Write C++ program that will read student records from a data file and sort the contents. Declare a class to describe a student record with private fields for: first name last name student id declared major grade point average date of birth Use appropriate data types, constructor(s), and accessor methods. Do not hard-code values. The comma-separated value (CSV) text data file students.csv is provided for development and testing. Other data files may also be used. These will have the same fields with an arbitrary number of records. After the file is read, and the data stored in memory, write a message to the console indicating how many records were read. xx records read. Prompt the user to select the sort criterion field Sort by: (L)ast name, (S)tudent Id, (M)ajor, (G)PA, (A)ge, or (Quit) Read the single-character user selection. Sort the data records using a selection sort method. Print the sorted data records to the console using a toString() method from the class that you created. After the last records are printed, repeat the user menu selections and loop until the user selects 'Q' to quit the application. Do not use any global variables.
Write C++ program that will read student records from a data file and sort the contents.
Declare a class to describe a student record with private fields for:
- first name
- last name
- student id
- declared major
- grade point average
- date of birth
Use appropriate data types, constructor(s), and accessor methods.
Do not hard-code values. The comma-separated value (CSV) text data file students.csv is provided for development and testing. Other data files may also be used. These will have the same fields with an arbitrary number of records.
After the file is read, and the data stored in memory, write a message to the console indicating how many records were read.
- xx records read.
Prompt the user to select the sort criterion field
- Sort by: (L)ast name, (S)tudent Id, (M)ajor, (G)PA, (A)ge, or (Quit)
Read the single-character user selection.
Sort the data records using a selection sort method. Print the sorted data records to the console using a toString() method from the class that you created.
After the last records are printed, repeat the user menu selections and loop until the user selects 'Q' to quit the application.
Do not use any global variables.
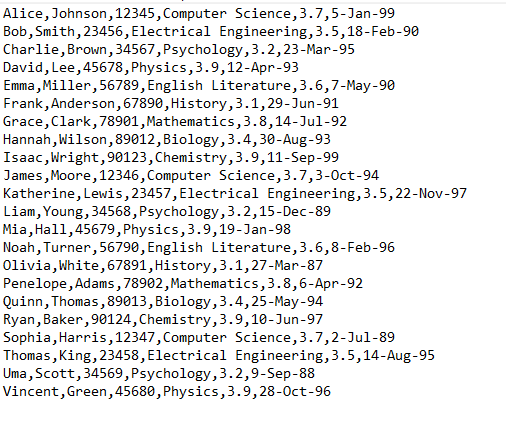

Step by step
Solved in 3 steps with 2 images

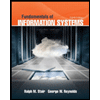
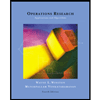
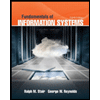
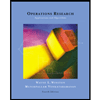