With two arms, a 12-hour clock runs from 00:00 to 11:59. One minute after 11:59, it goes back to 00:00. You will write a class Clock that implements the behaviors of such a clock via the following methods: definit_(self, initial_time: str) sets up the clock to the specified initial_time, which is guaranteed to be given in the format hh:mm (e.g., 11:24, 1:05, 08:07). Importantly, we guarantee that the given initial_time will be between 00:00 and 11:59. def forward(self, minutes: int) advances the time of the clock by minutes minutes. Keep in mind that our clock cannot display any number outside its range. Therefore, adjust the result accordingly. For example, adding 30 minutes to 11:40 will result in 00:10. The minutes parameter can be so large that the clock wraps around several times (e.g., 3800 minutes).
clock = Clock('02:05')
assert (clock.get_hour()==2)
assert (clock.get_minute()==5)
clock.forward(3726)
assert (clock.get_hour()==4)
assert (clock.get_minute()==11)
clock.reset()
assert (clock.get_hour()==0)
assert (clock.get_minute()==0)
clock.forward(3219)
assert (clock.get_hour()==5)
assert (clock.get_minute()==39)
clock.rewind(3551)
assert (clock.get_hour()==6)
assert (clock.get_minute()==28)
clock.rewind(900)
assert (clock.get_hour()==3)
assert (clock.get_minute()==28)
clock.rewind(656)
assert (clock.get_hour()==4)
assert (clock.get_minute()==32)
clock.rewind(2157)
assert (clock.get_hour()==4)
assert (clock.get_minute()==35)
clock.forward(1675)
assert (clock.get_hour()==8)
assert (clock.get_minute()==30)
clock.forward(2534)
assert (clock.get_hour()==2)
assert (clock.get_minute()==44)
clock.rewind(1670)
assert (clock.get_hour()==10)
assert (clock.get_minute()==54)
clock = Clock('11:35')
assert (clock.get_hour()==11)
assert (clock.get_minute()==35)
clock.reset()
assert (clock.get_hour()==0)
assert (clock.get_minute()==0)
clock.forward(2842)
assert (clock.get_hour()==11)
assert (clock.get_minute()==22)
clock.forward(3356)
assert (clock.get_hour()==7)
assert (clock.get_minute()==18)
clock.reset()
assert (clock.get_hour()==0)
assert (clock.get_minute()==0)
clock.forward(3777)
assert (clock.get_hour()==2)
assert (clock.get_minute()==57)
clock.rewind(3072)
assert (clock.get_hour()==11)
assert (clock.get_minute()==45)
clock.forward(1016)
assert (clock.get_hour()==4)
assert (clock.get_minute()==41)
clock.forward(4937)
assert (clock.get_hour()==2)
assert (clock.get_minute()==58)
clock.forward(4637)
assert (clock.get_hour()==8)
assert (clock.get_minute()==15)
clock.forward(1368)
assert (clock.get_hour()==7)
assert (clock.get_minute()==3)
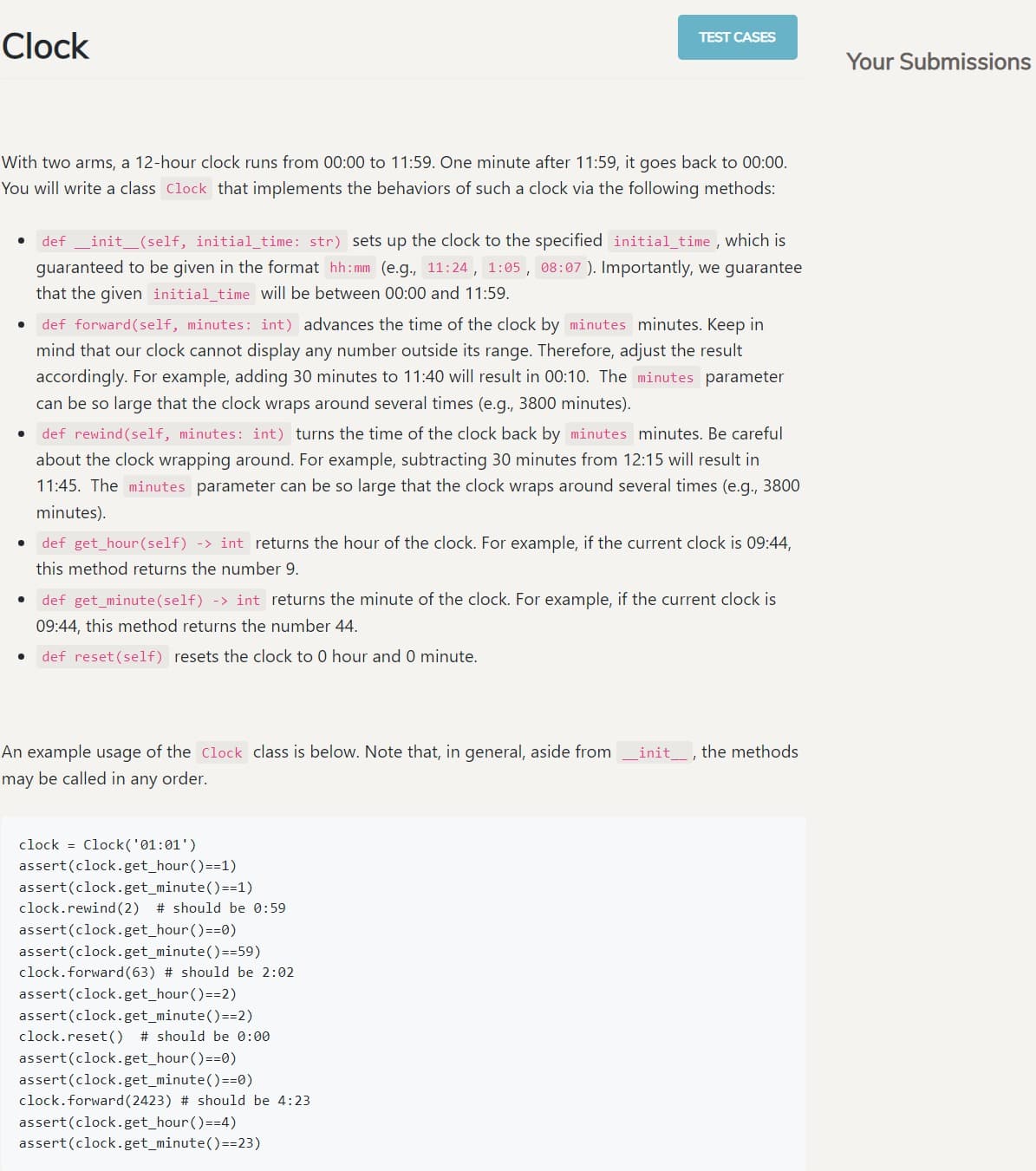

Step by step
Solved in 3 steps with 2 images

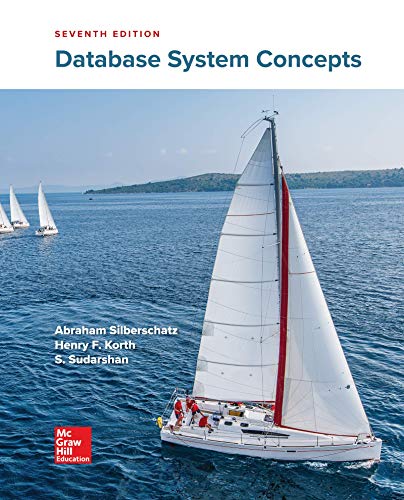
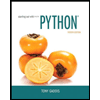
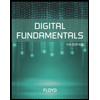
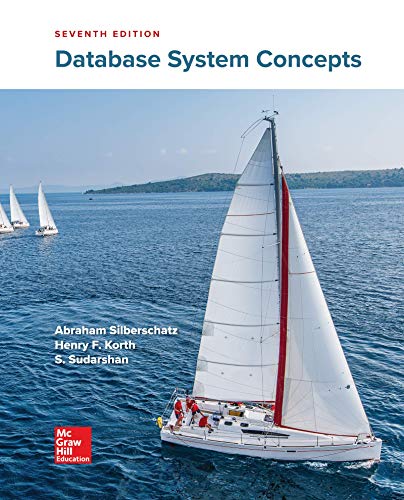
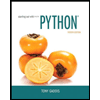
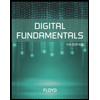
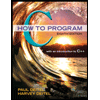
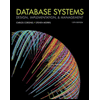
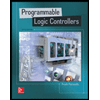