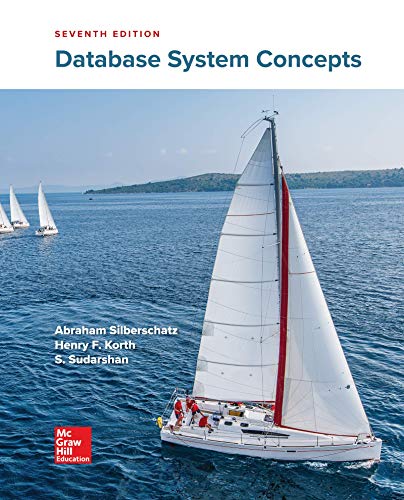
This is my C++ Codes for the problem but it has errors when I run it. Can you fix the errors? Please still use header files iostream. This is a topic under Data Structures: Arrays.
//Start of program
#include <iostream>
#define TRUE 0
#define FALSE 1
using namespace std;
int main()
{
//Declaration of array
int A[50];
//Display the first element of the array A
cout << "The value of the first element of the array A is: " << A[0] << endl;
//Store the value 26 in 25th element of array A
A[24] = 26;
//Store the computed value in 10th element of array A
A[9] = (A[39] * 3) + 10;
cout << "The value of the 10th element of the array A is: " << A[9] << endl;
int n = 0; //Subscript of array A
//For loop
for (int i = 0; i < n; i++)
{
if (n / 2 == 0 || n / 3 == 0) //If subscript is a multiple of 2 or 3
{
cout << A[i] << " " << endl; //Display the value of an element of array A
}
if (n / 2 != 0 || n / 3 != 0) //If subscript is not a multiple of 2 or 3
break; //Break out of loop
}
//End of program
return 0;
}
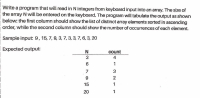

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C++arrow_forwardC++arrow_forwardExercise 2 This exercise is designed to assess student’s skills of writing programs using arrays and loops. Write a C++ program using array and loop that prompts a user to enter 15 Students’ marks in ProgramDesign and Implementation (PDI) Module in an array. The program sorts the students’ marks in descending order using a loop and displays the marks of all students as well as lowest, highest, average marks, and the class result based on the average module marks. The result criteria is given below:Average is less than 40 = Poor ResultAverage is between 40 to 49 = Fair ResultAverage is less than 50 to 59= Good ResultAverage is less than 60 to 69 = Very Good ResultAverage is between 70 to 79 = Excellent ResultAverage is 80 or greater = Outstanding ResultNote: You must demonstrate the use of arrays and loops. Your code must be properly commented Sample Output:Welcome to Result Management SystemEnter PDI Marks of Student 1: 50Enter PDI Marks of Student 2: 35Enter PDI Marks of Student 3:…arrow_forward
- Topic: Array covered in Chapter 7 Do not use any topic not covered in lecture. Write a C++ program to store and process an integer array. The program creates an array (numbers) of integers that can store up to 10 numbers. The program then asks the user to enter up to a maximum of 10 numbers, or enter 999 if there are less than 10 numbers. The program should store the numbers in the array. Then the program goes through the array to display all even (divisible by 2) numbers in the array that are entered by the user. Then the program goes through the array to display all odd (not divisible by 2) numbers in the array that are entered by the user. At the end, the program displays the total sum of all numbers that are entered by the user.arrow_forwardC++ Visual 2019 Write a program that uses a two identical arrays of at least 100 randomly generated integers. It should call a function that uses the bubble sort algorithm to sort one of the arrays in ascending order. The function should keep a count of the number of exchanges it makes. The program then should call a function that uses the selection sort algorithm to sort the other array. It should also keep count of the number of exchanges it makes. Display these values on the screen with appropriate descriptions.arrow_forwardC++, Please add a function where it can display the median, the maximum number, the minimum number, in an array entered by the user. Code: #include<iostream> using namespace std; class Search_Dynamic_Array { public: Search_Dynamic_Array(); int getArrSize(); void getArray(); void setArrsize(int); void setArray(int*); int getSum(int*); private: int arr_size; int arr[]; }; Search_Dynamic_Array::Search_Dynamic_Array() { //ctor } int Search_Dynamic_Array::getArrSize() { return arr_size; } void Search_Dynamic_Array::getArray() { cout << "The elements of the array are: "; for(int i=0; i<arr_size; i++){ cout << arr[i] << " "; } } void Search_Dynamic_Array::setArrsize(int s) { arr_size=s; } void Search_Dynamic_Array::setArray(int a[]) { for(int i=0; i<arr_size; i++){ arr[i]=a[i]; } } int Search_Dynamic_Array::getSum(int a[]) { int sum; for(int i=0; i<arr_size; i++){ sum +=arr[i]; } return sum; } int main() { int s=1, a[s]; cout << "Enter the size of the…arrow_forward
- C PROGRAMMING HELP I need help with an old script of mine. I'm having trouble getting math involving accessing an array to work properly, I'm getting an error message that's puzzling me. I'm pretty sure the solution is simple and right in front of me but it's stumping me. Below is the script: #include <stdio.h>#include <math.h>void value(float x){ printf("what is the value of x?"); scanf("%f",&x); }void power(float i,int p,int x,float arr[100]){ float equation,butt; printf("what is the highest power in the series?"); scanf("%d",&p); for (i = 0; i < p; ++i) equation = pow(x,i); //how the hell do i get the counter to do what i want butt = 1+equation^arr[i];}void shortcut(int x){ shortcut = 1/(1-x)}int main(){ printf("Here is the result!:") printf("%d %f", p, f); printf("If we had used shortcut, value would be: %d", shortcut); int diff; diff = shortcut - butt; printf("The difference is only %0.2f!",diff);…arrow_forwardGood evening, i am working on this arrays problem and was hoping you could help with it please? (my programming language is c++) Thanks!arrow_forwardPlease complete the following guidelines and hints. Using C language. Please use this template: #include <stdio.h>#define MAX 100struct cg { // structure to hold x and y coordinates and massfloat x, y, mass;}masses[MAX];int readin(void){/* Write this function to read in the datainto the array massesnote that this function should return the number ofmasses read in from the file */}void computecg(int n_masses){/* Write this function to compute the C of Gand print the result */}int main(void){int number;if((number = readin()) > 0)computecg(number);return 0;}Testing your workTypical Input from keyboard:40 0 10 1 11 0 11 1 1Typical Output to screen:CoG coordinates are: x = 0.50 y = 0.50arrow_forward
- use c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forwardA1: File Handling with struct C++ This program is to read a given file and display the information about employees according to the type of employee. Specifically, the requirements are as follows. Read the given file of the information of employees. Store the information in an array or arrays. Display all the salaried employees first and then the hourly employees Prompt the user to enter an SSN and find the corresponding employee and display the information of that employee. NOTE : S- salaryemployee , H- Hourly employee S 135-25-1234 Smith Sophia DevOps Developer 70000H 135-67-5462 Johnaon Jacob SecOps Pentester 30 60.50S 252-34-6728 William Emma DevOps DBExpert 100000S 237-12-1289 Miller Mason DevOps CloudArchitect 80000S 581-23-4536 Jones Jayden SecOps Pentester 250000S 501-56-9724 Rogers Mia DevOps Auditor 90000H 408-67-8234 Cook Chloe DevOps QAEngineer 40 45.10S 516-34-6524 Morris Daniel DevOps ProductOwner 300000H 526-47-2435 Smith Natalie DevOps…arrow_forwardIn C++ Assume that a 5 element array of type string named boroughs has been declared and initialized. Write the code necessary to switch (exchange) the values of the first and last elements of the array.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
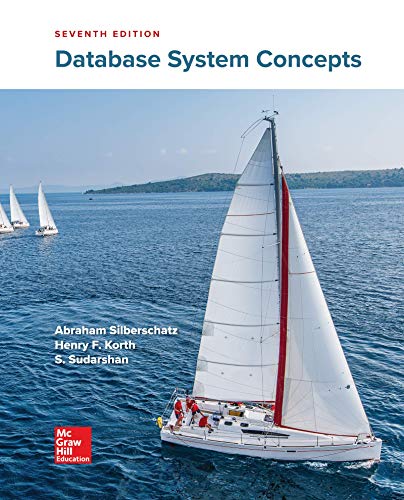
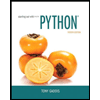
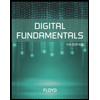
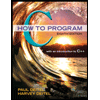
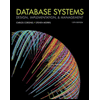
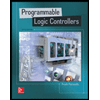