Using Queues to make a Stack Suppose that you have two integer queues but no stack. Implement the basic stack operations (push, pop, and peek) using the queues. (Use the queue class for integers called Queue attached below.) Queue.java is below public class Queue { private int[] queueArray; private int front; private int back; private int size; final int DEFAULT_CAP = 5; /* no-arg constructor initializes a queue with a default capacity of 5, * i.e. the stack can take 5 elements at most, */ public Queue(){ queueArray = new int[DEFAULT_CAP]; front = 0; back = 0; size = 0; } /* constructor that initializes a stack with a specific capacity (given as parameter) * */ public Queue(int cap){ queueArray = new int[cap]; front = 0; back = 0; size = 0; } /* adds a new element to the queue if it is not full * */ public void enqueue (int item){ if (!isFull()){ queueArray[back] = item; back = (back+1) % queueArray.length; size++; } } /* removes the first element from a queue if it is not empty * */ public int dequeue(){ int item=0; if (!isEmpty()){ item = queueArray[front]; front = (front+1) % queueArray.length; size--; } return item; } /* if the queue is not empty, returns the value of the first element from a queue, but does not * remove the element from the queue, */ public int peek(){ int item=0; if (!isEmpty()){ item = queueArray[front]; } return item; } /* returns how many elements are currently in the queue * */ public int getSize(){ return size; } /* returns the capacity of the queue * */ public int getCapacity(){ return queueArray.length; } /* returns true if the queue is full * */ public boolean isFull(){ return (size == queueArray.length); } /* * returns true if the queue is empty */ public boolean isEmpty(){ return (size == 0); } /* * returns a string of all the elements. */ public String toString (){ String str = "This is the queue, front to back: "; int i = front; int count = 0; while (count < size) { str += queueArray[i] + " "; i = (i+1) % queueArray.length; count++; } return str; } /* * compares the queue with another queue given as parameter and returns true if both are of * equal capacity and have the same elements in the same positions, */ public boolean equals(Queue otherQueue){ boolean result = (this.getCapacity() == otherQueue.getCapacity()) && (this.getSize() == otherQueue.getSize()); int i = front; int count = 0; while (count <= size && result){ if (queueArray[i] != otherQueue.queueArray[i]) result = false; i = (i+1) % queueArray.length; count++; } return result; } /* * constructor that copies another queue given as parameter, */ public Queue (Queue otherQueue){ queueArray = new int[otherQueue.queueArray.length]; front = otherQueue.front; back = otherQueue.back; size = otherQueue.size; int i = front; int count = 0; while (count < size) { queueArray[i] = otherQueue.queueArray[i]; i = (i+1) % queueArray.length; count++; } } }
Java
Using Queues to make a Stack
Suppose that you have two integer queues but no stack. Implement the basic stack operations (push, pop, and peek) using the queues. (Use the queue class for integers called Queue attached below.)
Queue.java is below
public class Queue {
private int[] queueArray;
private int front;
private int back;
private int size;
final int DEFAULT_CAP = 5;
/* no-arg constructor initializes a queue with a default capacity of 5,
* i.e. the stack can take 5 elements at most,
*/
public Queue(){
queueArray = new int[DEFAULT_CAP];
front = 0;
back = 0;
size = 0;
}
/* constructor that initializes a stack with a specific capacity (given as parameter)
*
*/
public Queue(int cap){
queueArray = new int[cap];
front = 0;
back = 0;
size = 0;
}
/* adds a new element to the queue if it is not full
*
*/
public void enqueue (int item){
if (!isFull()){
queueArray[back] = item;
back = (back+1) % queueArray.length;
size++;
}
}
/* removes the first element from a queue if it is not empty
*
*/
public int dequeue(){
int item=0;
if (!isEmpty()){
item = queueArray[front];
front = (front+1) % queueArray.length;
size--;
}
return item;
}
/* if the queue is not empty, returns the value of the first element from a queue, but does not
* remove the element from the queue,
*/
public int peek(){
int item=0;
if (!isEmpty()){
item = queueArray[front];
}
return item;
}
/* returns how many elements are currently in the queue
*
*/
public int getSize(){
return size;
}
/* returns the capacity of the queue
*
*/
public int getCapacity(){
return queueArray.length;
}
/* returns true if the queue is full
*
*/
public boolean isFull(){
return (size == queueArray.length);
}
/*
* returns true if the queue is empty
*/
public boolean isEmpty(){
return (size == 0);
}
/*
* returns a string of all the elements.
*/
public String toString (){
String str = "This is the queue, front to back: ";
int i = front;
int count = 0;
while (count < size) {
str += queueArray[i] + " ";
i = (i+1) % queueArray.length;
count++;
}
return str;
}
/*
* compares the queue with another queue given as parameter and returns true if both are of
* equal capacity and have the same elements in the same positions,
*/
public boolean equals(Queue otherQueue){
boolean result = (this.getCapacity() == otherQueue.getCapacity()) && (this.getSize() == otherQueue.getSize());
int i = front;
int count = 0;
while (count <= size && result){
if (queueArray[i] != otherQueue.queueArray[i])
result = false;
i = (i+1) % queueArray.length;
count++;
}
return result;
}
/*
* constructor that copies another queue given as parameter,
*/
public Queue (Queue otherQueue){
queueArray = new int[otherQueue.queueArray.length];
front = otherQueue.front;
back = otherQueue.back;
size = otherQueue.size;
int i = front;
int count = 0;
while (count < size) {
queueArray[i] = otherQueue.queueArray[i];
i = (i+1) % queueArray.length;
count++;
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

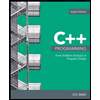
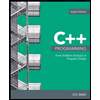