Write a program that reads a list of words. The program should output those words and their frequencies. The input begins with an integer indicating the number of words that follow. For example, If the input is: 5 hey hi Mark hi mark the output of main is: Word: hey Frequency: 1 Word: hi Frequency: 2 Word: Mark Frequency: 1 Word: mark Frequency: 1
Write a program that reads a list of words. The program should output those words and their frequencies. The input begins with an integer indicating the number of words that follow. For example, If the input is: 5 hey hi Mark hi mark the output of main is: Word: hey Frequency: 1 Word: hi Frequency: 2 Word: Mark Frequency: 1 Word: mark Frequency: 1
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Write a program that reads a list of words. The program should output those words and their frequencies. The input begins with an integer indicating the number of words that follow.
For example, If the input is:
5
hey
hi
Mark
hi
mark
the output of main is:
Word: hey Frequency: 1
Word: hi Frequency: 2
Word: Mark Frequency: 1
Word: mark Frequency: 1
![35
/*
о 32 А 12
36
Go through every element in String[] line and find all
37
words
38
39
input:
40
String[] line: Array of words
41
output:
42
ArrayList<String>: An Arraylist containing all the unique words in line
43
44
hint: Arraylists have a method called contains
45
*/
public static ArrayList<String> get_unique_words(String[] line)
46
47
{
48
<YOUR CODE HERE>
49
50
51
public static void main(String[] args)
52
{
53
int numwords;
// Implement a Scanner (call it input)
<YOUR CODE HERE>
54
55
56
// Get an int from the Scanner and save it to numwords
57
<YOUR CODE HERE>
58
59
String[] line = get_words (input, numwords);
60
ArrayList<String> unique_words = get_unique_words(line);
61
for (int i=0;i< unique_words.size();i++)
62
{
63
int frequency =
get_word_frequencies(unique_words.get(i),line);
System.out.println("Word:
+ unique_words.get(i) + "\tFrequency:
+ frequency);
64
65
66
67
68](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd173c705-5d93-4c6d-8e13-5d1915d3c529%2Ff638266d-5874-43b1-b80b-935a67d8e43d%2Fy02fkrw_processed.png&w=3840&q=75)
Transcribed Image Text:35
/*
о 32 А 12
36
Go through every element in String[] line and find all
37
words
38
39
input:
40
String[] line: Array of words
41
output:
42
ArrayList<String>: An Arraylist containing all the unique words in line
43
44
hint: Arraylists have a method called contains
45
*/
public static ArrayList<String> get_unique_words(String[] line)
46
47
{
48
<YOUR CODE HERE>
49
50
51
public static void main(String[] args)
52
{
53
int numwords;
// Implement a Scanner (call it input)
<YOUR CODE HERE>
54
55
56
// Get an int from the Scanner and save it to numwords
57
<YOUR CODE HERE>
58
59
String[] line = get_words (input, numwords);
60
ArrayList<String> unique_words = get_unique_words(line);
61
for (int i=0;i< unique_words.size();i++)
62
{
63
int frequency =
get_word_frequencies(unique_words.get(i),line);
System.out.println("Word:
+ unique_words.get(i) + "\tFrequency:
+ frequency);
64
65
66
67
68
![1
Gimport java.util.ArrayList;
9 32
2
Aimport java.util.Scanner;
4
5 >
public class FrequencyList
6.
{
/*
8.
Given a single String word, traverse the String[] line
9
to find the number of times word is given in line
10
11
input:
12
String word: Word we are looking for
13
String[] line: Array of words
14
output:
15
int: frequency of word in the array line
16
*/
public static int get_word_frequencies(String word, String[] line)
17
18
{
19
<YOUR CODE HERE>
20
}
21
22
23
Create a String[] of size numwords that is populated with words a scanner
24
25
input:
26
int numwords: the number of words to be expected as input
27
output:
28
String[]: Array of words that is recieved from user via Scanner
29
30
*/
31
public static String[] get_words(Scanner input, int numwords){
32
<YOUR CODE HERE>
33
34](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd173c705-5d93-4c6d-8e13-5d1915d3c529%2Ff638266d-5874-43b1-b80b-935a67d8e43d%2Fh56iy0q_processed.png&w=3840&q=75)
Transcribed Image Text:1
Gimport java.util.ArrayList;
9 32
2
Aimport java.util.Scanner;
4
5 >
public class FrequencyList
6.
{
/*
8.
Given a single String word, traverse the String[] line
9
to find the number of times word is given in line
10
11
input:
12
String word: Word we are looking for
13
String[] line: Array of words
14
output:
15
int: frequency of word in the array line
16
*/
public static int get_word_frequencies(String word, String[] line)
17
18
{
19
<YOUR CODE HERE>
20
}
21
22
23
Create a String[] of size numwords that is populated with words a scanner
24
25
input:
26
int numwords: the number of words to be expected as input
27
output:
28
String[]: Array of words that is recieved from user via Scanner
29
30
*/
31
public static String[] get_words(Scanner input, int numwords){
32
<YOUR CODE HERE>
33
34
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
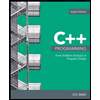
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
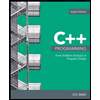
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning