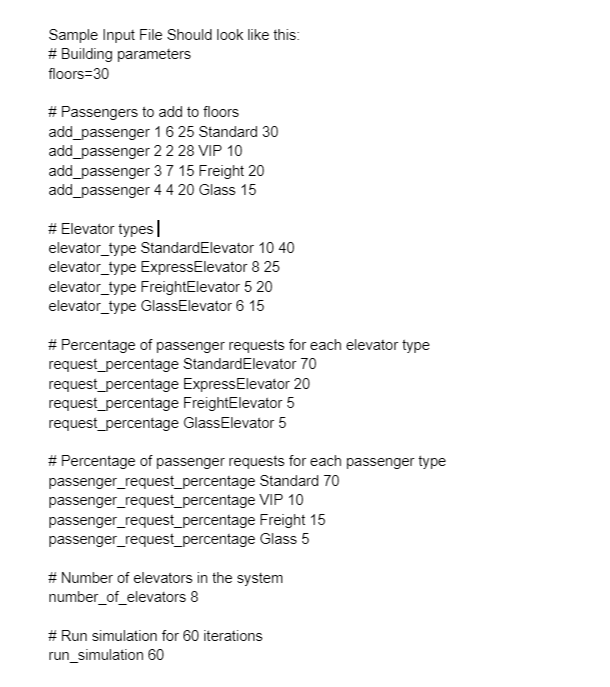

Step by step
Solved in 3 steps

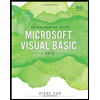
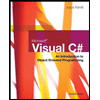
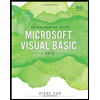
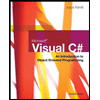