There is a segmentation fault when sorting. Where did I do wrong? The C code is written below #include #include struct Wstation { int id; double latitude; double longitude; int capacity; char url[100]; }; struct Wstation *stations; int read_ws(const char *fname) { FILE *fp = fopen(fname, "r"); if (!fp) { printf("not found %s\n", fname); return 0; } int num; fscanf(fp, "%d", &num); stations = malloc(num * sizeof(struct Wstation)); for (int i = 0; i < num; i++) { fscanf(fp, "%d, %lf, %lf, %d, %s", &stations[i].id, &stations[i].latitude, &stations[i].longitude, &stations[i].capacity, stations[i].url); } free(stations); fclose(fp); return num; } int cmp(const void *p1, const void *p2) { struct Wstation s1 = *(struct Wstation *)p1; struct Wstation s2 = *(struct Wstation *)p2; return s1.capacity - s2.capacity; } int main(void) { int N; N = read_ws("./waters-1.txt"); qsort(stations, N, sizeof(struct Wstation), cmp); printf("The stop with the largest capacity is \n"); printf("%d, %lf, %lf, %d, %s\n", stations[N-1].id, stations[N-1].latitude, stations[N-1].longitude, stations[N-1].capacity, stations[N-1].url); return 0; }
There is a segmentation fault when sorting. Where did I do wrong?
The C code is written below
#include <stdio.h>
#include <stdlib.h>
struct Wstation {
int id;
double latitude;
double longitude;
int capacity;
char url[100];
};
struct Wstation *stations;
int read_ws(const char *fname) {
FILE *fp = fopen(fname, "r");
if (!fp) {
printf("not found %s\n", fname);
return 0;
}
int num;
fscanf(fp, "%d", &num);
stations = malloc(num * sizeof(struct Wstation));
for (int i = 0; i < num; i++) {
fscanf(fp, "%d, %lf, %lf, %d, %s", &stations[i].id, &stations[i].latitude, &stations[i].longitude,
&stations[i].capacity, stations[i].url);
}
free(stations);
fclose(fp);
return num;
}
int cmp(const void *p1, const void *p2) {
struct Wstation s1 = *(struct Wstation *)p1;
struct Wstation s2 = *(struct Wstation *)p2;
return s1.capacity - s2.capacity;
}
int main(void) {
int N;
N = read_ws("./waters-1.txt");
qsort(stations, N, sizeof(struct Wstation), cmp);
printf("The stop with the largest capacity is \n");
printf("%d, %lf, %lf, %d, %s\n", stations[N-1].id, stations[N-1].latitude, stations[N-1].longitude,
stations[N-1].capacity, stations[N-1].url);
return 0;
}

Step by step
Solved in 2 steps with 1 images

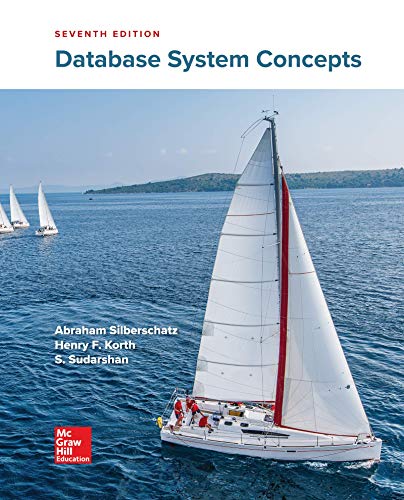
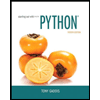
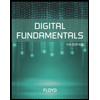
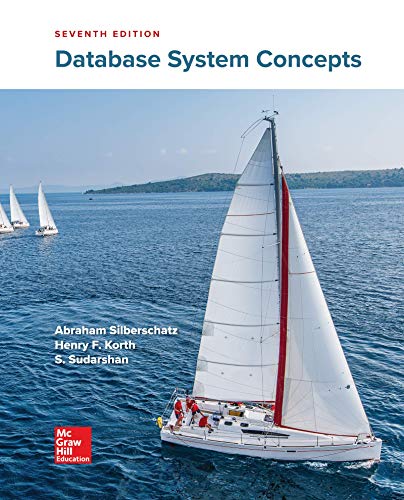
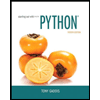
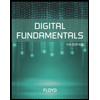
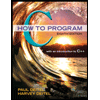
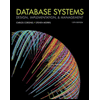
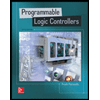