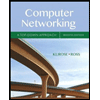
Using Java in Eclipse IDE
/*TASK 5. Implement part 1 of the report. You need to read data from input.txt file, store it into array of items (called items) and write it in a formatted form to the output file. Code, debug and present it in the best possible format.
Hint: It might be helpful to format the String coming from toString( ) method using String.format( ) method, which works similar to System.out.prinf.
Hint2: You might have to close and delete existing output .doc or .txt file from its folder *
public class YourUserName_hw7 {
static Item [ ] items = new Item [200];//an array of Items
static EdibleItem [ ] edibleItems;//an array of Edible Items
static int countEdibleitems = 0;//count edible items
static String pageHeader; //save the header
//main( ) method runs the program
public static void main(String[ ] args) throws FileNotFoundException {
double sum = 0, average = 0;
//Optional - create a new input file
//CreateInput.createInputFile( );
//call the method to read data from input.txt and store in the array of Items
readInputFile( );
//find the total revenue and the average price for this day
//create an output file
java.io.File file = new java.io.File(LocalDate.now( ).toString( )+"_yourusername_hw7_report.doc");
java.io.PrintWriter output = new java.io.PrintWriter(file);//you can rename later
//Part 1: lists all items sold within the December 4, 2021 by the timestamp
output.print("This report prepared on " + LocalDate.now( ) + " by yourusername, CPS*2231*xx\n\n");
//Print the header row of the data
//Print all items into the file (.txt or .doc)
//Display money total and the average price at the end of Part 1
The image is data from the input.txt file
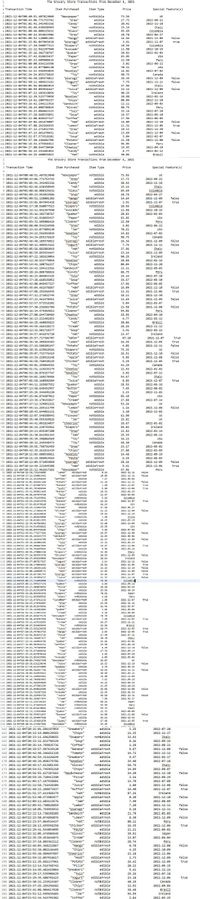

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- The editTemperatures() method takes in two parameters: integer array tempData and integer numMore. Complete editTemperatures() to create a new array called arrayCopy with the same elements as tempData, and the size increased by numMore. Ex: If the input is: 10 48 49 18 3 then the output is: 10 48 49 18 0 0 0 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 Scannerscnr=newScanner(System.in); int[] degreesFahrenheit=newint[4]; intinput; inti; for (i=0; i<degreesFahrenheit.length; ++i) { degreesFahrenheit[i] =scnr.nextInt(); } // Read number of values to increase array size by input=scnr.nextInt(); degreesFahrenheit=editTemperatures(degreesFahrenheit, input); for (i=0; i<degreesFahrenheit.length; ++i) { System.out.print(degreesFahrenheit[i] +" "); } } }arrow_forwardWrite a Java program that accomplishes the same thing as the program in the pictures, except by using two dimensional arrays instead.arrow_forwardThe second picture is the code. I want the output of the code to look like picture one. What part do I have to fix? THX!arrow_forward
- Write a program called Guests.java that prompts a user to enter how many guests he will host. Next, reads a series of the names into an array of Strings using Scanner, and then prints back "Welcome" to all of those guests.arrow_forwardcan you please write in java.util.scanner form and can you make it so i can copy and past it please Create a method public static String[] getFileWords(String filename) This method will 1. open up the file specified as the parameter, 2. read each line one by one, 3. parse each line into String[], 4. merge them into one array (using the mergeArrays() method you just developed), and 5. return the array as result Test this out with the "GettysburgAddress.txt" file in the class' main() method.arrow_forward1. In javascript. Declare an array to hold eight integers. Use a for loop to add eight random integers, all in the range from 50 to 100, inclusive, to this array. Duplicates are okay. Next, pass the array to a method that sorts the array and returns another array containing only the largest and smallest elements in the original array. Print these two values in main. Then use a foreach loop to display all elements of the sorted array on one line separated by a single space. This latter loop should also count the odd and even numbers in the array and determine the sum of all elements in the array.arrow_forward
- Hello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. Implement the following method that returns the maximum element in an array: public static <E extends Comparable<E>> E max(E[] list) Write a test program that generates 10 random integers, invokes this method to find the max, and then displays the random integers sorted smallest to largest and then prints the value returned from the method. Max sure the the last sorted and returned value as the same!arrow_forwardwrite java program for the above questionarrow_forwardCreate an application that reads a sequence of up to 25 names and postal (ZIP) codes for persons. Put the data in an object that can have a first name (string), last name (string), and postal code (integer). Assume that each line of input will have two strings separated by a tab character, followed by an integer value. After the input has been read in, print the list to the screen in the appropriate manner.arrow_forward
- write a program that reads the students.txt file and stores the name of the student and the grade information as a student object in an arraylist sorts the students names based on their first grade in decreasing order using the selection sort algorithm writes the sorted list as the students last name , middle name (if theres one), first name , and grade information into a text file output example: Robison, Lee 95 80 Green, Alex R 85 60 Waston, Zoe G 100 80 use javaarrow_forwardHello! I am having a problem trying to code a addNode method implementation to my program. I am trying to only get it to sort the lines alphabetically and then the categories will be sorted from the way it is first read in the input text file.So an input file would look like this: Name Clovis Bagwell Interesting The quick brown fox jumped over the lazy dog. Interesting Shake rattle and roll. Name Archibald Beechcroft and the output file should look like this: Name Archibald Beechcroft Clovis Bagwell Interesting Shake rattle and roll. The quick brown fox jumped over the lazy dog. My addNode code keeps organizing all of the categories and the lines in alphabetical order when categories is supposed to be organized by the way they are first read in the text file, and the lines under it are alphabetical.arrow_forwardWhen I run this code it says there is an error. Can anyone help point out why I am getting an error for this code? from Artist import *from Artwork import *if __name__ == "__main__":mylist = input().split(" ")user_artist_name = str(mylist[0]+" "+mylist[1])user_birth_year = int(mylist[2])user_death_year = int(mylist[3])#mylist1 = mylist(4:-1)user_title = ""for i in mylist[4:-1]:user_title+=i+" "#user_title = str(mylist[4:-1])user_year_created = int(mylist[-1])user_artist = Artist(user_artist_name,user_birth_year,user_death_year)new_artwork = Artwork(user_title, user_year_created, user_artist)new_artwork.print_info() Here is the error message: Traceback (most recent call last): File "main.py", line 7, in <module> user_birth_year = int(mylist[2]) IndexError: list index out of rangearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
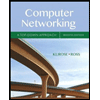
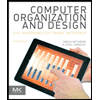
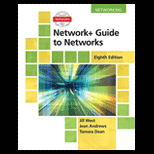
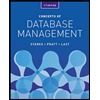
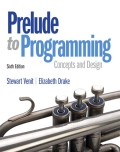
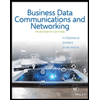