Write a patient billing program that displays a patient's name and bill. Here is sample output: Patient Billing Name: Jenna Parks Bill: $90.00 main follows this sequence: Loop until the user enters -1 for the patient last name. Remember you need to use a method with string comparisons; not the == relational operator. Request from the user: Patient last name Patient first name Claim amount Billing method (1 or 2) Call the adjustName method to format the first and last names. Make two separate calls to this method; one for last name and one for first name. Call the appropriate billing method: 1- Use the deductible (calcBillDeduct) method. 2- Use the medicare (calcBillMed) method. Display the following information: name billing amount The Java class contains these methods. The Chapter Five- Methods notes Area Example includes all the syntax needed for coding methods in the same class as the main method. • displayTitle method displays the title of the report: Patient Billing • adjustName method receives a name, converts the first character to uppercase and the rest to lowercase. The method returns the string. Use the code you wrote in Project One. • calcBillDeduct method receives the claim amount, calculates the patient bill as 80% of the claim after subtracting $50, and returns the billing amount. If the claim is less than or equal to $50, return a $10 fee for processing for the billing amount. • calcBilIMed method receives the claim amount, calculates the patient bill as 35% of the claim and returns the billing amount.
//********************************************************************
//EvenOdd.java Java Foundations
//
//Demonstrates the use of the JOptionPane class.
//********************************************************************
import javax.swing.JOptionPane;
public class EvenOdd
{
//-----------------------------------------------------------------
// Determines if the value input by the user is even or odd.
// Uses multiple dialog boxes for user interaction.
//-----------------------------------------------------------------
public static void main (String[] args)
{
String numStr, result;
int num, again;
do
{
numStr = JOptionPane.showInputDialog ("Enter an integer: ");
num = Integer.parseInt(numStr);
result = "That number is " + ((num%2 == 0) ? "even" : "odd");
JOptionPane.showMessageDialog (null, result);
again = JOptionPane.showConfirmDialog (null, "Do Another?");
}
while (again == JOptionPane.YES_OPTION);
System.out.println("here");
}
}
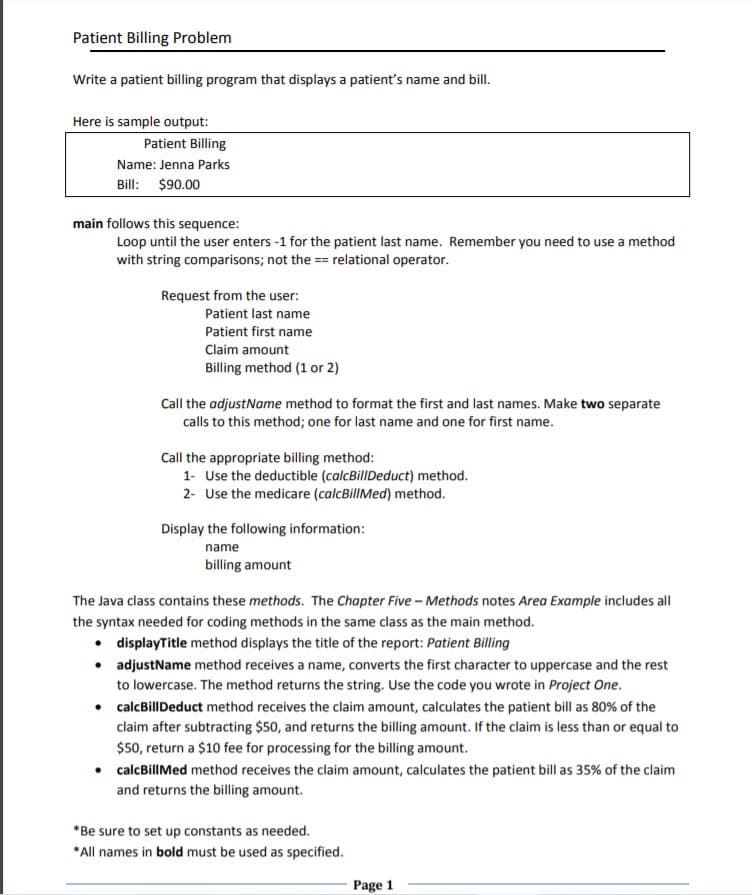
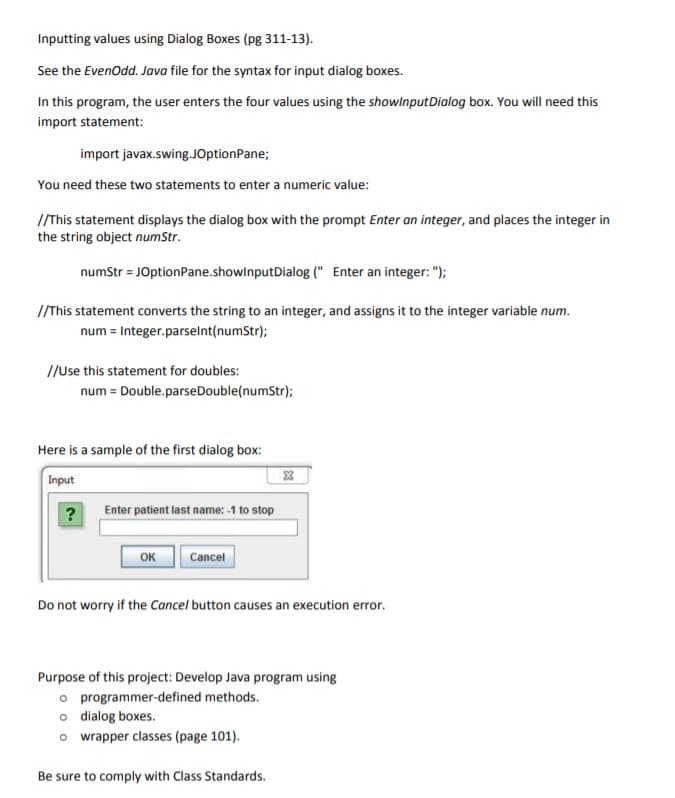

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

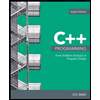
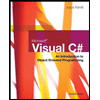
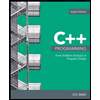
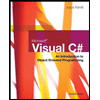