Using C# Language: Programming PLO 3 Measured: Create control structures, methods with the appropriate parameters, and data structures of the appropriate type, and implement algorithms to solve problems. Design a class named Contractor. The class should keep the following information: Contractor name Contractor number Contractor start date Write one or more constructors and the appropriate accessor and mutator functions for the class. Write another class named Subcontractor that is derived from the Contractor class. The Subcontractor class should have member variables to hold the following information: Shift (an integer) Hourly pay rate (a double) The work is divided between two shifts, a day shift, and a night shift. The shift variable will hold an integer value representing the shift that the subcontractor will work. The day shift is 1 and the night shift is 2. Write one or more constructors and the appropriate accessor functions for the class. Demonstrate the classes by writing a program that uses a subcontractor object and allows the user to create as many instances of the subcontractor as needed. Create a method that returns a float and computes pay with a 3% shift differential applied for night shift employees.
Using C# Language:
Design a class named Contractor. The class should keep the following information:
- Contractor name
- Contractor number
- Contractor start date
Write one or more constructors and the appropriate accessor and mutator functions for the class.
Write another class named Subcontractor that is derived from the Contractor class. The Subcontractor class should have member variables to hold the following information:
- Shift (an integer)
- Hourly pay rate (a double)
The work is divided between two shifts, a day shift, and a night shift. The shift variable will hold an integer value representing the shift that the subcontractor will work. The day shift is 1 and the night shift is 2. Write one or more constructors and the appropriate accessor functions for the class. Demonstrate the classes by writing a program that uses a subcontractor object and allows the user to create as many instances of the subcontractor as needed. Create a method that returns a float and computes pay with a 3% shift differential applied for night shift employees.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

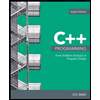
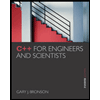
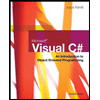
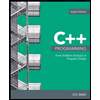
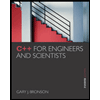
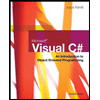