Write a recursive function: def how_deep(list_struct): You will be passed a list. However this is not a generic list, it will conform to these specific rules. A list will either be: Empty A list of lists. This means for example that a list can either be: [], or [[],[],[]], or [[[],[],[],[[[]]]], []] Our goal is to calculate the depth of these lists. It must be done recursively. The depth of [] is 1 since it's a single list. The depth of [[],[]] is 2 since there are lists within lists, but both of those lists are at the same depth. The depth of [[[]],[],[[]],[[[]]]] is 4 because the first sublist is depth 2, then 1, then 2 again, and then 3. Therefore 1 + 3 = 4. The depth of any list is considered to be the max of the depths of the sublists + 1. (This is the definition you should use for the purposes of the recursion).
I really need help with this python question. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable.
Write a recursive function:
def how_deep(list_struct):
You will be passed a list. However this is not a generic list, it will conform to these specific rules.
A list will either be:
- Empty
- A list of lists.
This means for example that a list can either be:
[], or [[],[],[]], or [[[],[],[],[[[]]]], []]
Our goal is to calculate the depth of these lists. It must be done recursively.
The depth of [] is 1 since it's a single list.
The depth of [[],[]] is 2 since there are lists within lists, but both of those lists are at the same depth.
The depth of [[[]],[],[[]],[[[]]]] is 4 because the first sublist is depth 2, then 1, then 2 again, and then 3. Therefore 1 + 3 = 4.
The depth of any list is considered to be the max of the depths of the sublists + 1. (This is the definition you should use for the purposes of the recursion).
Hint: You'll need a for loop in your recursive case.
For this problem, you may add helper functions if you want to, and/or add ONLY DEFAULT parameters to the recursive function (again, if you want). They must work with the given driver code exactly.
Test Driver and Starter Code
Here I've included a little function which produces samples to test out with the max_depth. It won't always produce something with that depth, but it will produce something with depth <= max_depth. Feel free to use this code to make new test cases and to test them out on your function.
The test driver code is:
import random
def make_list_structure(max_depth, p=.8):
if max_depth and random.random() < p:
new_list = []
for i in range(5):
sub_list = make_list_structure(max_depth - 1, p * .9)
if sub_list is not None:
new_list.append(sub_list)
return new_list
return None
if __name__ == '__main__':
print(how_deep([[[], [], [], [[[]]]], []]))
print(how_deep([]))
print(how_deep([[], []]))
print(how_deep([[[]], [], [[]], [[[]]]]))
print(how_deep([[[[], [[]], [[[]]], [[[[]]]]]]]))
print(how_deep([[[], []], [], [[], []]]))
Sample Output
linux5[109]% python3 how_deep.py 5 1 2 4 7 3 |
Coding Standards
- Constants above your function definitions, outside of the "if __name__ == '__main__':" block.
- A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance:
- print(first_creature_name, 'has died in the fight. ') does not involve magic values.
- However, if my_string == 'EXIT': exit is a magic value since it's being used to compare against variables within your code, so it should be:
EXIT_STRING = 'EXIT'
- A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance:
...
if my_string == EXIT_STRING:
- A number is a magic value when it is not 0, 1, and if it is not 2 being used to test parity (even/odd).
- A number is magic if it is a position in an array, like my_array[23], where we know that at the 23rd position, there is some special data. Instead it should be
USERNAME_INDEX = 23
my_array[USERNAME_INDEX]
- Constants in mathematical formulas can either be made into official constants or kept in a formula.
- Previously checked coding standards involving:
- snake_case_variable_names
- CAPITAL_SNAKE_CASE_CONSTANT_NAMES
- Use of whitespace (2 before and after a function, 1 for readability.)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

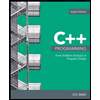
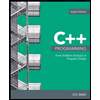