This is the question - Create a class named Apartment that holds an apartment number, number of bedrooms, number of baths, and rent amount. Create a constructor that accepts values for each data field. Also create a get method for each field. Write an application that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or No apartments met your criteria if no such apartments are available. This is the code it has given and I have done some on- public class Apartment { int aptNumber; int bedrooms; double baths; double rent; public Apartment(int num, int bdrms, double bths, double rent) { } public int getAptNumber() { return aptNumber; } public int getBedrooms() { retrun bedrooms; } public double getBaths() { return baths; } public double getRent() { return rent; } } import java.util.*; public class TestApartments { public static void main (String args[]) { Scanner input = new Scanner(System.in); Apartment apt1 = new Apartment(101, 2, 1, 725); Apartment apt2 = new Apartment(102, 2, 1.5, 775); Apartment apt3 = new Apartment(103, 3, 2, 870); Apartment apt4 = new Apartment(104, 3, 2.5, 960); Apartment apt5 = new Apartment(105, 3, 3, 1100); int bdrms; int baths; double rent; int count = 0; System.out.print("Enter minimum number of bedrooms needed >> "); bdrms = input.nextInt(); System.out.print("Enter minimum number of bathrooms needed >> "); baths = input.nextInt(); System.out.print("Enter maximum rent willing to pay >> "); rent = input.nextDouble(); System.out.println("\nApartments meeting citeria of\nat least " + bdrms + " bedrooms, at least " + baths + " baths, and " + " no more than $" + rent + " rent:"); // Write code here } public static boolean checkApt(Apartment apt, int bdrms, double baths, double rent) { // Write code here } public static void display(Apartment apt) { System.out.println(" Apt #" + apt.getAptNumber() + " " + apt.getBedrooms() + " bedrooms, and " + apt.getBaths() + " baths. Rent $" + apt.getRent()); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
This is the question -
Create a class named Apartment that holds an apartment number, number of bedrooms, number of baths, and rent amount. Create a constructor that accepts values for each data field. Also create a get method for each field.
Write an application that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or No apartments met your criteria if no such apartments are available.
This is the code it has given and I have done some on-

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

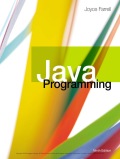
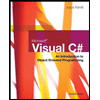
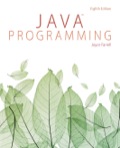
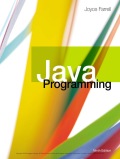
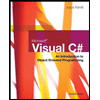
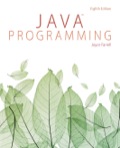
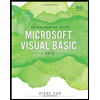