Hello. Please add a toString() function to the Java class implementation of the singly-linked list which returns the contents of the singly-linked list. Also, does Java have a built-in class that implements singly-linked lists? Thank you.
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Hello. Please add a toString() function to the Java class implementation of the singly-linked list which returns the contents of the singly-linked list. Also, does Java have a built-in class that implements singly-linked lists? Thank you.
public class SinglyLinkedList<E>
{
private static class Node<E>
{
private E element;
private Node<E> next;
public Node(E e, Node<E> n)
{
element = e;
next = n;
}
public E getElement() { return element;}
public Node<E> getNext() { return next;}
public void setNext(Node<E> n) { next = n;}
}
private Node<E> head = null;
private Node<E> tail = null;
private int size = 0;
public SinglyLinkedList() {}
public int size() { return size;}
public boolean isEmpty() { return size == 0;}
public E first()
{
if(isEmpty()) return null;
return head.getElement();
}
public E last()
{
if(isEmpty()) return null;
return tail.getElement();
}
public void addFirst(E e)
{
head = new Node<>(e, head);
if(size == 0)
tail = head;
size++;
}
public void addLast(E e)
{
Node<E> newest = new Node<>(e, null);
if(isEmpty())
head = newest;
else
tail.setNext(newest);
tail = newest;
size++;
}
public E removeFirst()
{
if(isEmpty()) return null;
E answer = head.getElement();
head = head.getNext();
size--;
if(size == 0)
tail = null;
return answer;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

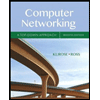
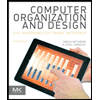
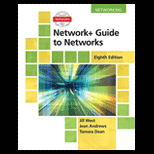
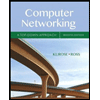
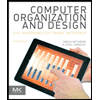
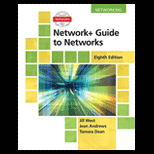
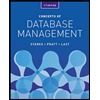
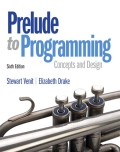
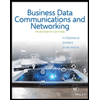