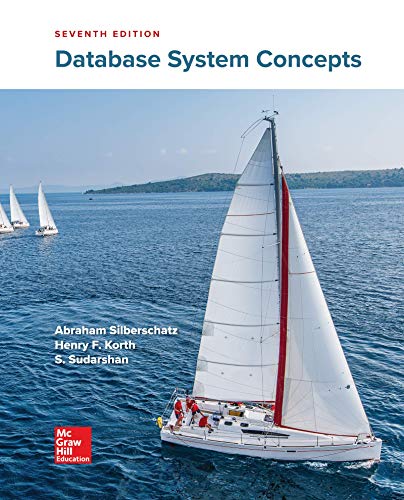
Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary linkedListLibrary) and the other implemented using the built-in ArrayList class (ArrayListLibrary arrayListLibrary). Each list contains 100 books (title, ISBN number, author), sorted in ascending order by ISBN number.
Complete main() by inserting a new book into each list using the respective LinkedListLibrary and ArrayListLibrary insertSorted() methods and outputting the number of operations the computer must perform to insert the new book. Each insertSorted() returns the number of operations the computer performs.
Ex: If the input is:
The Catcher in the Rye 9787543321724 J.D. Salinger
the output is:
Number of linked list operations: 1 Number of ArrayList operations: 1
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.IOException;
public class Library {
public static void fillLibraries(LinkedListLibrary linkedListLibrary, ArrayListLibrary arrayListLibrary) throws IOException {
FileInputStream fileByteStream = null; // File input stream
Scanner inFS = null; // Scanner object
int linkedListOperations = 0;
int arrayListOperations = 0;
BookNode currNode;
Book tempBook;
String bookTitle;
String bookAuthor;
long bookISBN;
// Try to open file
fileByteStream = new FileInputStream("Books.txt");
inFS = new Scanner(fileByteStream);
while (inFS.hasNextLine()) {
bookTitle = inFS.nextLine();
bookISBN = inFS.nextLong();
inFS.nextLine();
bookAuthor = inFS.nextLine();
// Insert into linked list
currNode = new BookNode(bookTitle, bookAuthor, bookISBN);
linkedListOperations = linkedListLibrary.insertSorted(currNode, linkedListOperations);
linkedListLibrary.lastNode = currNode;
// Insert into ArrayList
tempBook = new Book(bookTitle, bookAuthor, bookISBN);
arrayListOperations = arrayListLibrary.insertSorted(tempBook, arrayListOperations);
}
fileByteStream.close(); // close() may throw IOException if fails
}
public static void main (String[] args) throws IOException {
Scanner scnr = new Scanner(System.in);
int linkedListOperations = 0;
int arrayListOperations = 0;
// Create libraries
LinkedListLibrary linkedListLibrary = new LinkedListLibrary();
ArrayListLibrary arrayListLibrary = new ArrayListLibrary();
// Fill libraries with 100 books
fillLibraries(linkedListLibrary, arrayListLibrary);
// Create new book to insert into libraries
BookNode currNode;
Book tempBook;
String bookTitle;
String bookAuthor;
long bookISBN;
bookTitle = scnr.nextLine();
bookISBN = scnr.nextLong();
scnr.nextLine();
bookAuthor = scnr.nextLine();
// Insert into linked list
currNode = new BookNode(bookTitle, bookAuthor, bookISBN);
// TODO: Call LL_Library's insertSorted() method to insert currNode and return
// the number of operations performed
linkedListLibrary.lastNode = currNode;
// Insert into ArrayList
tempBook = new Book(bookTitle, bookAuthor, bookISBN);
// TODO: Call AL_Library's insertSorted() method to insert tempBook and return
// the number of operations performed
// TODO: Print number of operations for linked list
// TODO: Print number of operations for ArrayList
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 8 images

getting this issue: Library.java:118: error: class, interface, or enum expected import java.util.ArrayList; ^ 1 error
getting this issue: Library.java:118: error: class, interface, or enum expected import java.util.ArrayList; ^ 1 error
- The text's array-based list implementation stores elements in the lowest possible indices of the array. True or False screen shot shows the text's array based listarrow_forwardin Jave create a method work() with the follow instructions: If the work() method is passed a non-null then it must return a new List that contains some or all of the elements in data. The elements of the new List must be aliases for (not copies of) the objects in the List it is passed. If there are no sign attributes (i.e., the array is null or has 0 elements) then the work() method must return an empty List. If any of the conditions represented by the sign attribute holds for a particular element that that element must be included in the result (i.e., the conditions must be combined using a logical OR). The elements in the returned List must be in the same order they appeared in the original List (though not all elements must be in the result). public List<T> work(List<T> g){arrow_forwardGiven main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 main.py(can not edit) from IntNode import IntNodefrom IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py(can not edit) class IntNode: def __init__(self, initial_data, next = None, prev = None): self.data = initial_data self.next = next self.prev = prev IntList.py(need to edit)arrow_forward
- Has to be done in Java. Import ArrayList.arrow_forwardJava Complete a method calledRedundantCharacterMatch(ArrayList<Character> YourFirstName): the parameter of this method is an ArrayList<Character> whose elements are the characters in your first name (they should be in the order appear in your first name, e.g., if your first name is bob, then the ArrayList<Char> includes ‘b’, ‘o’, ‘b’.). The method will check whether there exists duplicate characters in your name and return the index of those duplicate characters. For example, when using bob as first name, it will return b: 0, 2. 2. Create an ArrayList<Character> NameExample. All the characters of your first name will appear twice in this ArrayList. For example, if your first name is bob, then NameExample will include the following element {b,o,b,b,o,b}. Then, please use NameExample as parameter for the method RedundantCharacterMatch(). If your first name is bob, the results that print in the console will be b: 0, 2, 3, 5 o: 1, 4arrow_forwardIn java Write a method public static ArrayList<Integer> merge(ArrayList<Integer> a, ArrayList<Integer> b) that merges one array list with another. Ex: a = 1 2 3 4 5, b = 6 7 8 9 10 then merge returns 1 6 2 7 3 8 4 9 5 10arrow_forward
- In python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardCan you please answer this fast and with correct answer. Thankyouarrow_forward
- Java's LinkedList provides a method listlterator(int index) returning a Listlterator for a list. Declare a variable of LinkedList of integer numbers and write a fragment of Java code to compute and print the sum of the numbers on the list. Do not write code to insert elements to the list and assume that the list already has elements on it. You must only use an iterator obtained from the list (you can not use the get(int index) method) .arrow_forwardPlease fill in all the code gaps if possible: (java) public class LinkedList { privateLinkedListNode head; **Creates a new instance of LinkedList** public LinkedList () { } public LinkedListNode getHead() public void setHead (LinkedListNode head) **Add item at the front of the linked list** public void insertFront (Object item) **Remove item at the front of linked list and return the object variable** public Object removeFront() }arrow_forwardWhich best describes this axiom: aList.getLength ( ) = ( aList.remove ( i ) ) . getLength ( ) + 1 You cannot remove an item from from existing list that is empty Removing an item from an existing list decreases its length by one Removing an item from an existing list increases the list size by one None of thesearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
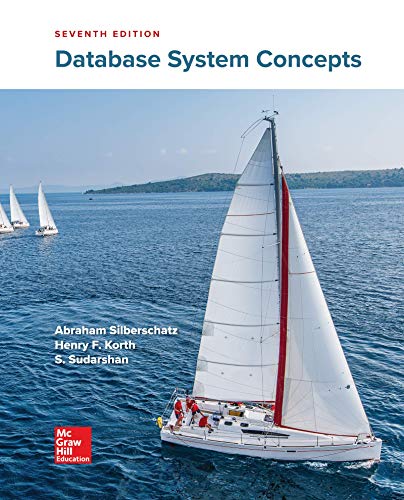
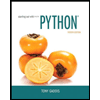
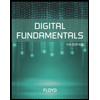
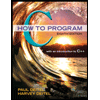
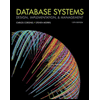
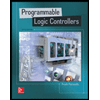