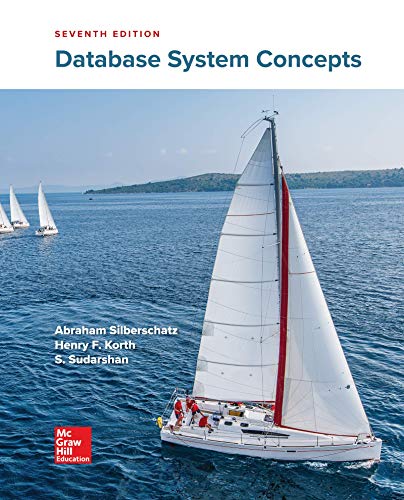
Create a JavaScript function expression that meets the following requirements:
_breakArray(array, n)
• Authored using function expression syntax (constant name _breakAway)
• Accepts an array of integers as the first argument, and a single integer as the second argument (n).
• The function divides the array into chunks of size n, where n is the length of each created (divided) array
• It is possible that the last array created is not completely filled (see example 2 below)
• The integers provided in the array argument, and for the value of n, are always single-digit integers.
o validation is not required for this single-digit assumption.
• Console log output is NOT permitted.
• The function returns an array containing the array groupings back to the caller.
_breakAway([2,3,4,5], 2) → [ [2,3],[4,5] ]
_breakAway([2,3,4,5,6], 2) → [ [2,3],[4,5],[6] ]
_breakAway([2,3,4,5,6,7], 3) → [ [2,3,4],[5,6,7] ]
_breakAway([2,3,4,5], 1) → [ [2],[3],[4],[5],[6],[7] ]
_breakAway([2,3,4,5,6,7], 7) → [ [2,3,4,5,6,7] ]

Step by stepSolved in 3 steps with 2 images

- Help now please Pythonarrow_forwardwrite this using java script arrow functions -thank you :)arrow_forwardIn C programming: Write a main() function using the following requirements:• Define a SIZE constant (the value is irrelevant, but for testing, you may want to keep it small enough – no bigger than 5)• Create an array of course pointers using SIZE• Dynamically allocate each element of the array• Call inputAllCourses()• Call printAllCourses().arrow_forward
- Java code Screenshot and output is mustarrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forward1. Display Function Implement a function to display the contents of the patient_list array. Add code to call this function. Note that there are multiple places where this function needs to be called. Look for the // TODO comments to find the correct locations. 2. Sorting the array by Age Implement the code to sort the contents of the patient_list array based on the value stored in the age field. To do this you will need to implement code that relies on the qsort function from the C Standard library A function that compares two patient elements, based on the value stored in the age field. A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements. Add code to call the qsort function, using the age comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main(). Sorting the array by…arrow_forward
- C++arrow_forwardMIPS Assembly The program: Write a function in MIPS assembly that takes an array of integers and finds local minimum points. i.e., points that if the input entry is smaller than both adjacent entries. The output is an array of the same size of the input array. The output point is 1 if the corresponding input entry is a relative minimum, otherwise 0. (You should ignore the output array's boundary items, set to 0.) My code: # (Note: The first/last entry of the output array is always 0# since it's ignored, never be a local minimum.)# $a0: The base address of the input array# $a1: The base address of the output array with local minimum points# $a2: Size of arrayfind_local_minima:############################ Part 2: your code begins here ###la $t1, ($t2)la $t1, ($t2)move $a1, $s0 li $a2, 4jal find_local_minima print:ble $a2, 0, exitlw $a0, ($s0)li $v0, 1syscall addi $s0, $s0, 4addi $a2, $a2, -1 ############################ Part 2: your code ends here ###jr $ra I am not getting the correct…arrow_forwardPlease complete the following guidelines and hints. Using C language. Please use this template: #include <stdio.h>#define MAX 100struct cg { // structure to hold x and y coordinates and massfloat x, y, mass;}masses[MAX];int readin(void){/* Write this function to read in the datainto the array massesnote that this function should return the number ofmasses read in from the file */}void computecg(int n_masses){/* Write this function to compute the C of Gand print the result */}int main(void){int number;if((number = readin()) > 0)computecg(number);return 0;}Testing your workTypical Input from keyboard:40 0 10 1 11 0 11 1 1Typical Output to screen:CoG coordinates are: x = 0.50 y = 0.50arrow_forward
- In cpparrow_forwardCreate the following functions makeBoard Accepts two parameters, integers mm and nn, and creates an mm x nn numpy array of zeros Returns the created array makeMove Accepts two parameters, a 2D array (such as created by makeBoard), and an integer xx Checks if the value at position xx in the array is 0. If it is, changes it to 1 and returns True If it isn't, does not change it and returns False Position xx in the array is given by reading the array top-down and left-to right. A simple 2x3 array is seen below with each value representing its position You may assume without checking that the value of xx provided is valid, that is it is within the array. Hint: Arrays have a property you can use to determine their shape. Hint: This function may (and indeed should) contain if statements, but no loops. Sample array with positions: [[0 1 2][ 3 4 5]] For testing purposes, you may copy the function provided here into your code def playGame(mm,nn): board = makeBoard(mm,nn)…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
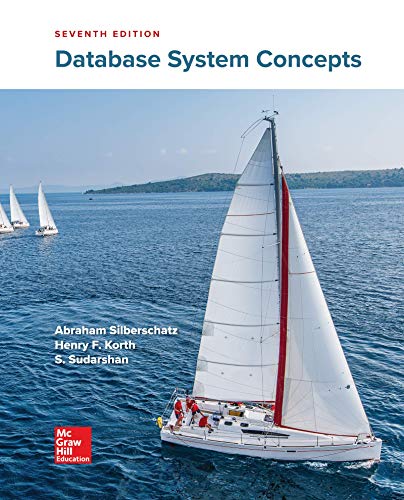
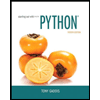
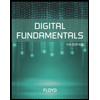
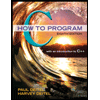
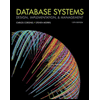
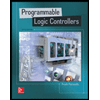