Using the Coin class defined in this chapter, design and imple- ment a driver class called F1ipRace whose main method creates two Coin objects, then continually flips them both to see which coin first comes up heads three flips in a row. Continue flipping the coins until one of the coins wins the race, and consider the possibility that they might tie. Print the results of each turn, and at the end print the winner and total number of flips that were equired.
Using your Eclipse / Java integrated development environment (IDE), code a solution to PP 5.6 from page 250 of your text book. Note that you should use the Coin java file included here without making any changes to it. the Coin java file is here
You should submit the Coin java file (with no changes) and a UML file created using the Violet UML Editor in html format.
//********************************************************************
// Coin.java Authors: Lewis & Loftus
//
// Represents a coin with two sides that can be flipped.
//********************************************************************
public class Coin
{
private final int HEADS = 0;
private final int TAILS = 1;
private int face;
//-----------------------------------------------------------------
// Sets up the coin by flipping it initially.
//-----------------------------------------------------------------
public Coin ()
{
flip();
}
//-----------------------------------------------------------------
// Flips the coin by randomly choosing a face value.
//-----------------------------------------------------------------
public void flip ()
{
face = (int) (Math.random() * 2);
}
//-----------------------------------------------------------------
// Returns true if the current face of the coin is heads.
//-----------------------------------------------------------------
public boolean isHeads ()
{
return (face == HEADS);
}
//-----------------------------------------------------------------
// Returns the current face of the coin as a string.
//-----------------------------------------------------------------
public String toString()
{
String faceName;
if (face == HEADS)
faceName = "Heads";
else
faceName = "Tails";
return faceName;
}
}
and page 250 is attached below
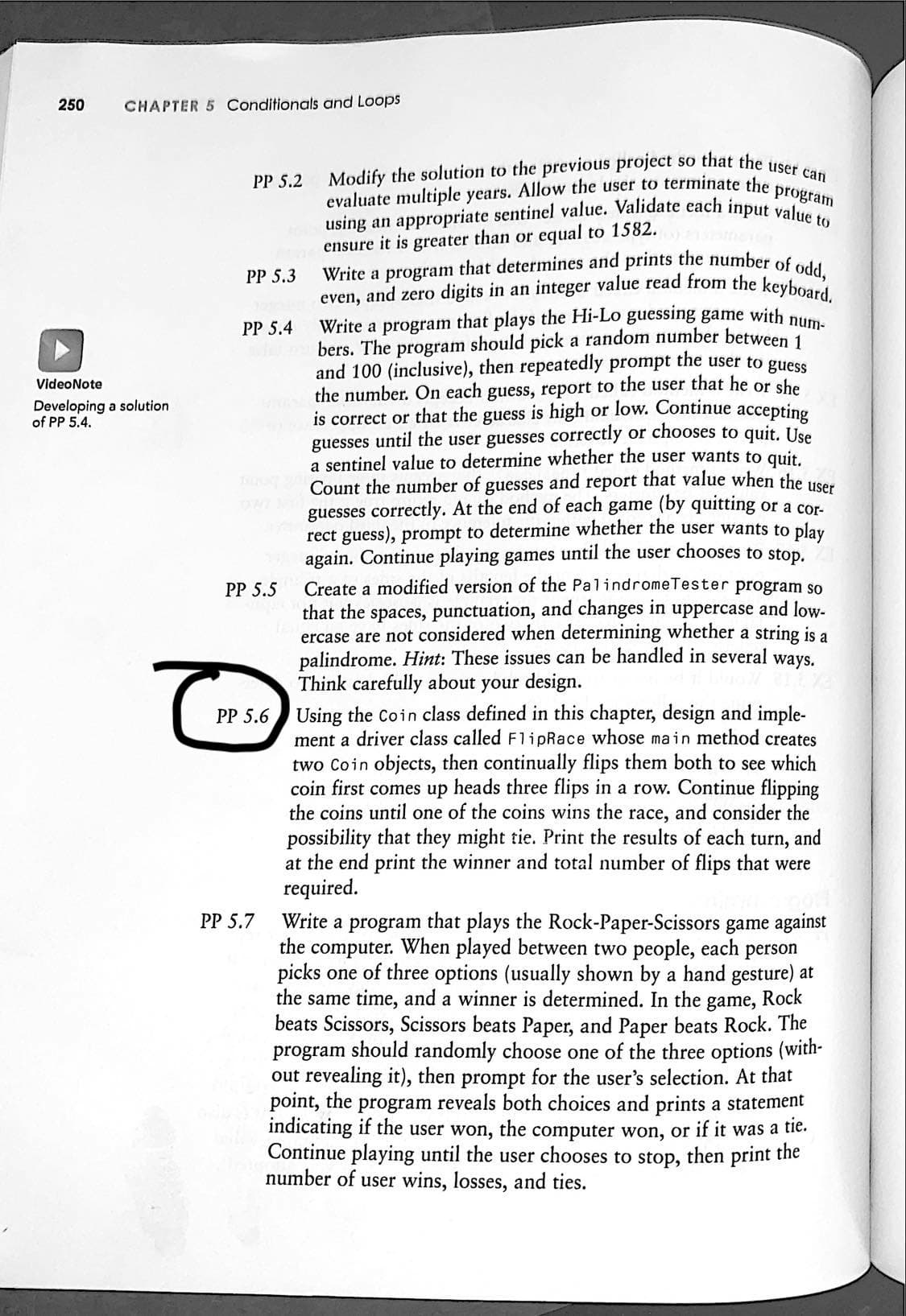

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

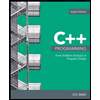
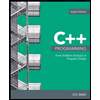