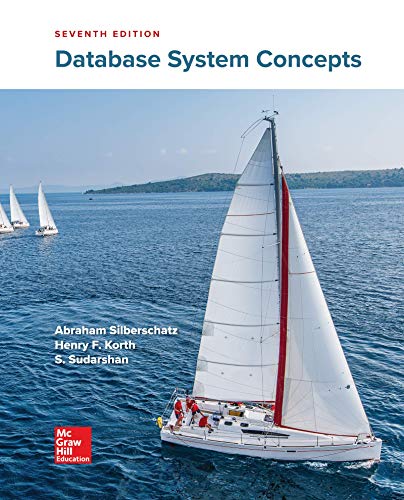
Concept explainers
When I attempt to run this program, I am getting an ArrayIndexOutOfBounds Exception on line 21 of the given Main. What is causing this to occur and what can be done to fix it?
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.LinkedList;
public class Main{
public static void main(String[] args) throws IOException {
// Create a new LinkedList object for holding the ValueData object:
LinkedList<ValueData> linkedList = new LinkedList<>();
// Create a new ObjectStack object
ObjectStack objectStack = new ObjectStack();
// Read a text file containing lines (records) of ValueData.
// This is in order to fill the LinkedList with ValueData from the file.
BufferedReader reader = new BufferedReader(new FileReader("values.txt"));
String line = reader.readLine();
while (line != null) {
String[] parts = line.split(",");
String name = parts[0].trim();
int value = Integer.parseInt(parts[1].trim());
ValueData data = new ValueData(name, value);
linkedList.add(data);
objectStack.push(data);
line = reader.readLine();
}
reader.close();
// Use the linkedList and objectStack objects as needed.
}
}
___________________________________________________________________________________________
ObjectStack.java:
import java.util.EmptyStackException;
public class ObjectStack extends LinkedList implements Stack {
private int size;
// Creates a new instance of ObjectStack:
public ObjectStack() {
super();
}
@Override
public void push(Object item) {
// Method body for push()
}
@Override
public Object pop() throws EmptyStackException {
// Method body for pop()
return null;
}
@Override
public Object top() throws EmptyStackException {
// Method body for top()
return null;
}
@Override
public boolean isEmpty() {
// Method body for isEmpty()
return false;
}
@Override
public int size() {
// Method body for size()
return 0;
}
}
__________________________________
Stack.java (interface):
import java.util.EmptyStackException;
public interface Stack {
public int size();
public boolean isEmpty();
public Object top () throws EmptyStackException;
public Object pop () throws EmptyStackException;
public void push (Object item);
}
___________________________________
LinkedListNode.java:
public class LinkedListNode
{
private Object data;
private LinkedListNode next;
// Constructor:
public LinkedListNode(Object data) {
this.data = data;
this.next = null;
}
// Getter and setter:
public LinkedListNode getNext() {
return next;
}
public void setNext(LinkedListNode next) {
this.next = next;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
public Object getItem() {
return null;
}
}
__________________________________________
LinkedList.java:
public class LinkedList{
private Node head; // head node of the list
/* Default constructor that creates an empty list */
public LinkedList() {
head = null;
}
//inserts new node at beginning of list
public void insert(Node v){
v.setNext(head);
head = v;
}
//searches elements of linked list for a match
public String search (String target) {
Node temp = head;
while(temp != null){
if(target.equals(temp.getElement())){
return "Found the target";
}
temp = temp.getNext();
}
return "Target not found";
}
//remove the first node of the array
public void removeFirstNode( ) {
if(head != null){
head = head.getNext();
}
}
//removes node with element matching the string target
public void removeNode( String target) {
Node prev = null;
Node curr = head;
while(curr != null){
if(target.equals(curr.getElement())){
break;
}
prev = curr;
curr = curr.getNext();
}
if(curr == null)
return;
if(prev == null){
removeFirstNode();
return;
}
prev.setNext(curr.getNext());
}
//loop from first to last element and prints them all
public void printList(){
Node temp = head;
while(temp != null){
System.out.print(temp.getElement() + " ");
temp = temp.getNext();
}
System.out.println();
}
}//end of class
______________________________________
Node.java:
public class Node {
private String element; // we assume elements are character strings
private Node next;
/* Creates a node with the given element and next node. */
public Node(String s, Node n) {
element = s;
next = n;
}
/* Returns the element of this node. */
public String getElement() {
return element;
}
/* Returns the next node of this node. */
public Node getNext() {
return next;
}
// Modifier methods:
/* Sets the element of this node. */
public void setElement(String newElem) {
element = newElem;
}
/* Sets the next node of this node. */
public void setNext(Node newNext) {
next = newNext;
}
}
__________________________________________
ValueData.java:
public class ValueData {
private String variable;
private double value;
// Define the constructor:
public ValueData(String variable, double value) {
this.variable = variable;
this.value = value;
}
// Define the getter and setter:
public String getVariable() {
return variable;
}
public void setVariable(String variable) {
this.variable = variable;
}
public double getValue() {
return value;
}
public void setValue(double value) {
this.value = value;
}
// Define the toString method:
@Override
public String toString() {
return "Variable: " + variable + ", Value: " + value;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- JAVA PROGRAM MODIFY AND CHANGE THIS PROGRAM EVEN MORE FOR THE FOLLOWING: IT MUST PASS THE TEST CASE WHEN I UPLOAD IT TO HYPERGRADE. I EVEN CHANGED THE TEXT FILES NAMES. I HAVE ALSO PROVIDED THE BOYMNAMES.TXT AND GIRLNAMES.TXT WHICH ARE INPUTS AND THE FAILED TEST CASES AS A SCREENSHOT. THANK YOU import java.io.*;import java.util.*;public class NameSearcher { private static List<String> loadFileToList(String filename) throws FileNotFoundException { List<String> namesList = new ArrayList<>(); File file = new File(filename); if (!file.exists()) { throw new FileNotFoundException(filename); } try (Scanner scanner = new Scanner(file)) { while (scanner.hasNextLine()) { String line = scanner.nextLine().trim(); String[] names = line.split("\\s+"); for (String name : names) { namesList.add(name.toLowerCase()); } } }…arrow_forwardi am having trouble with my code, I believe I need to change the bold information but don't know what to replace it with in order to display my required output import java.io.*;import java.util.*; public class AccountBalance { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); List<Account> list = new ArrayList<>(); try { FileInputStream file = new FileInputStream("accounts-with-names.dat"); DataInputStream input = new DataInputStream(file); // Read data from the file and store it in an array or list here while (input.available() > 0) { String name = input.readUTF(); long accountNumber = input.readLong(); double balance = input.readDouble(); boolean cashBack = input.readBoolean(); Account acc = new Account(name, accountNumber, balance, cashBack); list.add(acc); }…arrow_forwardI need this in JAVAarrow_forward
- Why do I get this error in my java program? Whenever I print a number greater than the amount of edges, I get an "Exception in thread "main" java.lang.ArrayIndexOutOfBounds" error. Please help. My code as well as the error (the picture with the red circle) are provided in the pictures.arrow_forwardChange the java code below to handle interrupted exceptions, and also to input the integer to multiply by sleeptime. public class SleepUtilities { /** * Nap between zero and NAP_TIME seconds. */ public static void nap() { nap(NAP_TIME); } /** * Nap between zero and duration seconds. */ public static void nap(int duration) { int sleeptime = (int) (NAP_TIME * Math.random() ); try { Thread.sleep(sleeptime*1000); } catch (InterruptedException e) {} } private static final int NAP_TIME = 5; }arrow_forwardI am writing a method to remove the first element in a doubly linked list. However I am supposed to throw an exception when the list is empty. When I test my class I keep getting "testRemoveFirst caused an ERROR: Uncompilable source code - exception Exceptions.EmptyCollectionException is never thrown in body of corresponding try statement" What does that mean and why is it not able to run?(I'm coding in Java)arrow_forward
- Getting this error when trying to run this program - java.lang.ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1 here is the program below. import java.util.ArrayList;import java.util.Scanner;import java.io.File;import java.io.FileNotFoundException; public class Largest_Country{public static ArrayList<Country> readCountry(String filename) throws FileNotFoundException { // Create an arraylist of the country class ArrayList<Country> countries = new ArrayList<Country>(); // Create a scanner object to open the input file Scanner scan = new Scanner(new File(filename)); // Initialize a string variable to read the file String line = ""; while(scan.hasNextLine()) { // Read the file line by line line = scan.nextLine(); // Split the line at spaces, and assign the values to // a string array String[] details = line.split(" "); // Create object of the class Country, and pass the values // to the object Country country_info = new Country(details[0],…arrow_forwardJava Program ASAP Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. Also change the program so that for test cases 2 and 3 the numbers are in there correct places as shown in the input files import java.io.*;import java.util.Scanner;public class FileSorting { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.equalsIgnoreCase("QUIT")) { break; // Exit the program } else { String filePath = new File("").getAbsolutePath() + "/" + input; File file = new File(filePath); if (file.exists() && !file.isDirectory()) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { String st;…arrow_forwardI ran the code and got an error. I even created a main.java file to run the test cases. Please fix the error and provide me the correct code for all parts. Make sure to give the screenshot of the output as well.arrow_forward
- I need help with this Java code, I have already found all the errors and I am getting the correct output. However; in regards to the next part, I have a hard time with loops. I need help creating a Do-while loop to validate all user input and handle exceptions. Please no advanced code, as I am new to Java.arrow_forwardimport java.io.BufferedReader;import java.io.File;import java.io.FileReader;import java.util.Scanner;public class romeoJuliet {public static void main(String[]args) throws Exception{Scanner keyboard=new Scanner(System.in);System.out.println("Enter a filename");String fN=keyboard.nextLine();int wLC=0, lC=0;String sW= keyboard.nextLine();File file=new File(fN);String [] wordSent=null;FileReader fO=new FileReader(fN);BufferedReader buff= new BufferedReader(fO);String sent;while((sent=buff.readLine())!=null){if(sent.contains(sW)){wLC++;}lC++;}System.out.println(fN+" has "+lC+" lines");System.out.println("Enter some text");System.out.println(wLC+" line(s) contain \""+sW+"\"");fO.close();}} Need to adjust code so that it reads all of the words that it was tasked to find. for some reason it isn't finding all of the word locations in a text. for example the first question is to find "the" in romeo and juliet which should have 1137, but it's only picking up 1032?arrow_forwardI'm still getting these errors when I run the code. Exception in thread "main" java.lang.NumberFormatException: For input string: "hov" at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67) at java.base/java.lang.Integer.parseInt(Integer.java:661) at java.base/java.lang.Integer.parseInt(Integer.java:777)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
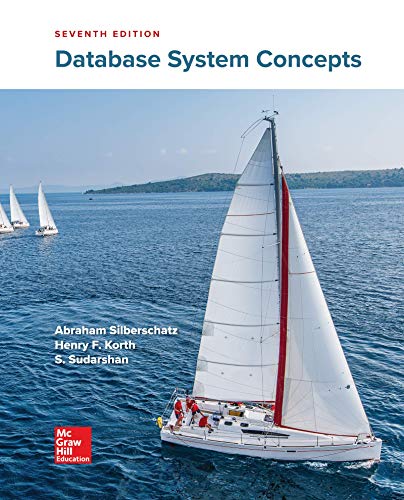
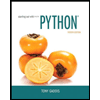
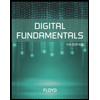
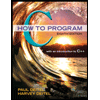
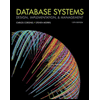
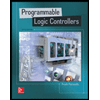