Write a program to help a university system to store records for its employee. You have to perform the following tasks: 1. Define a struct facultyMemberwith the following attributes: • ID number (int) • First Name (string) • Last Name (string) • Designation (string) e.g. Assistant professor, Lecturer etc. 2. Implement a function “void newrecord(facultyMember & fm)",which will take an argument of Faculty:Member type, input values for all the attributes from user, and store it in the argument variable. 3. Implement a function “void printdetails(facultyMember fm)", whichwill print the values of the variable fm passed as an argument. 4. Implement your main function. Declare a variable of FacultyMember type. Assign values to it using NewRecord function. Print its values using PrintDetails function. 5. Now, declare a FacultyMember type array of size 3 in main( ). Fill the values using newRecord function. (Note that your newRecord can assign value to a single FacultyMember type variable and you cannot change the prototype). 6. Print the values of the above array using PrintDetails Function without changing the prototype. 7. Implement a function “void sortid(facultyMember fm [], int size)", which takes a FacultyMember type array as an argument and its maximum size. You have to sort this array in ascending order with respeet to the ID number (use any sorting algorithms). Be careful while swapping the two locations of array.
Write a program to help a university system to store records for its employee. You have to perform the following tasks: 1. Define a struct facultyMemberwith the following attributes: • ID number (int) • First Name (string) • Last Name (string) • Designation (string) e.g. Assistant professor, Lecturer etc. 2. Implement a function “void newrecord(facultyMember & fm)",which will take an argument of Faculty:Member type, input values for all the attributes from user, and store it in the argument variable. 3. Implement a function “void printdetails(facultyMember fm)", whichwill print the values of the variable fm passed as an argument. 4. Implement your main function. Declare a variable of FacultyMember type. Assign values to it using NewRecord function. Print its values using PrintDetails function. 5. Now, declare a FacultyMember type array of size 3 in main( ). Fill the values using newRecord function. (Note that your newRecord can assign value to a single FacultyMember type variable and you cannot change the prototype). 6. Print the values of the above array using PrintDetails Function without changing the prototype. 7. Implement a function “void sortid(facultyMember fm [], int size)", which takes a FacultyMember type array as an argument and its maximum size. You have to sort this array in ascending order with respeet to the ID number (use any sorting algorithms). Be careful while swapping the two locations of array.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section12.2: Providing Class Conversion Capabilities
Problem 2E
Related questions
Question
100%
write a c++ program
![Write a program to help a university system to store records for its employee. You have to
perform the following tasks:
1. Define a struct facultyMemberwith the following attributes:
• ID number (int)
• First Name (string)
• Last Name (string)
• Designation (string) e.g. Assistant professor, Lecturer etc.
2. Implement a function “void newrecord(facultyMember & fm)",which will take an
argument of FacultyMember type, input values for all the attributes from user, and store it in
the argument variable.
3. Implement a function "void printdetails(facultyMember fm)", whichwill print the values
of the variable fm passed as an argument.
4. Implement your main function. Declare a variable of FacultyMember type. Assign values to
it using NewRecord function. Print its values using PrintDetails function.
5. Now, declare a FacultyMember type array of size 3 in main( ). Fill the values using
newRecord function. (Note that your newRecord can assign value to a single FacultyMember
type variable and you cannot change the prototype).
6. Print the values of the above array using PrintDetails Function without changing the
prototype.
7. Implement a function "void sortid(facultyMember fm [], int size)", which takes a
FacultyMember type array as an argument and its maximum size. You have to sort this array
in ascending order with respect to the ID number (use any sorting algorithms). Be careful
while swapping the two locations of array.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9b96bb27-33a3-41d9-b420-8de1a1cde6cd%2Fbe06cd56-5bbc-4436-8639-58a4983af79f%2Fg0cyqbh_processed.png&w=3840&q=75)
Transcribed Image Text:Write a program to help a university system to store records for its employee. You have to
perform the following tasks:
1. Define a struct facultyMemberwith the following attributes:
• ID number (int)
• First Name (string)
• Last Name (string)
• Designation (string) e.g. Assistant professor, Lecturer etc.
2. Implement a function “void newrecord(facultyMember & fm)",which will take an
argument of FacultyMember type, input values for all the attributes from user, and store it in
the argument variable.
3. Implement a function "void printdetails(facultyMember fm)", whichwill print the values
of the variable fm passed as an argument.
4. Implement your main function. Declare a variable of FacultyMember type. Assign values to
it using NewRecord function. Print its values using PrintDetails function.
5. Now, declare a FacultyMember type array of size 3 in main( ). Fill the values using
newRecord function. (Note that your newRecord can assign value to a single FacultyMember
type variable and you cannot change the prototype).
6. Print the values of the above array using PrintDetails Function without changing the
prototype.
7. Implement a function "void sortid(facultyMember fm [], int size)", which takes a
FacultyMember type array as an argument and its maximum size. You have to sort this array
in ascending order with respect to the ID number (use any sorting algorithms). Be careful
while swapping the two locations of array.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
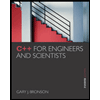
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
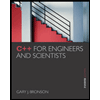
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr