This is exercise builds on the previous Exercise (Inheritance 2) classes. In this series of exercises we'll take the StudentType class and expand it to also hold a list of courses that the student has taken. Starting out we want to build a CourseType class that will hold the basic course information
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
This is exercise builds on the previous Exercise (Inheritance 2) classes. In this series of exercises we'll take the StudentType class and expand it to also hold a list of courses that the student has taken.
Starting out we want to build a CourseType class that will hold the basic course information:
- Create a CourseType class. (Keep it simple at this point. You don't need to put in "extra" members just to make it a bigger class.)
- You should create this class with its own .cpp and .h file, but include it in a "project" with your already existing StudentType class. (You can use your own StudentType solution or the one provided from the previous exercise.) Note: At this point you shouldn't be changing anything in the existing StudentType files!
- Make sure you test your new CourseType class by declaring an object and testing at least it's constructor.
Submit: Commented test, implementation and header files (.cpp and .h) for new CourseType along with previous StudentType and PersonType zipped into a single
I have submitted a picture of my personType.h file and personType.cpp implementation file as context. I just need a very, very simple courseType class .h and .cpp file
These are the studentType header and Implementation files I have:
//studentType.h
#ifndef STUDENTTYPE_H_INCLUDED
#define STUDENTTYPE_H_INCLUDED
#include "personType.h"
#include <iostream>
#include <string>
//base class = personType , derived class = studentType
class studentType : public personType {
public:
//constructor
studentType();
//first name and last name
studentType (string, string);
//first name, last name, student ID, major declaration, GPA
studentType (string, string, int, string, double);
//acquire GPA
void setGPA (double);
//acquire unique numerical student ID
void setID (int);
//assigns Major declaration based on student's curriculum
void setMajor (string);
//overrides parents' print function
void print() const;
//get's GPA based on calculated GPA
double getGPA() const;
//get function that acquires student's unique ID
int getID() const;
//get function that acquire's student major declaration
string getMajor() const;
private:
//variable for student's unique ID
int id;
//variable that stores GPA
double gpa;
//variable that stores major declaration
string major;
};
#endif // STUDENTTYPE_H_INCLUDED
//studentTypeImp.cpp
#include "studentType.h"
#include <iostream>
#include <string>
using namespace std;
studentType::studentType() : personType ("","") {
id = 0;
major = "";
gpa = 0;
}
studentType::studentType (string first, string last) : personType() {
id = 0;
major = "";
gpa = 0;
}
studentType::studentType (string first, string last, int id, string major,
double gpa) : personType (first, last) {
this -> id = id;
this -> major = major;
this -> gpa = gpa;
}
void studentType::setGPA (double gpa) {
this -> gpa = gpa;
}
void studentType::setID (int id) {
this -> id = id;
}
void studentType::setMajor (string major) {
this -> major = major;
}
void studentType::print() const {
personType::print();
cout << " ID: " << id;
cout << " Major: " << major;
cout << " GPA: " << gpa;
}
double studentType::getGPA() const {
return gpa;
}
int studentType::getID() const {
return id;
}
string studentType::getMajor() const {
return major;
}
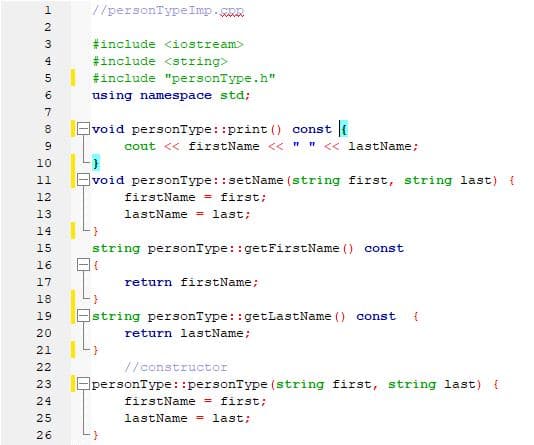
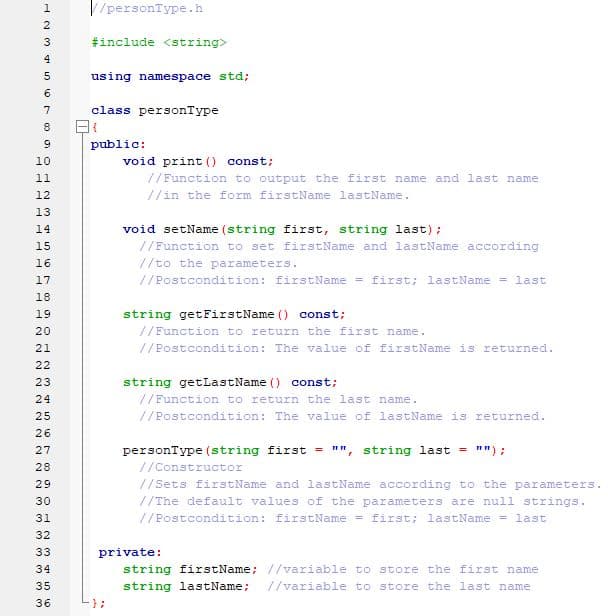

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

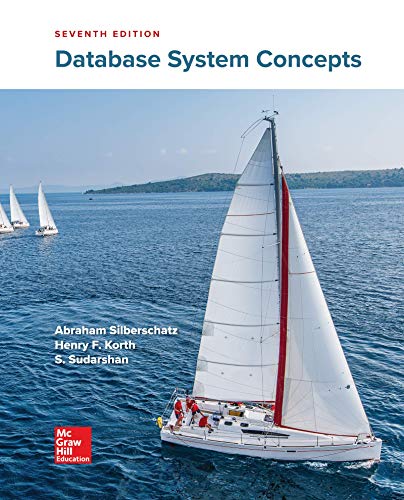
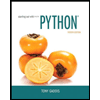
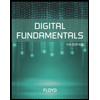
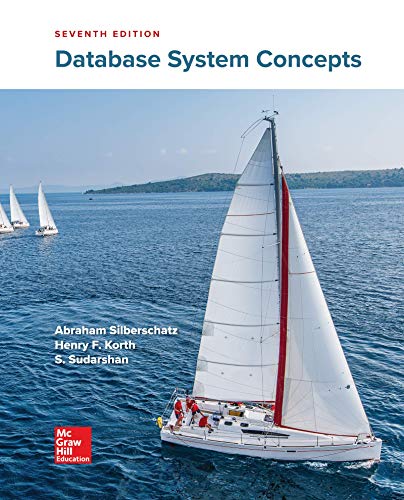
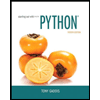
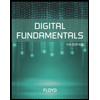
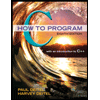
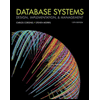
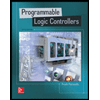