Using JAVA OOP, needs to work on the eclipse IDE The international Olympics Committee has asked you to write a program to process their data and determine the medal winners for the pairs figure skating. You will be given a file named Pairs.txt. This file contains the data for each pair of skaters. The data consists of each skater’s name, their country and the score from each of eight judges on the technical aspects and on the performance aspects. A typical record in the file would be as follows: Smith Jones Australia 5.0 4.9 5.1 5.2 5.0 5.1 5.2 4.8 4.3 4.7 4.8 4.9 4.6 4.8 4.9 4.5 Lennon Murray England 2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.8 3.1 3.2 3.3 3.4 3.5 3.6 3.7 3.8 Gusto Petitot Italia 4.1 4.2 4.3 4.4 4.5 4.6 4.7 4.8 5.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8 Lahaie Petit France 1.1 1.2 1.3 1.4 1.5 1.6 1.7 1.8 5.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8 Bilodeau Bernard Canada 2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.8 4.1 4.2 4.3 4.8 4.9 4.6 4.0 4.5 Lahore Pedro Mexico 3.2 3.1 3.8 3.9 3.0 3.6 3.9 3.3 5.9 5.8 5.8 5.8 5.9 5.6 5.0 5.5 Maliak Kolikov Russia 4.2 4.1 4.8 4.9 4.0 4.6 4.9 4.3 1.9 1.8 1.8 1.8 1.9 1.6 1.0 1.5 Mina Yian China 5.2 5.1 5.8 5.9 5.0 5.6 5.9 5.3 2.9 2.8 2.8 2.8 2.9 2.6 2.0 2.5 Gates Brown U.S 1.2 1.1 1.8 1.9 1.0 1.6 1.9 1.3 3.9 3.8 3.8 3.8 3.9 3.6 3.0 3.5 Joe Charlie Switzerland 2.2 2.1 2.8 2.9 2.0 2.6 2.9 2.3 4.9 4.8 4.8 4.8 4.9 4.6 4.0 4.5 The final score for each skater is the sum of the average of the two categories of the scores. I. Design UML diagram for a class named Skaters to hold the above data and calculate the final score. II. Implement the Skaters class. III. Write a driver class called SkatersDemo to read the data in from pairs.txt and calculates the final score for each pairs of skaters. This class should: • Create an array named skatersArray with elements of type Skaters. • Read the data about each skater from pairs.txt and create an object of type Skaters and store it in the skatersArray. • Sort the elements of the skatersArray in descending order based on the final score. • Display the results on the screen. • Give the special prominence to the medal winners (Gold, Silver, Bronze).
Using JAVA OOP, needs to work on the eclipse IDE
The international Olympics Committee has asked you to write a program to process their data and determine the
medal winners for the pairs figure skating.
You will be given a file named Pairs.txt.
This file contains the data for each pair of skaters. The data consists of each skater’s name, their country and the
score from each of eight judges on the technical aspects and on the performance aspects. A typical record in the
file would be as follows:
Smith
Jones
Australia
5.0 4.9 5.1 5.2 5.0 5.1 5.2 4.8
4.3 4.7 4.8 4.9 4.6 4.8 4.9 4.5
Lennon
Murray
England
2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.8
3.1 3.2 3.3 3.4 3.5 3.6 3.7 3.8
Gusto
Petitot
Italia
4.1 4.2 4.3 4.4 4.5 4.6 4.7 4.8
5.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8
Lahaie
Petit
France
1.1 1.2 1.3 1.4 1.5 1.6 1.7 1.8
5.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8
Bilodeau
Bernard
Canada
2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.8
4.1 4.2 4.3 4.8 4.9 4.6 4.0 4.5
Lahore
Pedro
Mexico
3.2 3.1 3.8 3.9 3.0 3.6 3.9 3.3
5.9 5.8 5.8 5.8 5.9 5.6 5.0 5.5
Maliak
Kolikov
Russia
4.2 4.1 4.8 4.9 4.0 4.6 4.9 4.3
1.9 1.8 1.8 1.8 1.9 1.6 1.0 1.5
Mina
Yian
China
5.2 5.1 5.8 5.9 5.0 5.6 5.9 5.3
2.9 2.8 2.8 2.8 2.9 2.6 2.0 2.5
Gates
Brown
U.S
1.2 1.1 1.8 1.9 1.0 1.6 1.9 1.3
3.9 3.8 3.8 3.8 3.9 3.6 3.0 3.5
Joe
Charlie
Switzerland
2.2 2.1 2.8 2.9 2.0 2.6 2.9 2.3
4.9 4.8 4.8 4.8 4.9 4.6 4.0 4.5
The final score for each skater is the sum of the average of the two categories of the scores.
I. Design UML diagram for a class named Skaters to hold the above data and calculate the final
score.
II. Implement the Skaters class.
III. Write a driver class called SkatersDemo to read the data in from pairs.txt and calculates the final score for each pairs of skaters.
This class should:
• Create an array named skatersArray with elements of type Skaters.
• Read the data about each skater from pairs.txt and create an object of type Skaters and
store it in the skatersArray.
• Sort the elements of the skatersArray in descending order based on the final score.
• Display the results on the screen.
• Give the special prominence to the medal winners (Gold, Silver, Bronze).

Step by step
Solved in 2 steps with 4 images

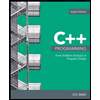
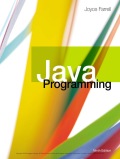
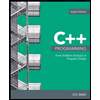
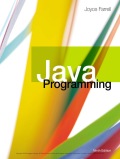