Write a C program to calculate monthly payment and print a table of payment schedule for a fixed rate loan, which performs a similar task as in the link below: http://www.bankrate.com/calculators/mortgages/loan-calculator.aspx In this C program, the input and output are defined as following: Input: amount of loan, interest rate per year and number of payments The attached screenshot below shows a sample of the output. PC yuanlong$ ./loanCalc $500 Output: a table of amortization schedule (also called payment schedule) containin payment number, monthly payment, principal paid, interest paid and new balance at eac row. Yuans-MacBook-Pro: Enter amount of loan Enter Interest rate per year : % 7.5 Enter number of payments : 5 UNP Montly payment should be 101.88 Note: Payment $101.88 $101.88 $101.88 $101.88 $101.88 ===AMORTIZATION SCHEDULE== Principal $98.76 $99.38 $100.00 $100.62 $101.25 Interest $3.12 $2.51 $1.89 $1.26 $0.63 Balance $401.24 $301.87 $201.87 $101.25 $0.00 monthly payments are equal. The way to calculate monthly payment and other values for each row are provided in Appendix. The C program can be implemented within 80 lines of code. If your program is longer than 80 lines, you may need to think about how to simplify your program. Hint: To print out a percentage %, please use %%. You may need to use C math library t calculate the powers of numbers. To compile a C program using math library, you must ad option Im at the end of the cc command to link math library. E.g. gcc -o test test.c -lm
Write a C program to calculate monthly payment and print a table of payment schedule for a fixed rate loan, which performs a similar task as in the link below: http://www.bankrate.com/calculators/mortgages/loan-calculator.aspx In this C program, the input and output are defined as following: Input: amount of loan, interest rate per year and number of payments The attached screenshot below shows a sample of the output. PC yuanlong$ ./loanCalc $500 Output: a table of amortization schedule (also called payment schedule) containin payment number, monthly payment, principal paid, interest paid and new balance at eac row. Yuans-MacBook-Pro: Enter amount of loan Enter Interest rate per year : % 7.5 Enter number of payments : 5 UNP Montly payment should be 101.88 Note: Payment $101.88 $101.88 $101.88 $101.88 $101.88 ===AMORTIZATION SCHEDULE== Principal $98.76 $99.38 $100.00 $100.62 $101.25 Interest $3.12 $2.51 $1.89 $1.26 $0.63 Balance $401.24 $301.87 $201.87 $101.25 $0.00 monthly payments are equal. The way to calculate monthly payment and other values for each row are provided in Appendix. The C program can be implemented within 80 lines of code. If your program is longer than 80 lines, you may need to think about how to simplify your program. Hint: To print out a percentage %, please use %%. You may need to use C math library t calculate the powers of numbers. To compile a C program using math library, you must ad option Im at the end of the cc command to link math library. E.g. gcc -o test test.c -lm
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Implement this C
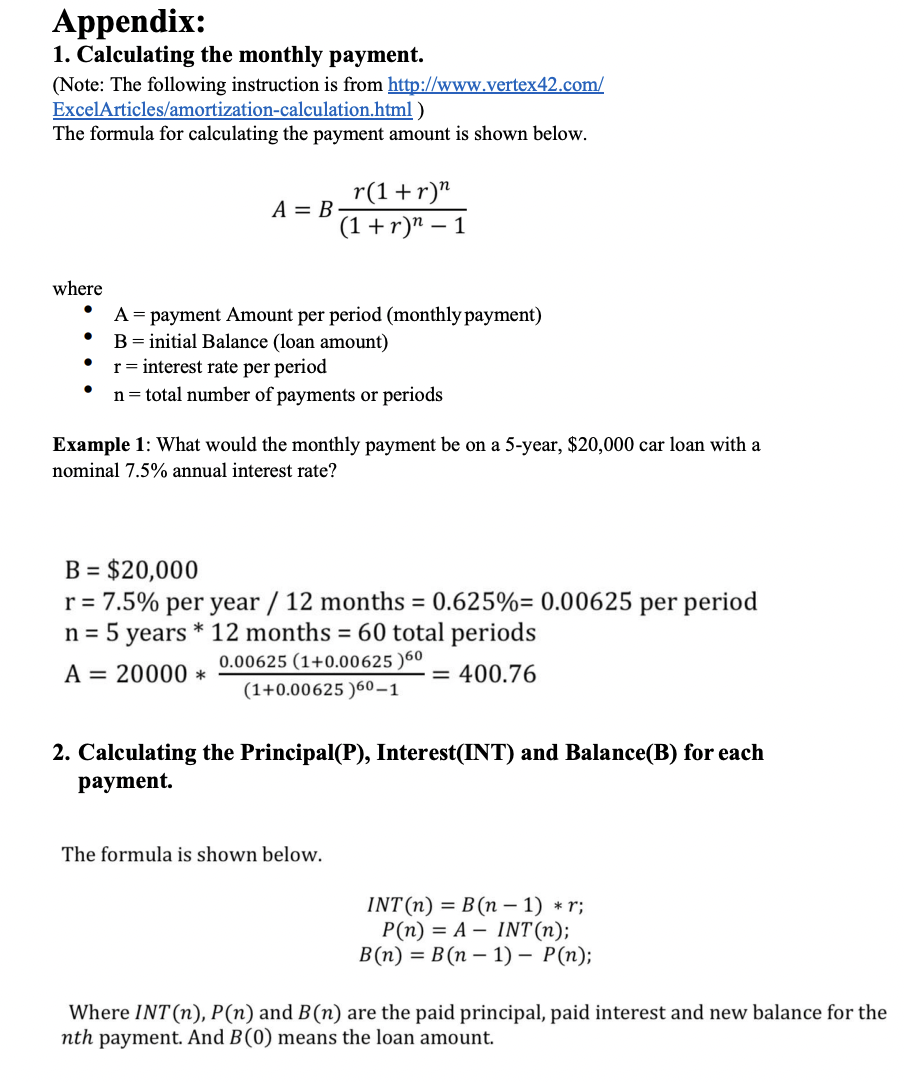
Transcribed Image Text:Appendix:
1. Calculating the monthly payment.
(Note: The following instruction is from http://www.vertex42.com/
ExcelArticles/amortization-calculation.html)
The formula for calculating the payment amount i shown below.
where
r(1+r)^
(1+r)n-1
A = B-
A = payment Amount per period (monthly payment)
B = initial Balance (loan amount)
r = interest rate per period
n = total number of payments or periods
Example 1: What would the monthly payment be on a 5-year, $20,000 car loan with a
nominal 7.5% annual interest rate?
B = $20,000
r = 7.5% per year / 12 months = 0.625%= 0.00625 per period
n = 5 years * 12 months = 60 total periods
0.00625 (1+0.00625)60
A = 20000 *
= 400.76
(1+0.00625 )60-1
2. Calculating the Principal(P), Interest(INT) and Balance(B) for each
payment.
The formula is shown below.
INT(n) = B(n-1) *r;
P(n) = A
INT(n);
B(n)= B(n-1) - P(n);
Where INT (n), P (n) and B(n) are the paid principal, paid interest and new balance for the
nth payment. And B (0) means the loan amount.
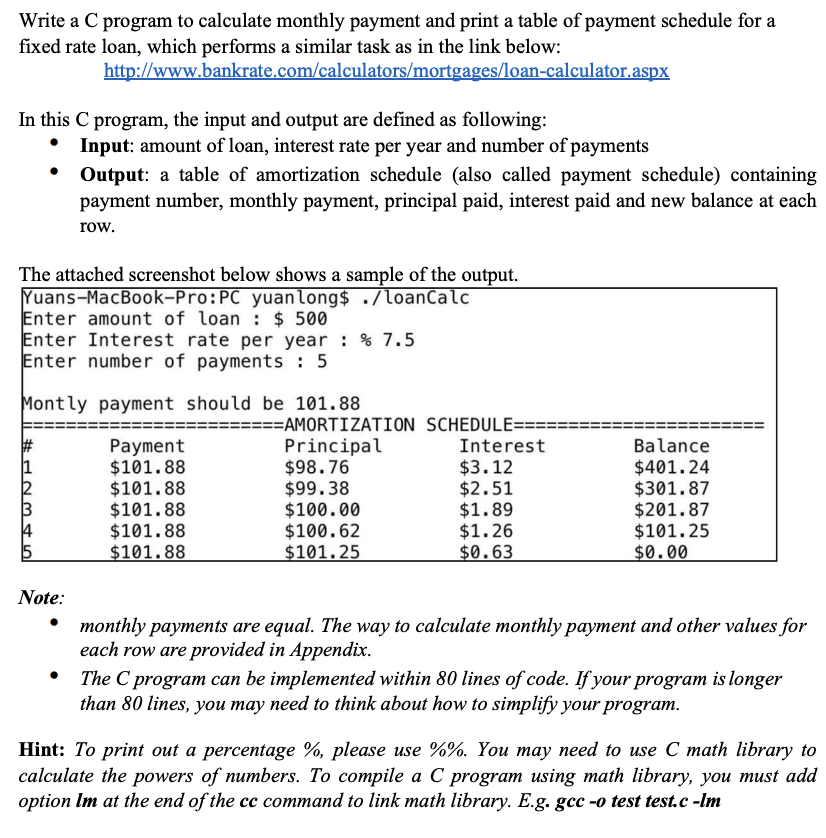
Transcribed Image Text:Write a C program to calculate monthly payment and print a table of payment schedule for a
fixed rate loan, which performs a similar task as in the link below:
http://www.bankrate.com/calculators/mortgages/loan-calculator.aspx
In this C program, the input and output are defined as following:
The attached screenshot below shows a sample of the output.
Yuans-MacBook-Pro:PC yuanlong$ ./loanCalc
Enter amount of loan $500
Enter Interest rate per year : % 7.5
Enter number of payments : 5
UNH
Input: amount of loan, interest rate per year and number of payments
Montly payment should be 101.88
45
Output: a table of amortization schedule (also called payment schedule) containing
payment number, monthly payment, principal paid, interest paid and new balance at each
row.
Note:
Payment
$101.88
$101.88
$101.88
$101.88
$101.88
=====AMORTIZATION SCHEDULE==
Principal
$98.76
$99.38
$100.00
$100.62
$101.25
Interest
$3.12
$2.51
$1.89
$1.26
$0.63
Balance
$401.24
$301.87
$201.87
$101.25
$0.00
monthly payments are equal. The way to calculate monthly payment and other values for
each row are provided in Appendix.
The C program can be implemented within 80 lines of code. If your program is longer
than 80 lines, you may need to think about how to simplify your program.
Hint: To print out a percentage %, please use %%. You may need to use C math library to
calculate the powers of numbers. To compile a C program using math library, you must add
option Im at the end of the cc command to link math library. E.g. gcc -o test test.c -lm
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
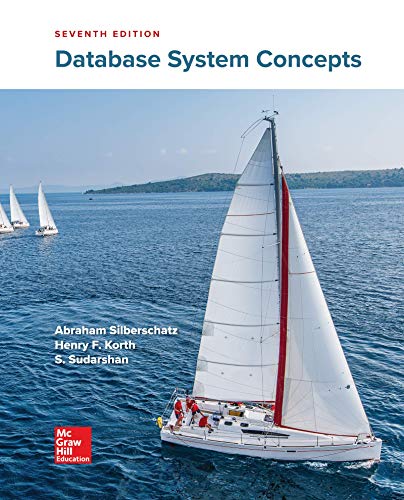
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
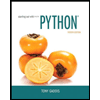
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
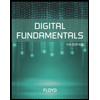
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
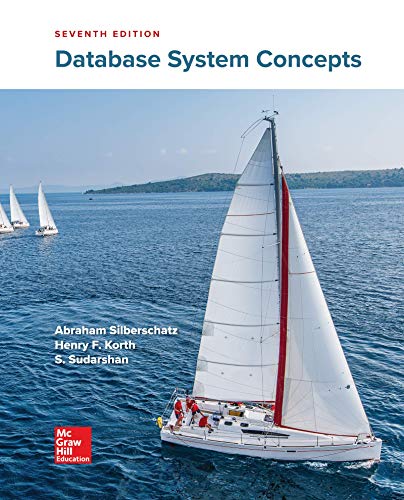
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
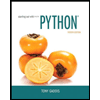
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
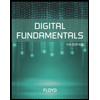
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
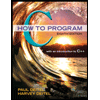
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
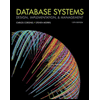
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
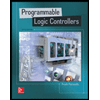
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education