Import the ArrayList and List classes from the java.util package to create a list of phone numbers and also import the HashSet and Set classes from the java.util package to create a set of unique prefixes. Create a class called PhoneNumberPrefix with a main method that will contain the code to find the unique prefixes. Create a List called phoneNumbers and use the add method to add several phone numbers to the list. List phoneNumbers = new ArrayList<>(); phoneNumbers.add("555-555-1234"); phoneNumbers.add("555-555-2345"); phoneNumbers.add("555-555-3456"); phoneNumbers.add("444-444-1234"); phoneNumbers.add("333-333-1234"); Create a Set called prefixes and use a for-each loop to iterate over the phoneNumbers list. For each phone number, we use the substring method to extract the first 7 characters, which represent the prefix, and add it to the prefixes set using the add method. Finally, use the println method to print the prefixes set, which will contain all of the unique prefixes: --------------------------------------------------------------------------------------------------------------------------------------------------------- import java.util.ArrayList; // import ArrayList class import java.util.HashSet; // import HashSet class import java.util.List; // import List interface import java.util.Set; // import Set interface public class PhoneNumberPrefix { public static void main(String[] args) { // Create a list of phone numbers // Create a set of unique prefixes Set prefixes = new HashSet<>(); // Add the prefix of each phone number to the set // Print the unique prefixes System.out.println("Unique prefixes: " + prefixes); } }
-
Import the ArrayList and List classes from the java.util package to create a list of phone numbers and also import the HashSet and Set classes from the java.util package to create a set of unique prefixes.
-
Create a class called PhoneNumberPrefix with a main method that will contain the code to find the unique prefixes.
-
Create a List called phoneNumbers and use the add method to add several phone numbers to the list.
List<String> phoneNumbers = new ArrayList<>(); phoneNumbers.add("555-555-1234"); phoneNumbers.add("555-555-2345"); phoneNumbers.add("555-555-3456"); phoneNumbers.add("444-444-1234"); phoneNumbers.add("333-333-1234");
- Create a Set called prefixes and use a for-each loop to iterate over the phoneNumbers list. For each phone number, we use the substring method to extract the first 7 characters, which represent the prefix, and add it to the prefixes set using the add method.
-
Finally, use the println method to print the prefixes set, which will contain all of the unique prefixes:
---------------------------------------------------------------------------------------------------------------------------------------------------------

Step by step
Solved in 4 steps with 2 images

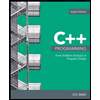
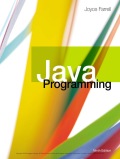
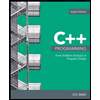
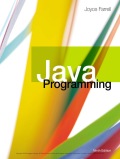