This is my C++ Codes but it has errors when I run it. The error states that at line 16, the expression must have a constant value. Can you fix the errors? Please still use header files iostream. //Start of program #include using namespace std; int main() { //Initialize variable int size; //Prompt the user to enter the size of the array N cout << "Enter the size of the array N: "<> size; //Declare array and initialize variables int N[size], i, j, temp; int count = 1; //Prompt the user to enter the integer value for the array N cout << "Enter the " << size << " elements for the array N: "; //Use for loop to iterate the value of i for (i = 0; i < size; i++) { cin >> N[i]; //Store each integer } //Nested loop for (i = 0; i < size; i++) //Outer for loop { for (j = i + 1; j < size; j++) //Inner for loop { if (N[i] > N[j]) //If the element at i is greater than the element at j { temp = N[i]; //Swap elements N[i] = N[j]; N[j] = temp; } } } //Display the heading cout << "\n\t\tN \t count"; cout << "\n\t ---------------"; //Count the number of occurrences //Nested loop for (i = 0; i < size; i++) //Outer for loop { for (j = 0; j < i; j++) //Inner for loop { //If the elements at i and j are same, count if (N[i] == N[j]) count++; } //If the values of the i and j are the same if (i == j) { //If the next element is not same as the current element if (N[i] != N[i + 1]) cout << "\n\t\t" << N[i] << "\t\t" << count; //Display the element and total number of occurrence of the element } } cout << endl; //End of program return 0; }
This is my C++ Codes but it has errors when I run it. The error states that at line 16, the expression must have a constant value. Can you fix the errors? Please still use header files iostream.
//Start of program
#include <iostream>
using namespace std;
int main()
{
//Initialize variable
int size;
//Prompt the user to enter the size of the array N
cout << "Enter the size of the array N: "<<endl;
cin >> size;
//Declare array and initialize variables
int N[size], i, j, temp;
int count = 1;
//Prompt the user to enter the integer value for the array N
cout << "Enter the " << size << " elements for the array N: ";
//Use for loop to iterate the value of i
for (i = 0; i < size; i++)
{
cin >> N[i]; //Store each integer
}
//Nested loop
for (i = 0; i < size; i++) //Outer for loop
{
for (j = i + 1; j < size; j++) //Inner for loop
{
if (N[i] > N[j]) //If the element at i is greater than the element at j
{
temp = N[i]; //Swap elements
N[i] = N[j];
N[j] = temp;
}
}
}
//Display the heading
cout << "\n\t\tN \t count";
cout << "\n\t ---------------";
//Count the number of occurrences
//Nested loop
for (i = 0; i < size; i++) //Outer for loop
{
for (j = 0; j < i; j++) //Inner for loop
{
//If the elements at i and j are same, count
if (N[i] == N[j])
count++;
}
//If the values of the i and j are the same
if (i == j)
{
//If the next element is not same as the current element
if (N[i] != N[i + 1])
cout << "\n\t\t" << N[i] << "\t\t" << count; //Display the element and total number of occurrence of the element
}
}
cout << endl;
//End of program
return 0;
}


Step by step
Solved in 4 steps with 2 images

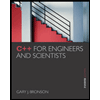
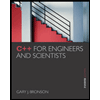