Use the Parser class to design a class called Dictionary. This class will hold word and their frequencies. Here is the UML diagram for the dictionary as well as the contract description : Dictionary -words:String[] -cnt : int[] -numWords : int +Dictionary() +Dictionary(capacity: int) +empty():boolean +locateWord: int +addWord (w : String): void +dumpDictionary(): void +showHistogram(): void +capacity(): int; An array to store words of the dictionary An array that stores the frequency of each word Number of words in the dictionary Constructs an empty Dictionary of with capacity=16 Constructs an empty Dictionary with specified capacity Returns true if Dictionary is empty Returns location of word in dictionary; -1 if it isn't in dictionary If word isn't in dictionary then word is added otherwise frequency is increased by 1. Displays contents of dictionary (word and frequency count) Displays histogram Returns capacity (ie length of arrays) Some things to consider when you are implementing this class : 1. both constructors are responsible for creating the arrays words and cnt. The second cons must make sure that capacity is >=0. If it is not then it will create the arrays with default size 16 ( just like the no-arg constructor). This is a perfect place to use the this pointer. Also, remember to use a named constant for the value 16. 2. Use the value of numElem to determine if the dictionary is empty. 3. The dictionary should never fill up. If you try to add another word to the dictionary and there is no room in arrays for that word, then allocate new arrays with twice the capacity of the current arrays, copy all elements in current arrays to the new arrays and make the new arrays your new word and cnt. The garbage collector will handle the old arrays. Question : how do you know that you have run out of room?
Use the Parser class to design a class called Dictionary. This class will hold word and their frequencies. Here is the UML diagram for the dictionary as well as the contract description : Dictionary -words:String[] -cnt : int[] -numWords : int +Dictionary() +Dictionary(capacity: int) +empty():boolean +locateWord: int +addWord (w : String): void +dumpDictionary(): void +showHistogram(): void +capacity(): int; An array to store words of the dictionary An array that stores the frequency of each word Number of words in the dictionary Constructs an empty Dictionary of with capacity=16 Constructs an empty Dictionary with specified capacity Returns true if Dictionary is empty Returns location of word in dictionary; -1 if it isn't in dictionary If word isn't in dictionary then word is added otherwise frequency is increased by 1. Displays contents of dictionary (word and frequency count) Displays histogram Returns capacity (ie length of arrays) Some things to consider when you are implementing this class : 1. both constructors are responsible for creating the arrays words and cnt. The second cons must make sure that capacity is >=0. If it is not then it will create the arrays with default size 16 ( just like the no-arg constructor). This is a perfect place to use the this pointer. Also, remember to use a named constant for the value 16. 2. Use the value of numElem to determine if the dictionary is empty. 3. The dictionary should never fill up. If you try to add another word to the dictionary and there is no room in arrays for that word, then allocate new arrays with twice the capacity of the current arrays, copy all elements in current arrays to the new arrays and make the new arrays your new word and cnt. The garbage collector will handle the old arrays. Question : how do you know that you have run out of room?
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter13: Overloading And Templates
Section: Chapter Questions
Problem 15PE
Related questions
Question
100%
how is this done in java? what is parser?
![Use the Parser class to design a class called Dictionary.
This class will hold word and their frequencies. Here is the UML diagram for the
dictionary as well as the contract description :
Dictionary
-words:String[]
-cnt : int[]
-numWords : int
+Dictionary()
+Dictionary(capacity: int)
+empty():boolean
+locateWord: int
+addWord (w : String): void
+dumpDictionary(): void
+showHistogram(): void
+capacity(): int;
An array to store words of the dictionary
An array that stores the frequency of each word
Number of words in the dictionary
Constructs an empty Dictionary of with capacity=16
Constructs an empty Dictionary with specified capacity
Returns true if Dictionary is empty
Returns location of word in dictionary; -1 if it isn't in
dictionary
If word isn't in dictionary then word is added otherwise
frequency is increased by 1.
Displays contents of dictionary (word and frequency count)
Displays histogram
Returns capacity (ie length of arrays)
Some things to consider when you are implementing this class :
1. both constructors are responsible for creating the arrays words and cnt.
The second constructor must make sure that capacity is >=0. If it is not then
it will create the arrays with default size 16 ( just like the no-arg
constructor). This is a perfect place to use the this pointer. Also, remember
to use a named constant for the value 16.
2. Use the value of numElem to determine if the dictionary is empty.
3. The dictionary should never fill up. If you try to add another word to the
dictionary and there is no room in arrays for that word, then allocate new
arrays with twice the capacity of the current arrays, copy all elements in
current arrays to the new arrays and make the new arrays your new word
and cnt. The garbage collector will handle the old arrays. Question : how
do you know that you have run out of room?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F66301e59-a86d-47c0-9dd8-21e24614422a%2F36397ebc-b5c1-43d8-b68e-ec2449ba4813%2F4maxd8_processed.png&w=3840&q=75)
Transcribed Image Text:Use the Parser class to design a class called Dictionary.
This class will hold word and their frequencies. Here is the UML diagram for the
dictionary as well as the contract description :
Dictionary
-words:String[]
-cnt : int[]
-numWords : int
+Dictionary()
+Dictionary(capacity: int)
+empty():boolean
+locateWord: int
+addWord (w : String): void
+dumpDictionary(): void
+showHistogram(): void
+capacity(): int;
An array to store words of the dictionary
An array that stores the frequency of each word
Number of words in the dictionary
Constructs an empty Dictionary of with capacity=16
Constructs an empty Dictionary with specified capacity
Returns true if Dictionary is empty
Returns location of word in dictionary; -1 if it isn't in
dictionary
If word isn't in dictionary then word is added otherwise
frequency is increased by 1.
Displays contents of dictionary (word and frequency count)
Displays histogram
Returns capacity (ie length of arrays)
Some things to consider when you are implementing this class :
1. both constructors are responsible for creating the arrays words and cnt.
The second constructor must make sure that capacity is >=0. If it is not then
it will create the arrays with default size 16 ( just like the no-arg
constructor). This is a perfect place to use the this pointer. Also, remember
to use a named constant for the value 16.
2. Use the value of numElem to determine if the dictionary is empty.
3. The dictionary should never fill up. If you try to add another word to the
dictionary and there is no room in arrays for that word, then allocate new
arrays with twice the capacity of the current arrays, copy all elements in
current arrays to the new arrays and make the new arrays your new word
and cnt. The garbage collector will handle the old arrays. Question : how
do you know that you have run out of room?
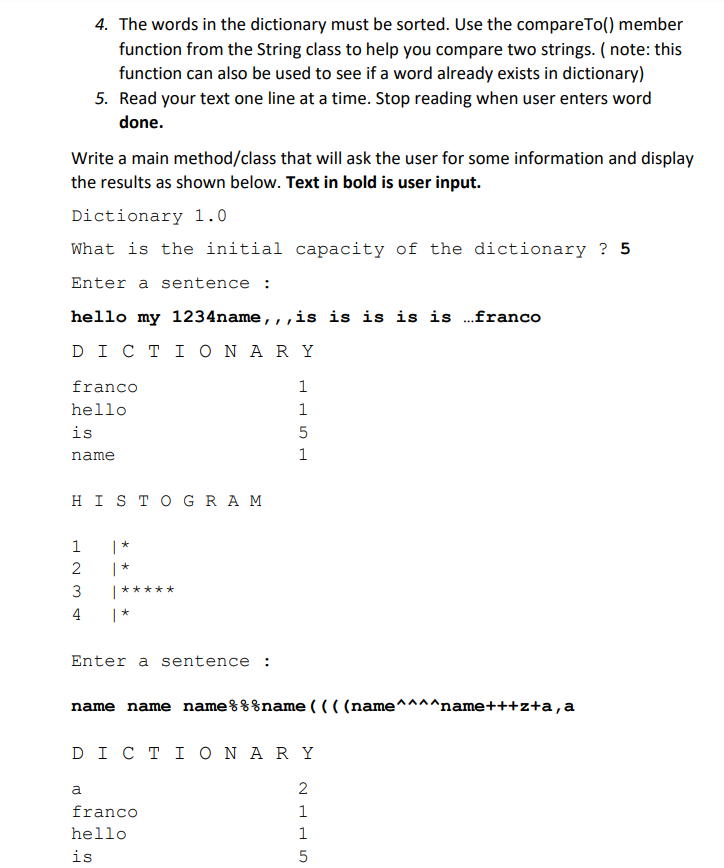
Transcribed Image Text:Write a main method/class that will ask the user for some information and display
the results as shown below. Text in bold is user input.
Dictionary 1.0
What is the initial capacity of the dictionary ? 5
Enter a sentence :
hello my 1234name,,,is is is is is ...franco
DICTIONARY
franco
hello
is
name
HISTOGRAM
1
NT
4. The words in the dictionary must be sorted. Use the compareTo() member
function from the String class to help you compare two strings. (note: this
function can also be used to see if a word already exists in dictionary)
5. Read your text one line at a time. Stop reading when user enters word
done.
34
3
4
|
*
Enter a sentence :
a
name name name%%%name ( ( ( (name^^^^name+++z+a, a
1
1
5
1
DICTIONARY
franco
hello
is
2
ыннN
1
1
5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
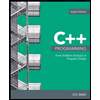
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
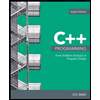
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning